Validating emails in PHP is one of the common needs in website development. Effective email verification improves user input accuracy and prevents malicious registrations and information leakage. This article will introduce how to use PHP to write simple and effective email verification code to ensure that the email format entered by the user conforms to the specification. Through the guidance of this article, you can easily implement the email verification function and improve the user experience and security of your website. PHP editor Apple will explain the method of verifying emails in detail, allowing you to easily master the skills.
We will also demonstrate another way to validate emails in php
using FILTER_SANITIZE_EMAIL
and FILTER_VALIDATE_EMAIL
filter name IDs and the fiter_var() function Address method. This method first cleans the email address and then verifies the email address.
We'll cover another way to validate emails in PHP using Regular Expressions. This method uses the preg_match()
function to check whether the email is valid based on the provided regular expression.
Validate email in PHP using filter_var()
function and FILTER_VALIDATE_EMAIL
We can use the filter_var() function to filter variables with a specific filter name.
FILTER_VALIDATE_EMAIL Filter name specifies an email that requires validation. This function takes the email address as a
string as the first argument and the filter ID specified above as the second argument. So we can check if the email provided is valid. If the function succeeds or returns false, the function returns the filtered data. Email is said to be valid, not that email exists. The filter id validates the email based on the syntax in RFC 822. We can test the verification of the email using valid and invalid emails.
validateEmail() that accepts a parameter
$email. Use the
filter_var() function on the
$email variable, specifying the filter ID
FILTER_VALIDATE_EMAIL as the second parameter. Apply
if-else conditions to the
filter_var() function. In the
if block, show the message saying the email is valid, and in the
else condition, show the email is invalid. Outside the function, call the function twice. Provide arguments in the first function call,
peter.piper@iana.org and
first.last@example.123
on the second call.
$_POST variable. The function in the example below is called twice. The first call passes a valid email address, the second an invalid email. The second email address is invalid because it contains a number from the top-level domain. The results are obvious.
<code> <code class="language-php hljs" data-lang="php"><span style="display:flex;"><span><span style="color:#408080;font-style:italic">#php 7.x </span></span></span><span style="display:flex;"><span><span style="color:#666"></span>php </span></span><span style="display:flex;"><span><span style="color:#008000;font-weight:bold">funct<strong class="keylink">io</strong>n</span> <span style="color:#00f">validateEmail</span>(<span style="color:#19177c">$email</span>) { </span></span><span style="display:flex;"><span><span style="color:#008000;font-weight:bold">if</span>(filter_var(<span style="color:#19177c">$email</span>, FILTER_VALIDATE_EMAIL)) { </span></span><span style="display:flex;"><span><span style="color:#008000;font-weight:bold">echo</span> <span style="color:#ba2121">"</span><span style="color:#b68;font-weight:bold">{</span><span style="color:#19177c">$email</span><span style="color:#b68;font-weight:bold">}</span><span style="color:#ba2121">: A valid email"</span><span style="color:#666">.</span><span style="color:#ba2121">"<br>"</span>; </span></span><span style="display:flex;"><span>} </span></span><span style="display:flex;"><span><span style="color:#008000;font-weight:bold">else</span> { </span></span><span style="display:flex;"><span><span style="color:#008000;font-weight:bold">echo</span> <span style="color:#ba2121">"</span><span style="color:#b68;font-weight:bold">{</span><span style="color:#19177c">$email</span><span style="color:#b68;font-weight:bold">}</span><span style="color:#ba2121">: Not a valid email"</span><span style="color:#666">.</span><span style="color:#ba2121">"<br>"</span>; </span></span><span style="display:flex;"><span>} </span></span><span style="display:flex;"><span>} </span></span><span style="display:flex;"><span>validateEmail(<span style="color:#ba2121">'peter.piper@iana.org'</span>); </span></span><span style="display:flex;"><span>validateEmail(<span style="color:#ba2121">'first.last@example.123'</span>); </span></span><span style="display:flex;"><span><span style="color:#bc7a00">?></span><span > </span></span></span></code></code>Output:
<code> <code class="language-php hljs" data-lang="php"><span style="display:flex;"><span>phppeter<span style="color:#666">.</span>piper<span style="color:#666">@</span>iana<span style="color:#666">.</span>org<span style="color:#666">:</span> A valid email </span></span><span style="display:flex;"><span>first<span style="color:#666">.</span>last<span style="color:#666">@</span>example<span style="color:#666">.</span><span style="color:#666">123</span><span style="color:#666">:</span><span style="color:#008000;font-weight:bold">Not</span> a valid email </span></span></code></code>
Validating emails in PHP using the
FILTER_VALIDATE_EMAIL,
FILTER_SANITIZE_EMAIL and
filter_var() functions
We can remove all illegal characters from email address using appended FILTER_SANITIZE_EMAIL filter name id in first method. The filter name id is the second parameter in the
filter_var() function where the email address is the first parameter. This function returns the sanitized email. We can use this feature again to check the validity of the sanitized email address. For this we can follow the first approach using
FILTER_VALIDATE_EMAIL filter name id.
$email and store an email address that contains illegal characters. Store the email
ram(.mugu)@exa//mple.org as a string in a variable. Use the
filter_var() function on a variable and use the
FILTER_SANITIZE_EMAIL id as the second parameter. Store the function in the same
$email variable. Then, apply the
if-else statement like the first method. This time, use
FILTER_VALIDATE_EMAIL email as the filter name in the function. Likewise, the message is displayed.
filter_var() function filters these characters and sanitizes the provided email. The email address provided in the example contains illegal characters, such as
() and
//. The function first removes these characters from the email and then validates the email.
<code> <code class="language-php hljs" data-lang="php"><span style="display:flex;"><span><span style="color:#408080;font-style:italic">#php 7.x </span></span></span><span style="display:flex;"><span><span style="color:#666"></span>php </span></span><span style="display:flex;"><span><span style="color:#19177c">$email</span> <span style="color:#666">=</span> <span style="color:#ba2121">"ram(.mugu)@exa//mple.org"</span>; </span></span><span style="display:flex;"><span><span style="color:#19177c">$email</span> <span style="color:#666">=</span> filter_var(<span style="color:#19177c">$email</span>, FILTER_SANITIZE_EMAIL); </span></span><span style="display:flex;"><span><span style="color:#008000;font-weight:bold">if</span>(filter_var(<span style="color:#19177c">$email</span>, FILTER_VALIDATE_EMAIL)) { </span></span><span style="display:flex;"><span><span style="color:#008000;font-weight:bold">echo</span> <span style="color:#ba2121">"</span><span style="color:#b68;font-weight:bold">{</span><span style="color:#19177c">$email</span><span style="color:#b68;font-weight:bold">}</span><span style="color:#ba2121">: A valid email"</span><span style="color:#666">.</span><span style="color:#ba2121">"<br>"</span>; </span></span><span style="display:flex;"><span>} </span></span><span style="display:flex;"><span><span style="color:#008000;font-weight:bold">else</span>{ </span></span><span style="display:flex;"><span><span style="color:#008000;font-weight:bold">echo</span> <span style="color:#ba2121">"</span><span style="color:#b68;font-weight:bold">{</span><span style="color:#19177c">$email</span><span style="color:#b68;font-weight:bold">}</span><span style="color:#ba2121">:Not a valid email"</span><span style="color:#666">.</span><span style="color:#ba2121">"<br>"</span>; </span></span><span style="display:flex;"><span>} </span></span><span style="display:flex;"><span><span style="color:#bc7a00">?></span><span > </span></span></span></code></code>Output:
<code> <code class="language-php hljs" data-lang="php"><span style="display:flex;"><span>ram<span style="color:#666">.</span>mugu<span style="color:#666">@</span>example<span style="color:#666">.</span>org<span style="color:#666">:</span> A valid email </span></span></code></code>
使用 preg_match()
函数根据正则表达式验证电子邮件
我们可以使用 preg_match()
函数来验证 PHP 中的电子邮件地址。此方法使用正则表达式作为电子邮件的验证规则。我们可以自己创建正则表达式并定义有效电子邮件的规则。preg_match()
函数接受两个参数,其中第一个是正则表达式,第二个是要检查的电子邮件。我们可以使用三元运算符和函数一起检查电子邮件的有效性。
例如,创建两个变量 $email_first
和 $email_secon
,并在这些变量中存储两个电子邮件地址。首先存储有效的电子邮件 firstlast11@gmail.com
,然后存储无效的电子邮件 firstlast@11gmail,com
。编写一个带有一个参数的函数 validateEmail()
。命名参数 $email
。在函数内部,在 $regex
变量中编写一个正则表达式,如示例代码所示。然后编写一个三元运算符,其中要检查的条件是 preg_match()
函数。将 $regex
作为第一个参数,将 $email
作为第二个参数。当条件为真时打印电子邮件有效的消息,当条件为假时打印电子邮件无效的消息。回显整个三元表达式。在函数外,调用 validateEmail()
函数两次。在第一个函数调用中使用 $email_first
变量,在第二个函数调用中使用 $email_second
变量。
在下面的示例中,我们编写了一个正则表达式,用于创建验证电子邮件的规则。有效的电子邮件包含收件人姓名、@
符号、域和顶级域。上面创建的正则表达式接受收件人姓名作为字母数字值。字母表由大写字母和小写字母组成。它也接受一个句点。电子邮件必须有 @
符号。该域仅包含字母。然后电子邮件应该有一个句点。顶级域应该只由字母组成,并且长度应该是两个或三个。正则表达式是基于此规则创建的。第一封电子邮件是有效的,因为它满足所有规则,但第二封电子邮件无效。无效,因为域名中有数字,顶级域名前没有句号。
示例代码:
<code> <code class="language-php hljs" data-lang="php"><span style="display:flex;"><span><span style="color:#408080;font-style:italic"># php 7.x </span></span></span><span style="display:flex;"><span><span style="color:#666"></span>php </span></span><span style="display:flex;"><span><span style="color:#19177c">$email_first</span> <span style="color:#666">=</span> <span style="color:#ba2121">'firstlast11@gmail.com'</span>; </span></span><span style="display:flex;"><span><span style="color:#19177c">$email_second</span> <span style="color:#666">=</span><span style="color:#ba2121">'firstlast@11gmail,com'</span>; </span></span><span style="display:flex;"><span><span style="color:#008000;font-weight:bold">function</span> <span style="color:#00f">validateEmail</span>(<span style="color:#19177c">$email</span>) { </span></span><span style="display:flex;"><span><span style="color:#19177c">$regex</span> <span style="color:#666">=</span> <span style="color:#ba2121">"/^([a-zA-Z0-9\.]+@+[a-zA-Z]+(\.)+[a-zA-Z]{2,3})$/"</span>; </span></span><span style="display:flex;"><span><span style="color:#008000;font-weight:bold">echo</span> preg_match(<span style="color:#19177c">$regex</span>, <span style="color:#19177c">$email</span>) <span style="color:#666">?</span> <span style="color:#ba2121">"The email is valid"</span><span style="color:#666">.</span><span style="color:#ba2121">"<br>"</span> <span style="color:#666">:</span><span style="color:#ba2121">"The email is not valid"</span>; </span></span><span style="display:flex;"><span>} </span></span><span style="display:flex;"><span>validateEmail(<span style="color:#19177c">$email_first</span>); </span></span><span style="display:flex;"><span>validateEmail(<span style="color:#19177c">$email_second</span>); </span></span><span style="display:flex;"><span><span style="color:#bc7a00">?></span><span > </span></span></span></code></code>
输出:
<code> <code class="language-text hljs" data-lang="text"><span style="display:flex;"><span>The email is valid </span></span><span style="display:flex;"><span>The email is not valid </span></span></code></code>
The above is the detailed content of Verify email in PHP. For more information, please follow other related articles on the PHP Chinese website!
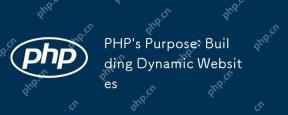
PHP is used to build dynamic websites, and its core functions include: 1. Generate dynamic content and generate web pages in real time by connecting with the database; 2. Process user interaction and form submissions, verify inputs and respond to operations; 3. Manage sessions and user authentication to provide a personalized experience; 4. Optimize performance and follow best practices to improve website efficiency and security.

PHP uses MySQLi and PDO extensions to interact in database operations and server-side logic processing, and processes server-side logic through functions such as session management. 1) Use MySQLi or PDO to connect to the database and execute SQL queries. 2) Handle HTTP requests and user status through session management and other functions. 3) Use transactions to ensure the atomicity of database operations. 4) Prevent SQL injection, use exception handling and closing connections for debugging. 5) Optimize performance through indexing and cache, write highly readable code and perform error handling.
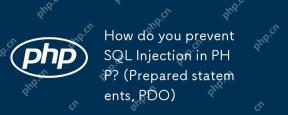
Using preprocessing statements and PDO in PHP can effectively prevent SQL injection attacks. 1) Use PDO to connect to the database and set the error mode. 2) Create preprocessing statements through the prepare method and pass data using placeholders and execute methods. 3) Process query results and ensure the security and performance of the code.
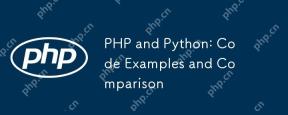
PHP and Python have their own advantages and disadvantages, and the choice depends on project needs and personal preferences. 1.PHP is suitable for rapid development and maintenance of large-scale web applications. 2. Python dominates the field of data science and machine learning.
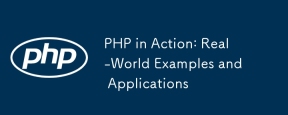
PHP is widely used in e-commerce, content management systems and API development. 1) E-commerce: used for shopping cart function and payment processing. 2) Content management system: used for dynamic content generation and user management. 3) API development: used for RESTful API development and API security. Through performance optimization and best practices, the efficiency and maintainability of PHP applications are improved.
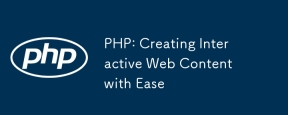
PHP makes it easy to create interactive web content. 1) Dynamically generate content by embedding HTML and display it in real time based on user input or database data. 2) Process form submission and generate dynamic output to ensure that htmlspecialchars is used to prevent XSS. 3) Use MySQL to create a user registration system, and use password_hash and preprocessing statements to enhance security. Mastering these techniques will improve the efficiency of web development.
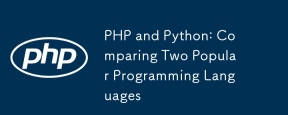
PHP and Python each have their own advantages, and choose according to project requirements. 1.PHP is suitable for web development, especially for rapid development and maintenance of websites. 2. Python is suitable for data science, machine learning and artificial intelligence, with concise syntax and suitable for beginners.
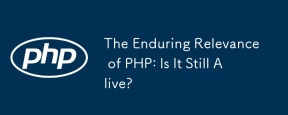
PHP is still dynamic and still occupies an important position in the field of modern programming. 1) PHP's simplicity and powerful community support make it widely used in web development; 2) Its flexibility and stability make it outstanding in handling web forms, database operations and file processing; 3) PHP is constantly evolving and optimizing, suitable for beginners and experienced developers.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use

Atom editor mac version download
The most popular open source editor
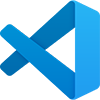
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

Zend Studio 13.0.1
Powerful PHP integrated development environment

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software