Analyze three practical application cases of Java factory pattern
Analysis of Three Practical Cases of Java Factory Pattern
Factory pattern is a commonly used design pattern, which can separate the creation and use of objects, making the program The scalability is better. In Java, there are three common practice cases of factory pattern: simple factory pattern, factory method pattern and abstract factory pattern. This article will analyze these three practical cases in detail and provide specific code examples.
- Simple Factory Pattern
The simple factory pattern is the most basic factory pattern, which creates objects through a factory class. In the simple factory pattern, the client only needs to interact with the factory class and does not need to interact directly with the specific product class.
The following takes creating a graphical object as an example to demonstrate the practice of the simple factory pattern:
// Create a graphical interface
public interface Shape {
void draw();
}
// Create a specific graphic class
public class Circle implements Shape {
@Override public void draw() { System.out.println("画一个圆形"); }
}
public class Rectangle implements Shape {
@Override public void draw() { System.out.println("画一个矩形"); }
}
// Create factory class
public class ShapeFactory {
public static Shape createShape(String type) { switch (type) { case "circle": return new Circle(); case "rectangle": return new Rectangle(); default: throw new IllegalArgumentException("不支持的图形类型"); } }
}
// Client usage example
public class Client {
public static void main(String[] args) { Shape circle = ShapeFactory.createShape("circle"); circle.draw(); Shape rectangle = ShapeFactory.createShape("rectangle"); rectangle.draw(); }
}
In the above code, a specific graphic object is created through the createShape method of the ShapeFactory factory class. The client only needs to call this method and pass in the corresponding parameters to obtain the corresponding graphic object and perform subsequent operations.
- Factory method pattern
The factory method pattern is a slightly more complex factory pattern. In the factory method pattern, each specific product class corresponds to a factory. kind. The client creates specific product objects by interacting with the corresponding factory class.
The following takes creating a car object as an example to demonstrate the practice of the factory method pattern:
// Create a car interface
public interface Car {
void drive();
}
// Create a specific car class
public class BenzCar implements Car {
@Override public void drive() { System.out.println("开奔驰车"); }
}
public class BmwCar implements Car {
@Override public void drive() { System.out.println("开宝马车"); }
}
// Create a factory interface
public interface CarFactory {
Car createCar();
}
// Create a specific factory class
public class BenzCarFactory implements CarFactory {
@Override public Car createCar() { return new BenzCar(); }
}
public class BmwCarFactory implements CarFactory {
@Override public Car createCar() { return new BmwCar(); }
}
// Client usage example
public class Client {
public static void main(String[] args) { CarFactory benzFactory = new BenzCarFactory(); Car benzCar = benzFactory.createCar(); benzCar.drive(); CarFactory bmwFactory = new BmwCarFactory(); Car bmwCar = bmwFactory.createCar(); bmwCar.drive(); }
}
In the above code, a specific car object is created through the CarFactory factory interface and its corresponding specific factory class. The client only needs to interact with the corresponding factory class to obtain the corresponding car object and perform subsequent operations.
- Abstract Factory Pattern
The abstract factory pattern is the most complex factory pattern. In the abstract factory pattern, each specific factory class can create multiple different types. product object. The client creates a series of related product objects by interacting with the factory class.
The following takes the creation of a mobile phone object as an example to demonstrate the practice of the abstract factory pattern:
// Create a mobile phone interface
public interface Phone {
void call();
}
// Create a specific mobile phone class
public class ApplePhone implements Phone {
@Override public void call() { System.out.println("使用苹果手机打电话"); }
}
public class HuaweiPhone implements Phone {
@Override public void call() { System.out.println("使用华为手机打电话"); }
}
// Create a factory interface
public interface PhoneFactory {
Phone createPhone();
}
// Create a specific factory class
public class ApplePhoneFactory implements PhoneFactory {
@Override public Phone createPhone() { return new ApplePhone(); }
}
public class HuaweiPhoneFactory implements PhoneFactory {
@Override public Phone createPhone() { return new HuaweiPhone(); }
}
// Client usage example
public class Client {
public static void main(String[] args) { PhoneFactory appleFactory = new ApplePhoneFactory(); Phone applePhone = appleFactory.createPhone(); applePhone.call(); PhoneFactory huaweiFactory = new HuaweiPhoneFactory(); Phone huaweiPhone = huaweiFactory.createPhone(); huaweiPhone.call(); }
}
In the above code, a specific mobile phone object is created through the PhoneFactory factory interface and its corresponding specific factory class. The client only needs to interact with the corresponding factory class to obtain the corresponding mobile phone object and perform subsequent operations.
Summary:
The above introduces three practical cases of the factory pattern: simple factory pattern, factory method pattern and abstract factory pattern. These three modes can effectively separate the creation and use of objects and improve the scalability of the program. In actual development, you can choose the appropriate factory mode to use according to specific needs.
The above is the detailed content of Analyze three practical application cases of Java factory pattern. For more information, please follow other related articles on the PHP Chinese website!
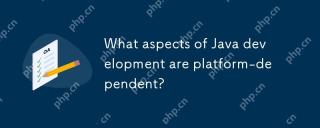
Javadevelopmentisnotentirelyplatform-independentduetoseveralfactors.1)JVMvariationsaffectperformanceandbehavioracrossdifferentOS.2)NativelibrariesviaJNIintroduceplatform-specificissues.3)Filepathsandsystempropertiesdifferbetweenplatforms.4)GUIapplica
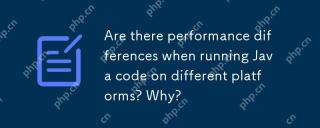
Java code will have performance differences when running on different platforms. 1) The implementation and optimization strategies of JVM are different, such as OracleJDK and OpenJDK. 2) The characteristics of the operating system, such as memory management and thread scheduling, will also affect performance. 3) Performance can be improved by selecting the appropriate JVM, adjusting JVM parameters and code optimization.
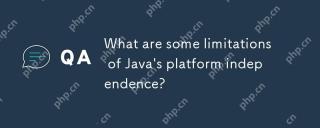
Java'splatformindependencehaslimitationsincludingperformanceoverhead,versioncompatibilityissues,challengeswithnativelibraryintegration,platform-specificfeatures,andJVMinstallation/maintenance.Thesefactorscomplicatethe"writeonce,runanywhere"
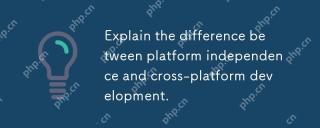
Platformindependenceallowsprogramstorunonanyplatformwithoutmodification,whilecross-platformdevelopmentrequiressomeplatform-specificadjustments.Platformindependence,exemplifiedbyJava,enablesuniversalexecutionbutmaycompromiseperformance.Cross-platformd
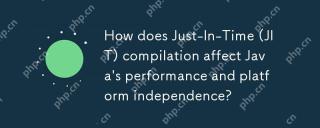
JITcompilationinJavaenhancesperformancewhilemaintainingplatformindependence.1)Itdynamicallytranslatesbytecodeintonativemachinecodeatruntime,optimizingfrequentlyusedcode.2)TheJVMremainsplatform-independent,allowingthesameJavaapplicationtorunondifferen
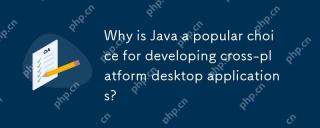
Javaispopularforcross-platformdesktopapplicationsduetoits"WriteOnce,RunAnywhere"philosophy.1)ItusesbytecodethatrunsonanyJVM-equippedplatform.2)LibrarieslikeSwingandJavaFXhelpcreatenative-lookingUIs.3)Itsextensivestandardlibrarysupportscompr
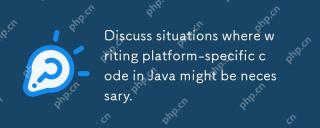
Reasons for writing platform-specific code in Java include access to specific operating system features, interacting with specific hardware, and optimizing performance. 1) Use JNA or JNI to access the Windows registry; 2) Interact with Linux-specific hardware drivers through JNI; 3) Use Metal to optimize gaming performance on macOS through JNI. Nevertheless, writing platform-specific code can affect the portability of the code, increase complexity, and potentially pose performance overhead and security risks.
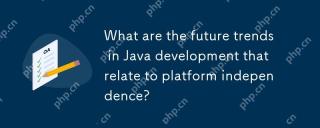
Java will further enhance platform independence through cloud-native applications, multi-platform deployment and cross-language interoperability. 1) Cloud native applications will use GraalVM and Quarkus to increase startup speed. 2) Java will be extended to embedded devices, mobile devices and quantum computers. 3) Through GraalVM, Java will seamlessly integrate with languages such as Python and JavaScript to enhance cross-language interoperability.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
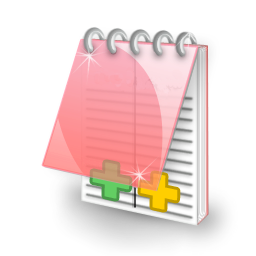
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
