The principle and application of file locking in Golang
The principle and application of file locking in Golang
In the operating system, file locking is a method used to protect files or resources from being accessed by multiple processes at the same time or Modified mechanism. In Golang, the data structure in memory can be locked by using the Mutex
lock provided by the sync
package, but when multiple processes are involved in reading and writing the same file During operation, file locks need to be used to ensure data consistency and security.
Types of file locks
In Golang, you can use the FcntlFlock
method of the File
structure provided by the os
package To implement file locks, which include the following file lock types:
-
F_RDLCK
: Read lock, used to prevent other processes from writing files. -
F_WRLCK
: Write lock, used to prevent other processes from reading or writing files. -
F_UNLCK
: Unlock, used to release file lock.
Principle of file lock
The implementation principle of file lock is to lock files through the fcntl
system call provided by the operating system. When a process acquires a file lock, other processes that try to acquire the same lock will be blocked until the lock is released.
Golang implements file lock sample code
The following is a simple sample code that demonstrates how to implement file lock application in Golang:
package main import ( "os" "syscall" ) func main() { file, err := os.OpenFile("test.txt", os.O_RDWR|os.O_CREATE, 0666) if err != nil { panic(err) } defer file.Close() // 获取文件锁 err = syscall.Flock(int(file.Fd()), syscall.LOCK_EX) if err != nil { panic(err) } defer syscall.Flock(int(file.Fd()), syscall.LOCK_UN) // 在文件中写入数据 _, err = file.Write([]byte("Hello, World!")) if err != nil { panic(err) } }
In the above code , we first open a file named test.txt
through the os.OpenFile
method, and then lock the file through the syscall.Flock
method, here syscall.LOCK_EX
is used to indicate write lock. After writing data, release the file lock through the defer
statement.
Application scenarios of file locks
File locks are widely used in situations where multiple processes share resources, such as when multiple processes write log files and read and write configuration files at the same time. By using file locks, data conflicts and inconsistencies caused by multiple processes on the same file can be effectively avoided.
In short, file lock is a powerful mechanism that can ensure safe access and manipulation of files. In Golang, the file lock function can be easily implemented through the File
structure provided by the os
package and the FcntlFlock
method provided by the syscall
package. . When writing applications that share resources among multiple processes, be sure to consider using file locks to ensure data consistency and security.
The above is the detailed content of The principle and application of file locking in Golang. For more information, please follow other related articles on the PHP Chinese website!
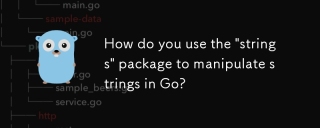
The article discusses using Go's "strings" package for string manipulation, detailing common functions and best practices to enhance efficiency and handle Unicode effectively.
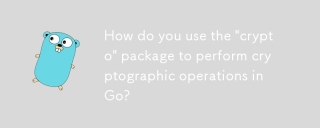
The article details using Go's "crypto" package for cryptographic operations, discussing key generation, management, and best practices for secure implementation.Character count: 159
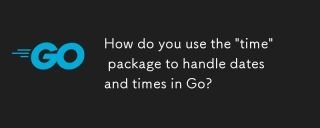
The article details the use of Go's "time" package for handling dates, times, and time zones, including getting current time, creating specific times, parsing strings, and measuring elapsed time.
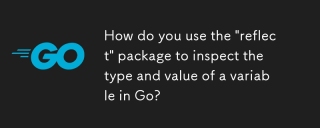
Article discusses using Go's "reflect" package for variable inspection and modification, highlighting methods and performance considerations.
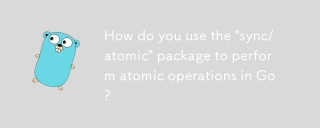
The article discusses using Go's "sync/atomic" package for atomic operations in concurrent programming, detailing its benefits like preventing race conditions and improving performance.
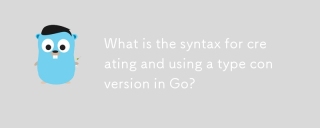
The article discusses type conversions in Go, including syntax, safe conversion practices, common pitfalls, and learning resources. It emphasizes explicit type conversion and error handling.[159 characters]
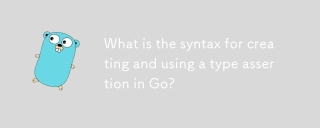
The article discusses type assertions in Go, focusing on syntax, potential errors like panics and incorrect types, safe handling methods, and performance implications.
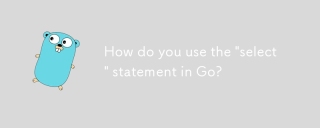
The article explains the use of the "select" statement in Go for handling multiple channel operations, its differences from the "switch" statement, and common use cases like handling multiple channels, implementing timeouts, non-b


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
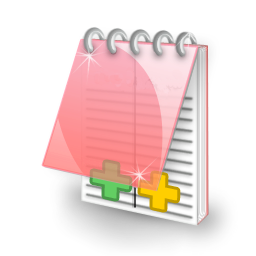
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SublimeText3 English version
Recommended: Win version, supports code prompts!

SublimeText3 Linux new version
SublimeText3 Linux latest version

Notepad++7.3.1
Easy-to-use and free code editor
