How do you use the "strings" package to manipulate strings in Go?
To use the "strings" package for string manipulation in Go, you first need to import it. You can do this by adding the following line at the top of your Go file:
import "strings"
Once the package is imported, you can use its various functions to perform operations on strings. Here's a basic example of using the strings.ToUpper()
function to convert a string to uppercase:
package main import ( "fmt" "strings" ) func main() { originalString := "hello, world!" upperString := strings.ToUpper(originalString) fmt.Println(upperString) // Output: HELLO, WORLD! }
In this example, strings.ToUpper()
is used to convert the string "hello, world!" to "HELLO, WORLD!". The strings
package provides many other functions that can be used in a similar manner to manipulate strings according to your needs.
What are some common functions in the "strings" package for string manipulation in Go?
The "strings" package in Go offers a wide range of functions for string manipulation. Some of the most commonly used functions include:
-
strings.Contains(s, substr string) bool:
This function checks if the strings
contains the substringsubstr
.fmt.Println(strings.Contains("test", "es")) // Output: true
-
strings.HasPrefix(s, prefix string) bool:
This function checks if the strings
starts with the prefixprefix
.fmt.Println(strings.HasPrefix("test", "te")) // Output: true
-
strings.HasSuffix(s, suffix string) bool:
This function checks if the strings
ends with the suffixsuffix
.fmt.Println(strings.HasSuffix("test", "st")) // Output: true
-
strings.Index(s, substr string) int:
This function returns the index of the first instance ofsubstr
ins
, or -1 ifsubstr
is not present ins
.fmt.Println(strings.Index("test", "es")) // Output: 1
-
strings.Join(a []string, sep string) string:
This function concatenates the elements ofa
to create a single string. The separator stringsep
is placed between elements in the resulting string.fmt.Println(strings.Join([]string{"foo", "bar", "baz"}, ",")) // Output: foo,bar,baz
-
strings.Replace(s, old, new string, n int) string:
This function replaces occurrences ofold
withnew
ins
. Ifn
is -1, there is no limit on the number of replacements.fmt.Println(strings.Replace("oink oink oink", "oink", "moo", 2)) // Output: moo moo oink
-
strings.Split(s, sep string) []string:
This function slicess
into all substrings separated bysep
and returns a slice of the substrings between those separators.fmt.Println(strings.Split("a,b,c", ",")) // Output: [a b c]
-
strings.Trim(s, cutset string) string:
This function returns a slice of the strings
with all leading and trailing Unicode code points contained incutset
removed.fmt.Println(strings.Trim(" !!! Achtung! Achtung! !!! ", "! ")) // Output: Achtung! Achtung
These functions are essential for everyday string manipulation tasks in Go programming.
How can the "strings" package improve string handling efficiency in Go?
The "strings" package in Go is designed to optimize string manipulation tasks, which can significantly improve the efficiency of string handling in several ways:
-
Built-in Optimization:
The functions within the "strings" package are highly optimized and written in Go's native code, which ensures that they perform operations in the most efficient way possible. For example, when usingstrings.Contains()
, the package uses efficient algorithms to quickly check for the existence of a substring. -
Reduced Memory Allocation:
Many functions in the "strings" package are designed to minimize memory allocation. For instance,strings.Join()
can concatenate strings without creating unnecessary intermediate strings, which helps reduce memory usage and improve performance. -
Effective Use of Unicode:
The "strings" package takes into account Unicode characters and their properties, which is crucial for handling text in multiple languages efficiently. Functions likestrings.ToLower()
andstrings.ToUpper()
handle Unicode characters correctly, ensuring that the operations are done accurately and efficiently. -
Bulk Operations:
Functions likestrings.ReplaceAll()
andstrings.Split()
allow for bulk operations, which can be more efficient than performing multiple individual operations. For example,strings.ReplaceAll()
performs the replacement in one pass, which is more efficient than doing multiple calls tostrings.Replace()
with a count of -1. -
In-place Modifications:
Some functions in the "strings" package enable in-place modifications, which can reduce the need for creating new strings. This can be particularly beneficial in scenarios where memory efficiency is a priority.
By leveraging the optimized functions provided by the "strings" package, Go developers can significantly improve the efficiency of their string manipulation tasks.
What are the best practices for using the "strings" package in Go programming?
To make the most out of the "strings" package and ensure best practices are followed, consider the following recommendations:
-
Choose the Right Function:
Always select the most appropriate function for your task. For instance, usestrings.Contains()
instead of manually iterating over a string to check for a substring. This not only improves efficiency but also makes your code more readable and maintainable. -
Avoid Unnecessary Allocations:
Try to minimize unnecessary string allocations. For example, instead of concatenating strings using thestrings.Builder
orstrings.Join()
to create a single string more efficiently.var builder strings.Builder for i := 0; i < 10; i { builder.WriteString("Hello ") } result := builder.String() fmt.Println(result)
-
Use Constants Where Possible:
When dealing with fixed strings like delimiters or prefixes, define them as constants to improve code clarity and maintainability.const comma = "," fmt.Println(strings.Join([]string{"foo", "bar", "baz"}, comma))
-
Handle Edge Cases:
Always consider edge cases, such as empty strings or strings containing only whitespace. Functions likestrings.TrimSpace()
can be useful for handling whitespace effectively.input := " Hello, World! " trimmed := strings.TrimSpace(input) fmt.Println(trimmed) // Output: Hello, World!
-
Be Mindful of Unicode:
When working with Unicode strings, use functions that are aware of Unicode properties, such asstrings.ToLower()
andstrings.ToUpper()
, to ensure correct handling of international text. -
Document and Comment Your Code:
Use clear and descriptive comments to explain the purpose of using specific "strings" functions, particularly if the reason is not immediately obvious from the code itself.// Remove leading and trailing whitespace from the input string cleaned := strings.TrimSpace(input)
By adhering to these best practices, you can effectively utilize the "strings" package to perform efficient and robust string manipulation in your Go programs.
The above is the detailed content of How do you use the "strings" package to manipulate strings in Go?. For more information, please follow other related articles on the PHP Chinese website!
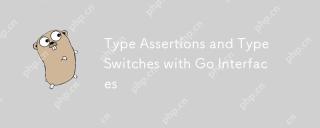
Gohandlesinterfacesandtypeassertionseffectively,enhancingcodeflexibilityandrobustness.1)Typeassertionsallowruntimetypechecking,asseenwiththeShapeinterfaceandCircletype.2)Typeswitcheshandlemultipletypesefficiently,usefulforvariousshapesimplementingthe
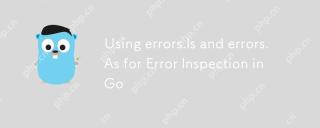
Go language error handling becomes more flexible and readable through errors.Is and errors.As functions. 1.errors.Is is used to check whether the error is the same as the specified error and is suitable for the processing of the error chain. 2.errors.As can not only check the error type, but also convert the error to a specific type, which is convenient for extracting error information. Using these functions can simplify error handling logic, but pay attention to the correct delivery of error chains and avoid excessive dependence to prevent code complexity.
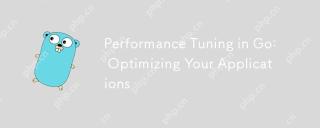
TomakeGoapplicationsrunfasterandmoreefficiently,useprofilingtools,leverageconcurrency,andmanagememoryeffectively.1)UsepprofforCPUandmemoryprofilingtoidentifybottlenecks.2)Utilizegoroutinesandchannelstoparallelizetasksandimproveperformance.3)Implement
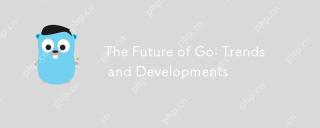
Go'sfutureisbrightwithtrendslikeimprovedtooling,generics,cloud-nativeadoption,performanceenhancements,andWebAssemblyintegration,butchallengesincludemaintainingsimplicityandimprovingerrorhandling.
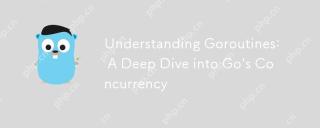
GoroutinesarefunctionsormethodsthatrunconcurrentlyinGo,enablingefficientandlightweightconcurrency.1)TheyaremanagedbyGo'sruntimeusingmultiplexing,allowingthousandstorunonfewerOSthreads.2)Goroutinesimproveperformancethrougheasytaskparallelizationandeff
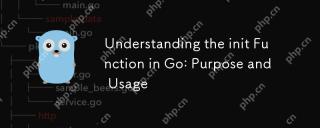
ThepurposeoftheinitfunctioninGoistoinitializevariables,setupconfigurations,orperformnecessarysetupbeforethemainfunctionexecutes.Useinitby:1)Placingitinyourcodetorunautomaticallybeforemain,2)Keepingitshortandfocusedonsimpletasks,3)Consideringusingexpl
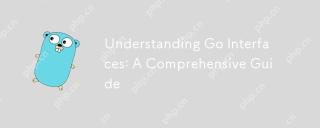
Gointerfacesaremethodsignaturesetsthattypesmustimplement,enablingpolymorphismwithoutinheritanceforcleaner,modularcode.Theyareimplicitlysatisfied,usefulforflexibleAPIsanddecoupling,butrequirecarefulusetoavoidruntimeerrorsandmaintaintypesafety.
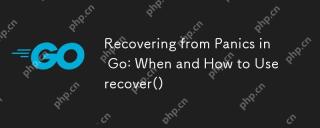
Use the recover() function in Go to recover from panic. The specific methods are: 1) Use recover() to capture panic in the defer function to avoid program crashes; 2) Record detailed error information for debugging; 3) Decide whether to resume program execution based on the specific situation; 4) Use with caution to avoid affecting performance.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
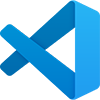
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

Zend Studio 13.0.1
Powerful PHP integrated development environment

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
