Explore the secrets of the basic units of C language
To explore the secrets of the basic units of C language, specific code examples are needed
C language is a language widely used in system programming and low-level development The basic units of high-level programming language include variables, data types, operators, etc. The use of these basic units is the key to understanding the core mechanism of C language. This article will delve into the basic units of the C language and reveal its secrets through actual code examples.
1. Variables
In C language, variables are the basic unit for storing data in the program. They can be various data types such as integer, floating point, character, etc. Variables need to be declared before use, and their values can be modified at any time. Here's a simple example:
#include <stdio.h> int main() { int a = 10; float b = 3.14; char c = 'A'; printf("The value of integer variable a is: %d ", a); printf("The value of floating point variable b is: %.2f ", b); printf("The value of character variable c is: %c ", c); return 0; }
In the above code, three variables a, b, and c are defined and assigned different values respectively, and then the values of the variables are output through the printf function, thus demonstrating the basic usage of variables.
2. Data types
C language provides a variety of data types, including basic data types and derived data types. Basic data types include integers, floating point types, character types, etc., while derived data types include arrays, structures, pointers, etc. Different data types occupy different amounts of space in memory, so when selecting a data type, you need to make a reasonable choice based on actual needs.
#include <stdio.h> int main() { int x = 10; float y = 3.14; char z = 'A'; printf("Number of bytes occupied by integer variable x: %d ", sizeof(x)); printf("The number of bytes occupied by floating point variable y: %d ", sizeof(y)); printf("Number of bytes occupied by character variable z: %d ", sizeof(z)); return 0; }
In the above example, the sizeof operator can be used to obtain the number of bytes occupied by variables of different data types, and then understand how the data types are stored in memory.
3. Operators
C language provides a wealth of operators, including arithmetic operators, relational operators, logical operators, etc. These operators can perform various operations on variables. achieve different computing purposes. Here's a simple example:
#include <stdio.h> int main() { int a = 5, b = 3; printf("a b = %d ", a b); printf("a - b = %d ", a - b); printf("a * b = %d ", a * b); printf("a / b = %d ", a / b); return 0; }
The above code shows four basic arithmetic operations, using operators to calculate variables a and b and output the results.
Summary:
Through the above code examples, we have deeply explored the basic units of C language-variables, data types, operators, and revealed their mysteries. When writing C language programs, it is crucial to master these basic units. Only by deeply understanding their principles and usage can we write more efficient and stable programs. It is hoped that readers will have a deeper understanding of the basic units of C language through the introduction and sample code of this article, so that they can use it freely in daily programming practice.
The above is the detailed content of Explore the secrets of the basic units of C language. For more information, please follow other related articles on the PHP Chinese website!
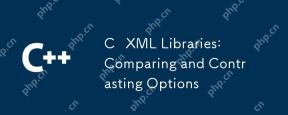
There are four commonly used XML libraries in C: TinyXML-2, PugiXML, Xerces-C, and RapidXML. 1.TinyXML-2 is suitable for environments with limited resources, lightweight but limited functions. 2. PugiXML is fast and supports XPath query, suitable for complex XML structures. 3.Xerces-C is powerful, supports DOM and SAX resolution, and is suitable for complex processing. 4. RapidXML focuses on performance and parses extremely fast, but does not support XPath queries.
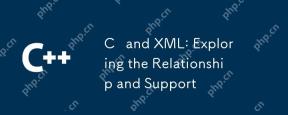
C interacts with XML through third-party libraries (such as TinyXML, Pugixml, Xerces-C). 1) Use the library to parse XML files and convert them into C-processable data structures. 2) When generating XML, convert the C data structure to XML format. 3) In practical applications, XML is often used for configuration files and data exchange to improve development efficiency.
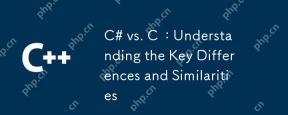
The main differences between C# and C are syntax, performance and application scenarios. 1) The C# syntax is more concise, supports garbage collection, and is suitable for .NET framework development. 2) C has higher performance and requires manual memory management, which is often used in system programming and game development.
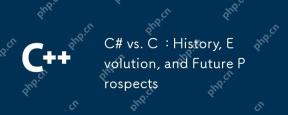
The history and evolution of C# and C are unique, and the future prospects are also different. 1.C was invented by BjarneStroustrup in 1983 to introduce object-oriented programming into the C language. Its evolution process includes multiple standardizations, such as C 11 introducing auto keywords and lambda expressions, C 20 introducing concepts and coroutines, and will focus on performance and system-level programming in the future. 2.C# was released by Microsoft in 2000. Combining the advantages of C and Java, its evolution focuses on simplicity and productivity. For example, C#2.0 introduced generics and C#5.0 introduced asynchronous programming, which will focus on developers' productivity and cloud computing in the future.
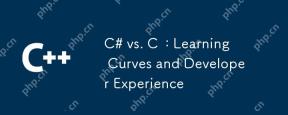
There are significant differences in the learning curves of C# and C and developer experience. 1) The learning curve of C# is relatively flat and is suitable for rapid development and enterprise-level applications. 2) The learning curve of C is steep and is suitable for high-performance and low-level control scenarios.
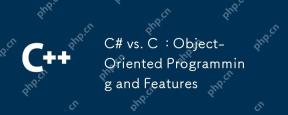
There are significant differences in how C# and C implement and features in object-oriented programming (OOP). 1) The class definition and syntax of C# are more concise and support advanced features such as LINQ. 2) C provides finer granular control, suitable for system programming and high performance needs. Both have their own advantages, and the choice should be based on the specific application scenario.
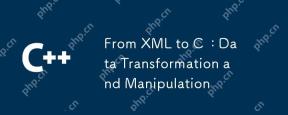
Converting from XML to C and performing data operations can be achieved through the following steps: 1) parsing XML files using tinyxml2 library, 2) mapping data into C's data structure, 3) using C standard library such as std::vector for data operations. Through these steps, data converted from XML can be processed and manipulated efficiently.
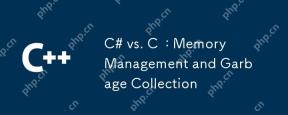
C# uses automatic garbage collection mechanism, while C uses manual memory management. 1. C#'s garbage collector automatically manages memory to reduce the risk of memory leakage, but may lead to performance degradation. 2.C provides flexible memory control, suitable for applications that require fine management, but should be handled with caution to avoid memory leakage.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
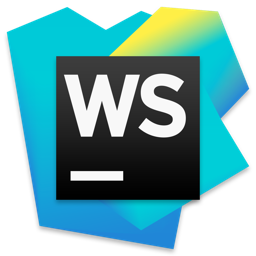
WebStorm Mac version
Useful JavaScript development tools

Atom editor mac version download
The most popular open source editor
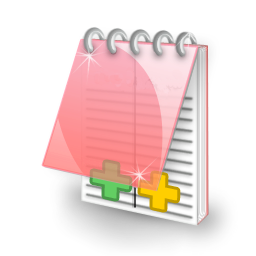
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software