The Linux process entering sleep refers to the process of transitioning the process from running state to sleep state. In Linux systems, there are many reasons why a process goes to sleep, including waiting for certain resources, waiting for I/O operations to complete, waiting for signals, etc. In this article, we will explore some common reasons why Linux processes go to sleep and illustrate them with concrete code examples.
Resource waiting
A process may enter sleep state because it needs certain resources, such as waiting for other processes to release a shared resource. In the following example, we create two child processes. One process obtains resources first, and the other process waits for the first process to release the resources before it can continue execution.
#include <stdio.h> #include <stdlib.h> #include <unistd.h> #include <sys/wait.h> #include <sys/types.h> int main() { int fd[2]; pipe(fd); pid_t pid1 = fork(); if (pid1 == 0) { // Child process 1 close(fd[0]); // Close the read port sleep(2); // Simulate the process of obtaining resources close(fd[1]); // Release resources exit(0); } pid_t pid2 = fork(); if (pid2 == 0) { // Child process 2 close(fd[1]); // Close the write port printf("Subprocess 2 is waiting for resources... "); char buf[10]; read(fd[0], buf, sizeof(buf)); // Block waiting for resources printf("Subprocess 2 obtains resources and continues execution. "); exit(0); } // Wait for the child process to end wait(NULL); wait(NULL); return 0; }
In the above code, child process 2 is blocked at the read()
function and cannot continue execution until child process 1 releases resources.
I/O operations
The process may also enter sleep state because it needs to perform I/O operations. The following is a simple example showing a process waiting for user input.
#include <stdio.h> #include <unistd.h> int main() { char buf[10]; printf("Please enter some content: "); fgets(buf, sizeof(buf), stdin); // Block waiting for user input printf("What you entered is: %s", buf); return 0; }
In the above example, the fgets()
function will always wait for user input.
Signal waiting
The process may also enter sleep state due to waiting for signals. The following example shows a process waiting for a signal.
#include <stdio.h> #include <unistd.h> #include <signal.h> void signal_handler(int signal) { printf("Signal received: %d ", signal); } int main() { signal(SIGUSR1, signal_handler); // Register signal processing function printf("Waiting for signal... "); pause(); // The process keeps waiting for the signal return 0; }
In the above example, the process waits for the arrival of the signal through the pause()
function.
Through the above code examples, we can see that there are many reasons why a Linux process goes to sleep, including waiting for certain resources, waiting for I/O operations to complete, waiting for signals, etc. These are important aspects of process scheduling and operation in Linux systems. An in-depth understanding of these principles can help us better understand the operating mechanism of the process.
The above is the detailed content of Explore why Linux processes go to sleep. For more information, please follow other related articles on the PHP Chinese website!
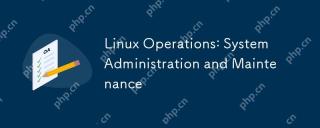
The key steps in Linux system management and maintenance include: 1) Master the basic knowledge, such as file system structure and user management; 2) Carry out system monitoring and resource management, use top, htop and other tools; 3) Use system logs to troubleshoot, use journalctl and other tools; 4) Write automated scripts and task scheduling, use cron tools; 5) implement security management and protection, configure firewalls through iptables; 6) Carry out performance optimization and best practices, adjust kernel parameters and develop good habits.
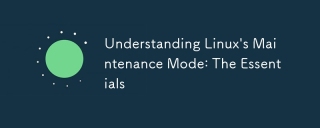
Linux maintenance mode is entered by adding init=/bin/bash or single parameters at startup. 1. Enter maintenance mode: Edit the GRUB menu and add startup parameters. 2. Remount the file system to read and write mode: mount-oremount,rw/. 3. Repair the file system: Use the fsck command, such as fsck/dev/sda1. 4. Back up the data and operate with caution to avoid data loss.
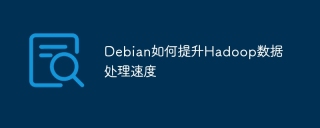
This article discusses how to improve Hadoop data processing efficiency on Debian systems. Optimization strategies cover hardware upgrades, operating system parameter adjustments, Hadoop configuration modifications, and the use of efficient algorithms and tools. 1. Hardware resource strengthening ensures that all nodes have consistent hardware configurations, especially paying attention to CPU, memory and network equipment performance. Choosing high-performance hardware components is essential to improve overall processing speed. 2. Operating system tunes file descriptors and network connections: Modify the /etc/security/limits.conf file to increase the upper limit of file descriptors and network connections allowed to be opened at the same time by the system. JVM parameter adjustment: Adjust in hadoop-env.sh file
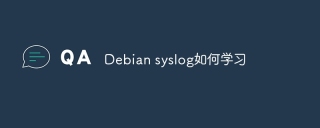
This guide will guide you to learn how to use Syslog in Debian systems. Syslog is a key service in Linux systems for logging system and application log messages. It helps administrators monitor and analyze system activity to quickly identify and resolve problems. 1. Basic knowledge of Syslog The core functions of Syslog include: centrally collecting and managing log messages; supporting multiple log output formats and target locations (such as files or networks); providing real-time log viewing and filtering functions. 2. Install and configure Syslog (using Rsyslog) The Debian system uses Rsyslog by default. You can install it with the following command: sudoaptupdatesud
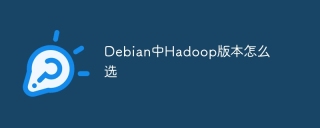
When choosing a Hadoop version suitable for Debian system, the following key factors need to be considered: 1. Stability and long-term support: For users who pursue stability and security, it is recommended to choose a Debian stable version, such as Debian11 (Bullseye). This version has been fully tested and has a support cycle of up to five years, which can ensure the stable operation of the system. 2. Package update speed: If you need to use the latest Hadoop features and features, you can consider Debian's unstable version (Sid). However, it should be noted that unstable versions may have compatibility issues and stability risks. 3. Community support and resources: Debian has huge community support, which can provide rich documentation and

This article describes how to use TigerVNC to share files on Debian systems. You need to install the TigerVNC server first and then configure it. 1. Install the TigerVNC server and open the terminal. Update the software package list: sudoaptupdate to install TigerVNC server: sudoaptinstalltigervnc-standalone-servertigervnc-common 2. Configure TigerVNC server to set VNC server password: vncpasswd Start VNC server: vncserver:1-localhostno
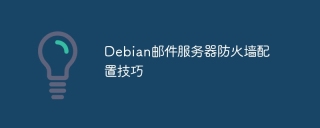
Configuring a Debian mail server's firewall is an important step in ensuring server security. The following are several commonly used firewall configuration methods, including the use of iptables and firewalld. Use iptables to configure firewall to install iptables (if not already installed): sudoapt-getupdatesudoapt-getinstalliptablesView current iptables rules: sudoiptables-L configuration
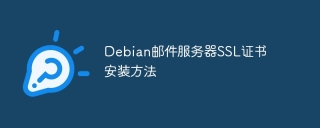
The steps to install an SSL certificate on the Debian mail server are as follows: 1. Install the OpenSSL toolkit First, make sure that the OpenSSL toolkit is already installed on your system. If not installed, you can use the following command to install: sudoapt-getupdatesudoapt-getinstallopenssl2. Generate private key and certificate request Next, use OpenSSL to generate a 2048-bit RSA private key and a certificate request (CSR): openss


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use
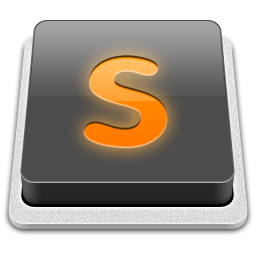
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
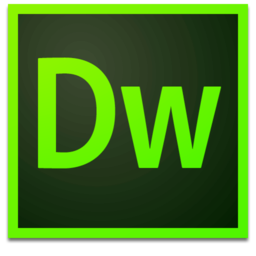
Dreamweaver Mac version
Visual web development tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool