


How do you use the "sync/atomic" package to perform atomic operations in Go?
How do you use the "sync/atomic" package to perform atomic operations in Go?
The "sync/atomic" package in Go provides low-level atomic memory primitives useful for implementing synchronization algorithms. These operations are crucial in concurrent programming to ensure that updates to shared variables are performed atomically, meaning they are executed as a single, uninterruptible unit of work. Here's how you can use it:
-
Import the Package:
First, you need to import the "sync/atomic" package in your Go program:import "sync/atomic"
-
Choose the Appropriate Data Type:
The "sync/atomic" package supports atomic operations on several data types, includingint32
,int64
,uint32
,uint64
,uintptr
, andunsafe.Pointer
. You need to choose the appropriate type for your use case. -
Perform Atomic Operations:
You can perform various atomic operations such asLoad
,Store
,Add
,Swap
, andCompareAndSwap
. Here are examples of these operations:-
Load:
var value int32 loadedValue := atomic.LoadInt32(&value)
This operation atomically reads the value of
value
. -
Store:
var value int32 atomic.StoreInt32(&value, 5)
This operation atomically stores the value 5 into
value
. -
Add:
var value int32 newValue := atomic.AddInt32(&value, 3)
This operation atomically adds 3 to
value
and returns the new value. -
Swap:
var value int32 oldValue := atomic.SwapInt32(&value, 10)
This operation atomically stores 10 into
value
and returns the old value. -
CompareAndSwap:
var value int32 swapped := atomic.CompareAndSwapInt32(&value, 0, 1)
This operation atomically compares
value
with 0, and if they are equal, it stores 1 intovalue
. The function returns true if the swap occurred, otherwise false.
-
Using these operations ensures that multiple goroutines can safely access and modify shared variables without race conditions.
What are the benefits of using atomic operations in Go programs?
Atomic operations offer several benefits in Go programs, particularly in concurrent scenarios:
-
Race Condition Prevention:
Atomic operations ensure that updates to shared variables are executed as a single unit, preventing race conditions where multiple goroutines might interfere with each other's operations on the same variable. -
Improved Performance:
By eliminating the need for locks or mutexes in some scenarios, atomic operations can reduce the overhead associated with locking mechanisms, potentially leading to better performance in concurrent applications. -
Thread Safety:
Atomic operations inherently provide thread safety, as they guarantee that operations on shared variables are atomic and cannot be interrupted by other goroutines. -
Simplified Code:
In some cases, using atomic operations can simplify code by removing the need for more complex synchronization mechanisms like mutexes or channels. -
Fine-Grained Control:
Atomic operations allow for fine-grained control over shared state, enabling developers to precisely control which operations need to be atomic, potentially optimizing the performance of their applications.
How can atomic operations improve the performance of concurrent Go applications?
Atomic operations can significantly enhance the performance of concurrent Go applications in several ways:
-
Reduced Lock Contention:
Atomic operations can eliminate the need for locks in many scenarios, reducing the contention that occurs when multiple goroutines are competing for the same lock. This can lead to better scalability and throughput in concurrent applications. -
Lower Overhead:
The overhead of atomic operations is generally lower compared to using mutexes or channels, as they involve less context switching and do not require the acquisition and release of locks. -
Efficient Memory Access:
Atomic operations are designed to be as efficient as possible at the CPU level, often using special instructions provided by the hardware to perform operations quickly and safely. -
Avoiding Deadlocks:
By minimizing the use of locks, atomic operations can help avoid deadlocks, which can severely impact the performance and reliability of concurrent applications. -
Optimized Resource Utilization:
By allowing multiple goroutines to operate on shared data without significant overhead, atomic operations can lead to better resource utilization and improved overall system performance.
Which specific functions in the "sync/atomic" package are most useful for managing shared state?
Several functions in the "sync/atomic" package are particularly useful for managing shared state in Go applications:
-
LoadInt32, LoadInt64, LoadUint32, LoadUint64, LoadUintptr, LoadPointer:
These functions are used to atomically read values from shared variables. They are essential for safely accessing shared state without the risk of race conditions. -
StoreInt32, StoreInt64, StoreUint32, StoreUint64, StoreUintptr, StorePointer:
These functions allow for the atomic storage of values into shared variables. They ensure that the value is stored as a single, uninterruptible operation. -
AddInt32, AddInt64, AddUint32, AddUint64:
These functions atomically add a value to a shared variable and return the new value. They are useful for scenarios such as counters or incrementing/decrementing shared values. -
SwapInt32, SwapInt64, SwapUint32, SwapUint64, SwapUintptr, SwapPointer:
These functions atomically swap a value with a new value in a shared variable and return the old value. They are useful for scenarios where you need to atomically replace a value and retrieve the original one. -
CompareAndSwapInt32, CompareAndSwapInt64, CompareAndSwapUint32, CompareAndSwapUint64, CompareAndSwapUintptr, CompareAndSwapPointer:
These functions atomically compare a shared variable with a given value and, if they match, store a new value. They are particularly useful for implementing optimistic locking or for scenarios requiring conditional updates.
Using these functions, developers can effectively manage shared state in concurrent Go applications, ensuring safety and efficiency.
The above is the detailed content of How do you use the "sync/atomic" package to perform atomic operations in Go?. For more information, please follow other related articles on the PHP Chinese website!
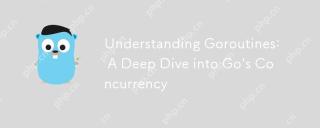
GoroutinesarefunctionsormethodsthatrunconcurrentlyinGo,enablingefficientandlightweightconcurrency.1)TheyaremanagedbyGo'sruntimeusingmultiplexing,allowingthousandstorunonfewerOSthreads.2)Goroutinesimproveperformancethrougheasytaskparallelizationandeff
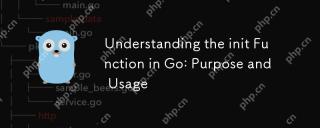
ThepurposeoftheinitfunctioninGoistoinitializevariables,setupconfigurations,orperformnecessarysetupbeforethemainfunctionexecutes.Useinitby:1)Placingitinyourcodetorunautomaticallybeforemain,2)Keepingitshortandfocusedonsimpletasks,3)Consideringusingexpl
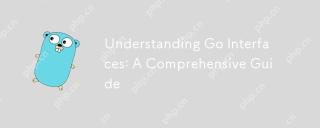
Gointerfacesaremethodsignaturesetsthattypesmustimplement,enablingpolymorphismwithoutinheritanceforcleaner,modularcode.Theyareimplicitlysatisfied,usefulforflexibleAPIsanddecoupling,butrequirecarefulusetoavoidruntimeerrorsandmaintaintypesafety.
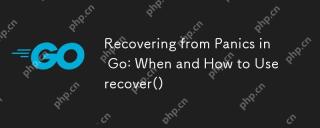
Use the recover() function in Go to recover from panic. The specific methods are: 1) Use recover() to capture panic in the defer function to avoid program crashes; 2) Record detailed error information for debugging; 3) Decide whether to resume program execution based on the specific situation; 4) Use with caution to avoid affecting performance.
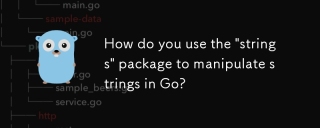
The article discusses using Go's "strings" package for string manipulation, detailing common functions and best practices to enhance efficiency and handle Unicode effectively.
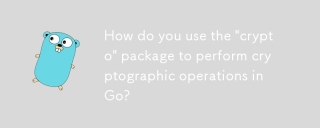
The article details using Go's "crypto" package for cryptographic operations, discussing key generation, management, and best practices for secure implementation.Character count: 159
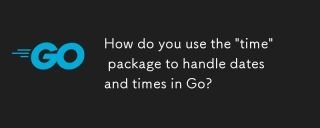
The article details the use of Go's "time" package for handling dates, times, and time zones, including getting current time, creating specific times, parsing strings, and measuring elapsed time.
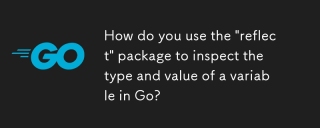
Article discusses using Go's "reflect" package for variable inspection and modification, highlighting methods and performance considerations.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
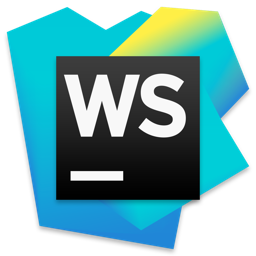
WebStorm Mac version
Useful JavaScript development tools
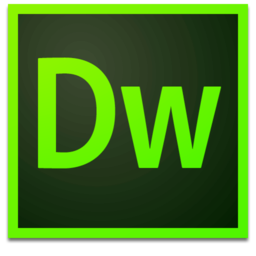
Dreamweaver Mac version
Visual web development tools
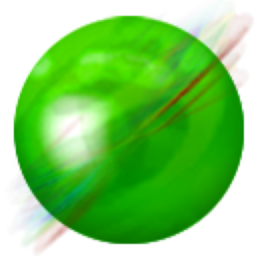
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
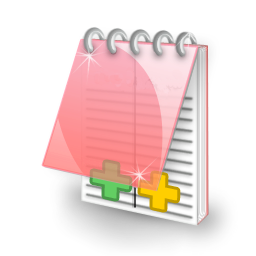
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
