


Are there performance differences when running Java code on different platforms? Why?
Java code will have performance differences when running on different platforms. 1) The implementation and optimization strategies of JVM are different, such as Oracle JDK and OpenJDK. 2) The characteristics of the operating system, such as memory management and thread scheduling, will also affect performance. 3) You can improve performance by selecting the appropriate JVM, adjusting JVM parameters and code optimization.
introduction
As we explore the world of Java programming, a question that is often mentioned but easily overlooked is: Will there be performance differences in running Java code on different platforms? This is not only a technical issue, but also a key factor that every Java developer needs to consider when developing cross-platform. Today, we will explore this issue in depth and share some practical experience to help you better understand and optimize the performance of your Java code.
In this article, you will learn about the cross-platform features of Java, learn how to evaluate and optimize performance on different operating systems, and master some practical tips to improve the overall efficiency of your Java application.
Review of basic knowledge
A core advantage of Java is its "write once, run everywhere" feature. This is thanks to the Java Virtual Machine (JVM), which runs on different operating systems and is responsible for converting Java bytecode into local machine code. Understanding the working mechanism of JVM is the basis for understanding performance differences.
The implementation of JVMs is different, such as Oracle JDK, OpenJDK, etc., and their optimization strategies on different platforms are also different. In addition, the characteristics of the operating system itself, such as memory management, thread scheduling, etc., will also affect the performance of Java applications.
Core concept or function analysis
The definition and role of Java cross-platform performance
Java cross-platform performance refers to the performance differences shown by the same piece of Java code when it runs on different operating systems. This difference may be reflected in the execution speed of the program, memory usage, and resource utilization.
The cross-platform feature of Java allows us to write code once and then run on multiple platforms such as Windows, Linux, macOS, etc., which greatly simplifies the development process. However, the existence of performance differences reminds us that when cross-platform development, specialized optimization of the code is needed.
How it works
When you run a Java program, the JVM will first load and verify the bytecode, and then perform JIT (instant compilation) optimization to convert the bytecode into local machine code. In this process, the JVM will be optimized according to the characteristics of the current platform. For example, it may utilize more efficient thread scheduling algorithms on Linux, and file I/O operations may be optimized on Windows.
JVM implementations on different platforms may adopt different garbage collection algorithms, which will also affect performance. For example, the G1 garbage collector is more efficient on Linux than on Windows.
Example of usage
Basic usage
Let's look at a simple Java program that shows the basic performance differences on different platforms:
public class PerformanceTest { public static void main(String[] args) { long startTime = System.currentTimeMillis(); for (int i = 0; i < 1000000; i ) { // A simple operation int result = i * i; } long endTime = System.currentTimeMillis(); System.out.println("Execution time: " (endTime - startTime) " ms"); } }
This code may have different execution times when it runs on Windows, Linux, and macOS. This is because the JVM optimization strategies are different on different platforms.
Advanced Usage
In practical applications, we may encounter more complex scenarios, such as multi-threaded concurrent processing. Let's look at a multi-threaded example showing the performance differences on different platforms:
public class MultiThreadPerformanceTest { public static void main(String[] args) throws InterruptedException { int numThreads = 4; Thread[] threads = new Thread[numThreads]; for (int i = 0; i < numThreads; i ) { threads[i] = new Thread(() -> { for (int j = 0; j < 10000000; j ) { int result = j * j; } }); threads[i].start(); } for (Thread thread : threads) { thread.join(); } long endTime = System.currentTimeMillis(); System.out.println("Execution time: " (endTime - startTime) " ms"); } }
In this example, thread scheduling strategies on different platforms affect performance. Thread scheduling on Linux is usually more efficient than on Windows, which may result in shorter execution time on Linux.
Common Errors and Debugging Tips
In cross-platform development, a common mistake is to assume that performance is the same across all platforms. In fact, you need to perform performance testing on each target platform and optimize based on the test results.
One of the debugging tips is to use Java's own performance analysis tools, such as JVisualVM or JProfiler. These tools can help you identify performance bottlenecks and provide detailed performance reports.
Performance optimization and best practices
In practical applications, many aspects need to be considered for optimizing the performance of Java code:
- Choose the right JVM : Different JVM implementations may show different performance on different platforms. For example, OpenJDK usually performs better on Linux than on Windows.
- Adjust JVM parameters : By adjusting JVM garbage collection policy, heap size and other parameters, performance can be significantly improved. For example, using a G1 garbage collector on Linux is often more efficient than using CMS.
- Code optimization : When writing Java code, be careful to avoid unnecessary object creation, use efficient data structures and algorithms, etc. For example, using StringBuilder instead of String splicing can improve performance.
One of the best practices in cross-platform development is continuous performance monitoring and optimization. By running performance tests on different platforms regularly and adjusting code and JVM parameters based on test results, you can ensure that your Java application achieves the best performance on all target platforms.
In short, understanding and optimizing the performance differences of Java code on different platforms is an indispensable skill for every Java developer. Through the discussion of this article and sharing of practical experience, I hope you can easily write efficient and reliable Java code in cross-platform development.
The above is the detailed content of Are there performance differences when running Java code on different platforms? Why?. For more information, please follow other related articles on the PHP Chinese website!
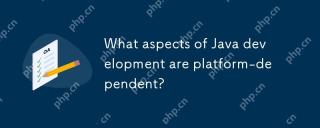
Javadevelopmentisnotentirelyplatform-independentduetoseveralfactors.1)JVMvariationsaffectperformanceandbehavioracrossdifferentOS.2)NativelibrariesviaJNIintroduceplatform-specificissues.3)Filepathsandsystempropertiesdifferbetweenplatforms.4)GUIapplica
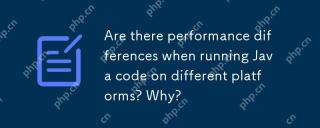
Java code will have performance differences when running on different platforms. 1) The implementation and optimization strategies of JVM are different, such as OracleJDK and OpenJDK. 2) The characteristics of the operating system, such as memory management and thread scheduling, will also affect performance. 3) Performance can be improved by selecting the appropriate JVM, adjusting JVM parameters and code optimization.
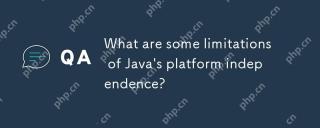
Java'splatformindependencehaslimitationsincludingperformanceoverhead,versioncompatibilityissues,challengeswithnativelibraryintegration,platform-specificfeatures,andJVMinstallation/maintenance.Thesefactorscomplicatethe"writeonce,runanywhere"
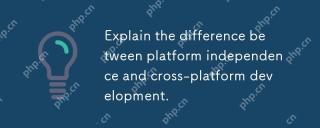
Platformindependenceallowsprogramstorunonanyplatformwithoutmodification,whilecross-platformdevelopmentrequiressomeplatform-specificadjustments.Platformindependence,exemplifiedbyJava,enablesuniversalexecutionbutmaycompromiseperformance.Cross-platformd
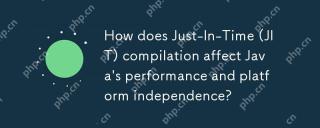
JITcompilationinJavaenhancesperformancewhilemaintainingplatformindependence.1)Itdynamicallytranslatesbytecodeintonativemachinecodeatruntime,optimizingfrequentlyusedcode.2)TheJVMremainsplatform-independent,allowingthesameJavaapplicationtorunondifferen
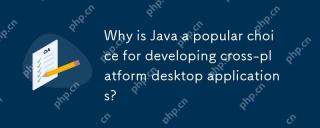
Javaispopularforcross-platformdesktopapplicationsduetoits"WriteOnce,RunAnywhere"philosophy.1)ItusesbytecodethatrunsonanyJVM-equippedplatform.2)LibrarieslikeSwingandJavaFXhelpcreatenative-lookingUIs.3)Itsextensivestandardlibrarysupportscompr
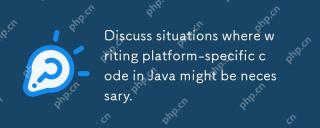
Reasons for writing platform-specific code in Java include access to specific operating system features, interacting with specific hardware, and optimizing performance. 1) Use JNA or JNI to access the Windows registry; 2) Interact with Linux-specific hardware drivers through JNI; 3) Use Metal to optimize gaming performance on macOS through JNI. Nevertheless, writing platform-specific code can affect the portability of the code, increase complexity, and potentially pose performance overhead and security risks.
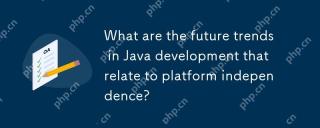
Java will further enhance platform independence through cloud-native applications, multi-platform deployment and cross-language interoperability. 1) Cloud native applications will use GraalVM and Quarkus to increase startup speed. 2) Java will be extended to embedded devices, mobile devices and quantum computers. 3) Through GraalVM, Java will seamlessly integrate with languages such as Python and JavaScript to enhance cross-language interoperability.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
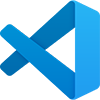
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
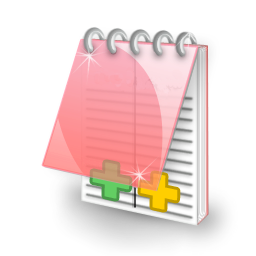
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
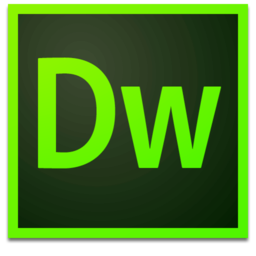
Dreamweaver Mac version
Visual web development tools
