


Explore the role and necessity of abstract methods in PHP classes
Title: Exploring the role and necessity of abstract methods in PHP classes
Abstract methods are an important concept in object-oriented programming, which play a role in PHP classes plays a key role. This article will deeply explore the role and necessity of abstract methods in PHP classes, and demonstrate its usage and advantages through specific code examples.
What is an abstract method?
In PHP, abstract methods refer to methods defined in abstract classes without specific implementation. Abstract methods must be implemented in the subclass, otherwise the subclass must also be declared as an abstract class. By defining abstract methods, we can require subclasses to implement these methods, thereby ensuring class consistency and scalability.
The role of abstract methods
- Forcing subclasses to implement methods: Abstract methods require subclasses to implement these methods to ensure interface consistency between parent classes and subclasses.
- Improve the logic and readability of the code: Through abstract methods, we can define the behavior of the class more clearly, making the code logic clearer and easier to understand.
- Achieve polymorphism: The existence of abstract methods allows different subclasses to implement methods differently according to their own needs to achieve polymorphism.
The necessity of abstract methods
- Interface specification: Abstract method ensures that the class follows certain interface specifications, which helps to better organize and maintain the code.
- Code reuse: Through abstract methods, we can define common behaviors to facilitate reuse in different classes.
- Extensibility: Abstract methods provide good extensibility and can add new behaviors to existing classes without modifying the underlying code.
Code Example
<?php //Define an abstract class Animal abstract class Animal { // Abstract method speak, subclasses must implement this method abstract public function speak(); } //Define a subclass Dog, inherited from Animal class Dog extends Animal { // Implement the abstract method speak public function speak() { echo "woof woof woof "; } } //Define a subclass Cat, inherited from Animal class Cat extends Animal { // Implement the abstract method speak public function speak() { echo "meow meow meow "; } } //Create a Dog instance $dog = new Dog(); $dog->speak(); // Output: woof woof woof //Create a Cat instance $cat = new Cat(); $cat->speak(); // Output: meow meow meow ?>
In the above code example, an abstract class Animal is defined, and an abstract method speak is defined in it. The subclasses Dog and Cat inherit from Animal and implement the speak method respectively. Through the use of abstract methods, we can see the flexibility and diversity of different subclasses when implementing the same method.
Conclusion
Abstract method is an important concept in PHP object-oriented programming. It can improve the logic, readability and maintainability of the code. It also has interface specifications and code reuse. and scalability. Reasonable use of abstract methods can make our code clearer, more flexible and scalable, and is an excellent programming practice.
The above is the detailed content of Explore the role and necessity of abstract methods in PHP classes. For more information, please follow other related articles on the PHP Chinese website!
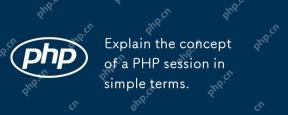
PHPsessionstrackuserdataacrossmultiplepagerequestsusingauniqueIDstoredinacookie.Here'showtomanagethemeffectively:1)Startasessionwithsession_start()andstoredatain$_SESSION.2)RegeneratethesessionIDafterloginwithsession_regenerate_id(true)topreventsessi
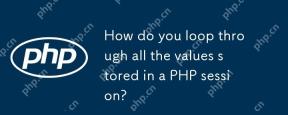
In PHP, iterating through session data can be achieved through the following steps: 1. Start the session using session_start(). 2. Iterate through foreach loop through all key-value pairs in the $_SESSION array. 3. When processing complex data structures, use is_array() or is_object() functions and use print_r() to output detailed information. 4. When optimizing traversal, paging can be used to avoid processing large amounts of data at one time. This will help you manage and use PHP session data more efficiently in your actual project.
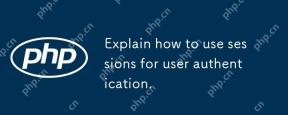
The session realizes user authentication through the server-side state management mechanism. 1) Session creation and generation of unique IDs, 2) IDs are passed through cookies, 3) Server stores and accesses session data through IDs, 4) User authentication and status management are realized, improving application security and user experience.
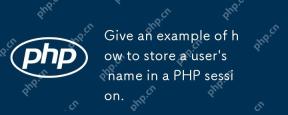
Tostoreauser'snameinaPHPsession,startthesessionwithsession_start(),thenassignthenameto$_SESSION['username'].1)Usesession_start()toinitializethesession.2)Assigntheuser'snameto$_SESSION['username'].Thisallowsyoutoaccessthenameacrossmultiplepages,enhanc
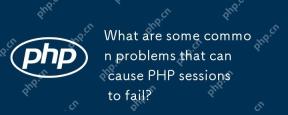
Reasons for PHPSession failure include configuration errors, cookie issues, and session expiration. 1. Configuration error: Check and set the correct session.save_path. 2.Cookie problem: Make sure the cookie is set correctly. 3.Session expires: Adjust session.gc_maxlifetime value to extend session time.
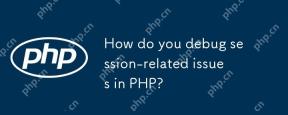
Methods to debug session problems in PHP include: 1. Check whether the session is started correctly; 2. Verify the delivery of the session ID; 3. Check the storage and reading of session data; 4. Check the server configuration. By outputting session ID and data, viewing session file content, etc., you can effectively diagnose and solve session-related problems.
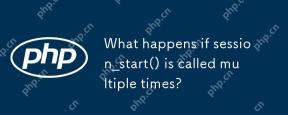
Multiple calls to session_start() will result in warning messages and possible data overwrites. 1) PHP will issue a warning, prompting that the session has been started. 2) It may cause unexpected overwriting of session data. 3) Use session_status() to check the session status to avoid repeated calls.
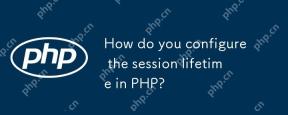
Configuring the session lifecycle in PHP can be achieved by setting session.gc_maxlifetime and session.cookie_lifetime. 1) session.gc_maxlifetime controls the survival time of server-side session data, 2) session.cookie_lifetime controls the life cycle of client cookies. When set to 0, the cookie expires when the browser is closed.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

Atom editor mac version download
The most popular open source editor
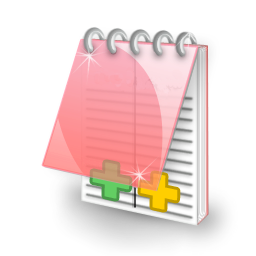
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SublimeText3 English version
Recommended: Win version, supports code prompts!

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
