


How to optimize the efficiency of Go language input functions in practice
Title: How to optimize the efficiency of Go language input functions in practice
In daily Go language development work, the efficiency of input functions often affects the performance of the entire program . This article will introduce how to optimize the efficiency of the Go language input function in practice, and explain the optimization method through specific code examples.
Choose the appropriate input method
First of all, you need to choose the appropriate input method according to actual needs. Normally, the most common input method in the Go language is to obtain data through command line parameters or standard input. When determining the input method, factors such as data volume and data format need to be considered. For example, for large amounts of data input, consider using file input instead of command line parameters.
Reasonable design of data structure
When processing input data, designing a reasonable data structure can greatly improve the operating efficiency of the program. It is necessary to choose a suitable data structure based on the characteristics of the input data, such as using map, slice, etc. When designing the data structure, you need to consider the frequency of data reading and writing to avoid frequent memory allocation and copy operations.
Optimize reading method
In the Go language, you can use bufio in the standard library to optimize the efficiency of the input function. Bufio provides a buffered reading function, which can reduce the number of disk or network I/O and improve data reading speed. The following is a sample code:
package main import ( "bufio" "os" ) func main() { file, err := os.Open("input.txt") if err != nil { panic(err) } defer file.Close() scanner := bufio.NewScanner(file) for scanner.Scan() { line := scanner.Text() // 处理每一行数据 } }
Concurrent processing of data
For large-scale data input processing, you can consider using the concurrency features of the Go language to improve processing efficiency. Through the cooperation of goroutine and channel, concurrent reading and processing of data can be achieved. The following is a simple example of concurrent processing:
package main import ( "fmt" "sync" ) func process(data string, wg *sync.WaitGroup) { defer wg.Done() // 处理数据逻辑 fmt.Println("处理数据:", data) } func main() { data := []string{"data1", "data2", "data3", "data4", "data5"} var wg sync.WaitGroup wg.Add(len(data)) for _, d := range data { go process(d, &wg) } wg.Wait() }
Avoid repeated calculations
In the input function, repeated calculations may sometimes occur, resulting in reduced program efficiency. In order to avoid repeated calculations, caches and other methods can be used to store intermediate calculation results and avoid repeated operations. This not only improves program efficiency, but also saves computing resources. The following is a simple cache example:
package main import ( "fmt" "sync" ) var cache = make(map[int]int) var mu sync.Mutex func fib(n int) int { if n <= 1 { return n } mu.Lock() defer mu.Unlock() if val, ok := cache[n]; ok { return val } cache[n] = fib(n-1) + fib(n-2) return cache[n] } func main() { fmt.Println(fib(10)) }
By reasonably selecting the input method, optimizing the data structure design, using the bufio library to optimize the reading method, processing data concurrently and avoiding repeated calculations, the Go language can be effectively improved. The efficiency of the input function makes the program run more efficiently and stably. Hope the above content is helpful to you.
The above is the detailed content of How to optimize the efficiency of Go language input functions in practice. For more information, please follow other related articles on the PHP Chinese website!
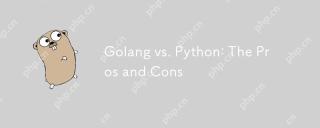
Golangisidealforbuildingscalablesystemsduetoitsefficiencyandconcurrency,whilePythonexcelsinquickscriptinganddataanalysisduetoitssimplicityandvastecosystem.Golang'sdesignencouragesclean,readablecodeanditsgoroutinesenableefficientconcurrentoperations,t
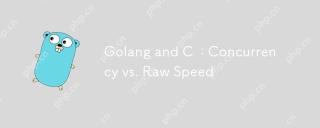
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
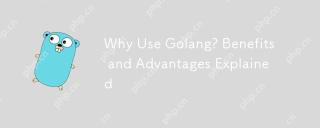
Reasons for choosing Golang include: 1) high concurrency performance, 2) static type system, 3) garbage collection mechanism, 4) rich standard libraries and ecosystems, which make it an ideal choice for developing efficient and reliable software.
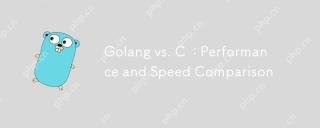
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
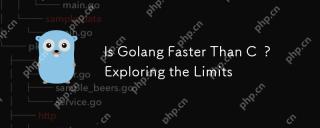
Golang performs better in compilation time and concurrent processing, while C has more advantages in running speed and memory management. 1.Golang has fast compilation speed and is suitable for rapid development. 2.C runs fast and is suitable for performance-critical applications. 3. Golang is simple and efficient in concurrent processing, suitable for concurrent programming. 4.C Manual memory management provides higher performance, but increases development complexity.
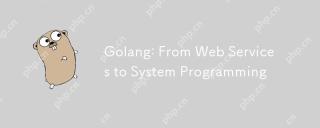
Golang's application in web services and system programming is mainly reflected in its simplicity, efficiency and concurrency. 1) In web services, Golang supports the creation of high-performance web applications and APIs through powerful HTTP libraries and concurrent processing capabilities. 2) In system programming, Golang uses features close to hardware and compatibility with C language to be suitable for operating system development and embedded systems.
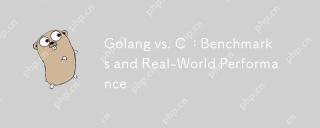
Golang and C have their own advantages and disadvantages in performance comparison: 1. Golang is suitable for high concurrency and rapid development, but garbage collection may affect performance; 2.C provides higher performance and hardware control, but has high development complexity. When making a choice, you need to consider project requirements and team skills in a comprehensive way.
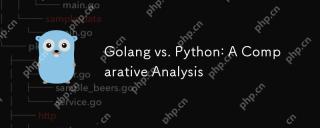
Golang is suitable for high-performance and concurrent programming scenarios, while Python is suitable for rapid development and data processing. 1.Golang emphasizes simplicity and efficiency, and is suitable for back-end services and microservices. 2. Python is known for its concise syntax and rich libraries, suitable for data science and machine learning.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.