


Master dependency injection in Go language and improve code flexibility
依赖注入是一种设计模式,它允许在运行时动态设置对象依赖项,提高代码灵活性。Go 语言中可以使用 context 包实现依赖注入,只需通过 context.WithValue 添加值,并通过 context.Value 检索即可。例如,可以使用依赖注入来模拟数据库,通过 context 注入 MockDB 实现,可轻松切换依赖项,提升代码可测试性和可维护性。
掌握 Go 语言中的依赖注入,提升代码灵活性
什么是依赖注入?
依赖注入是一种设计模式,它允许我们在运行时动态设置对象的依赖项,而不是在编译时硬编码它们。这提供了更大的灵活性,并使我们能够更轻松地测试和维护代码。
Go 语言中的依赖注入
Go 语言有几种实现依赖注入的方法。最流行的方法之一是使用 context
包。我们可以通过 context.WithValue
函数向 context 添加值,然后通过 context.Value
函数检索值。
案例:使用依赖注入来模拟数据库
让我们考虑一个使用模拟数据库的示例。我们首先定义一个 DB
接口,该接口声明了我们数据库的所需方法:
type DB interface { Get(key string) (string, error) Set(key string, value string) error }
然后,我们将创建一个 MockDB
(模拟数据库)的实现:
type MockDB struct { m map[string]string } func (m *MockDB) Get(key string) (string, error) { return m.m[key], nil } func (m *MockDB) Set(key string, value string) error { m.m[key] = value return nil }
现在,我们可以使用依赖注入来将 MockDB
注入到我们的服务中。首先,创建一个 context
对象并将其注入到我们的服务中:
ctx := context.Background() ctx = context.WithValue(ctx, "db", &MockDB{m: make(map[string]string)})
然后,我们可以从上下文中检索 DB
对象:
db := ctx.Value("db").(DB)
现在,我们可以像使用普通数据库一样使用我们的 MockDB
:
value, err := db.Get("foo") if err != nil { // 处理错误 }
优点
使用依赖注入有很多优点,包括:
- 灵活性:我们可以轻松地在运行时切换依赖项,而无需更改代码。
- 可测试性:我们可以通过注入模拟依赖项来更轻松地测试我们的代码。
- 可维护性:我们不必在编译时硬编码依赖项,从而使代码更易于维护。
结论
依赖注入是提高 Go 代码灵活性和可测试性的有用模式。通过使用 context
包或其他依赖注入库,我们可以轻松地注入依赖项并提升代码的质量。
The above is the detailed content of Master dependency injection in Go language and improve code flexibility. For more information, please follow other related articles on the PHP Chinese website!
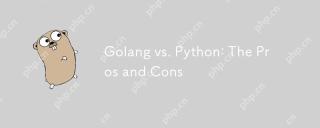
Golangisidealforbuildingscalablesystemsduetoitsefficiencyandconcurrency,whilePythonexcelsinquickscriptinganddataanalysisduetoitssimplicityandvastecosystem.Golang'sdesignencouragesclean,readablecodeanditsgoroutinesenableefficientconcurrentoperations,t
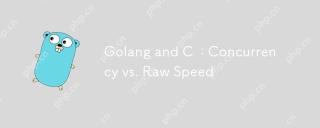
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
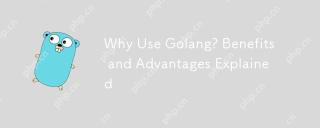
Reasons for choosing Golang include: 1) high concurrency performance, 2) static type system, 3) garbage collection mechanism, 4) rich standard libraries and ecosystems, which make it an ideal choice for developing efficient and reliable software.
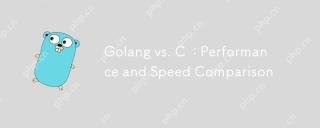
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
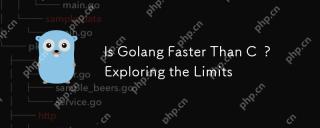
Golang performs better in compilation time and concurrent processing, while C has more advantages in running speed and memory management. 1.Golang has fast compilation speed and is suitable for rapid development. 2.C runs fast and is suitable for performance-critical applications. 3. Golang is simple and efficient in concurrent processing, suitable for concurrent programming. 4.C Manual memory management provides higher performance, but increases development complexity.
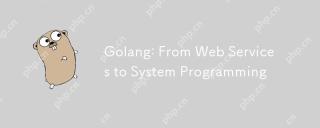
Golang's application in web services and system programming is mainly reflected in its simplicity, efficiency and concurrency. 1) In web services, Golang supports the creation of high-performance web applications and APIs through powerful HTTP libraries and concurrent processing capabilities. 2) In system programming, Golang uses features close to hardware and compatibility with C language to be suitable for operating system development and embedded systems.
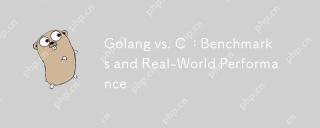
Golang and C have their own advantages and disadvantages in performance comparison: 1. Golang is suitable for high concurrency and rapid development, but garbage collection may affect performance; 2.C provides higher performance and hardware control, but has high development complexity. When making a choice, you need to consider project requirements and team skills in a comprehensive way.
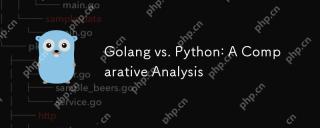
Golang is suitable for high-performance and concurrent programming scenarios, while Python is suitable for rapid development and data processing. 1.Golang emphasizes simplicity and efficiency, and is suitable for back-end services and microservices. 2. Python is known for its concise syntax and rich libraries, suitable for data science and machine learning.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
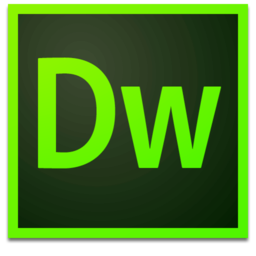
Dreamweaver Mac version
Visual web development tools
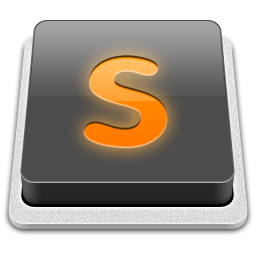
SublimeText3 Mac version
God-level code editing software (SublimeText3)

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
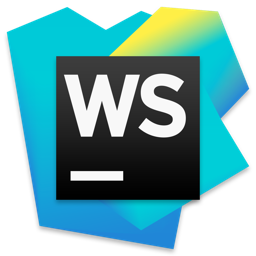
WebStorm Mac version
Useful JavaScript development tools