


Quick Start with Django Projects: Master the project creation commands and quickly build your own applications
Quick Start with Django Project: Master the project creation commands and quickly build your own application. Specific code examples are required
Django is an open source, efficient Python Web framework. It can help developers quickly build stable and reliable web applications. In this article, we will learn how to use Django to create projects and build our own applications.
1. Install Django
Before we begin, we need to install Django first. You can use the following command to install Django in the terminal:
pip install django
2. Create a Django project
After installing Django, we can use the following command to create a new project:
django-admin startproject myproject
This will create a Django project folder named "myproject". Enter the folder:
cd myproject
3. Create a Django application
Next, we will create an application in the project. A Django project can contain multiple applications, each application is responsible for completing different functions.
Create an app using the following command:
python manage.py startapp myapp
This will create an app folder named "myapp".
4. Configure the application
After creating the application, you need to add it to the project configuration. Open the settings.py
file and find the INSTALLED_APPS
list. Add the application we just created to the list:
INSTALLED_APPS = [ ... 'myapp', ... ]
5. Write the view function
In Django, the view function is responsible for processing user requests and returning corresponding content.
Go to the myapp
folder, create a file named views.py
, and add the following code:
from django.http import HttpResponse def hello(request): return HttpResponse("Hello, Django!")
6. Configure URL Mapping
To let Django know how to map user requests to our view functions, we need to configure URL mapping.
Under the myapp
folder, create a file named urls.py
and add the following code:
from django.urls import path from . import views urlpatterns = [ path('hello/', views.hello, name='hello'), ]
This configuration means When a user accesses the /hello/
path, the views.hello
function will be called to process the request.
7. Configure project URL mapping
In the project folder, there is a file named urls.py
. This file is used to configure the URL mapping of the entire project.
Edit the urls.py
file and add the following code to the urlpatterns
list:
from django.contrib import admin from django.urls import path, include urlpatterns = [ path('admin/', admin.site.urls), path('myapp/', include('myapp.urls')), ]
In this configuration, we add the URL of the project Requests starting with /myapp/
are handled by the URL configuration of the myapp
application.
8. Run the project
Now that we have completed the basic configuration of the project, we can try to run the project. Execute the following command on the command line:
python manage.py runserver
This command will start Django’s development server. Visit http://127.0.0.1:8000/myapp/hello/
in the browser, and you will see the "Hello, Django!" message.
Through the above steps, we successfully created a Django project and built a simple application. You can add more features and view functions to your application according to your needs.
Summary
In this article, we learned how to use Django to create a project and build a simple application. By mastering the project creation commands and the process of building applications, we can quickly start developing our own web applications. I hope this article will help you get started with Django!
The above is the detailed content of Quick Start with Django Projects: Master the project creation commands and quickly build your own applications. For more information, please follow other related articles on the PHP Chinese website!
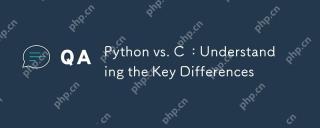
Python and C each have their own advantages, and the choice should be based on project requirements. 1) Python is suitable for rapid development and data processing due to its concise syntax and dynamic typing. 2)C is suitable for high performance and system programming due to its static typing and manual memory management.
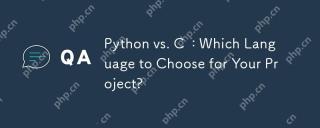
Choosing Python or C depends on project requirements: 1) If you need rapid development, data processing and prototype design, choose Python; 2) If you need high performance, low latency and close hardware control, choose C.
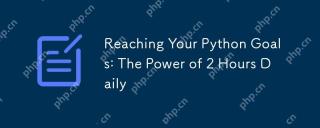
By investing 2 hours of Python learning every day, you can effectively improve your programming skills. 1. Learn new knowledge: read documents or watch tutorials. 2. Practice: Write code and complete exercises. 3. Review: Consolidate the content you have learned. 4. Project practice: Apply what you have learned in actual projects. Such a structured learning plan can help you systematically master Python and achieve career goals.
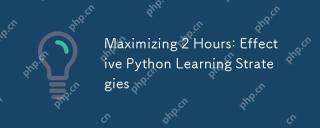
Methods to learn Python efficiently within two hours include: 1. Review the basic knowledge and ensure that you are familiar with Python installation and basic syntax; 2. Understand the core concepts of Python, such as variables, lists, functions, etc.; 3. Master basic and advanced usage by using examples; 4. Learn common errors and debugging techniques; 5. Apply performance optimization and best practices, such as using list comprehensions and following the PEP8 style guide.

Python is suitable for beginners and data science, and C is suitable for system programming and game development. 1. Python is simple and easy to use, suitable for data science and web development. 2.C provides high performance and control, suitable for game development and system programming. The choice should be based on project needs and personal interests.
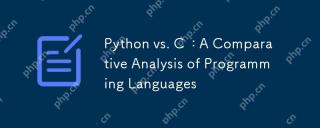
Python is more suitable for data science and rapid development, while C is more suitable for high performance and system programming. 1. Python syntax is concise and easy to learn, suitable for data processing and scientific computing. 2.C has complex syntax but excellent performance and is often used in game development and system programming.
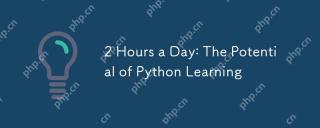
It is feasible to invest two hours a day to learn Python. 1. Learn new knowledge: Learn new concepts in one hour, such as lists and dictionaries. 2. Practice and exercises: Use one hour to perform programming exercises, such as writing small programs. Through reasonable planning and perseverance, you can master the core concepts of Python in a short time.
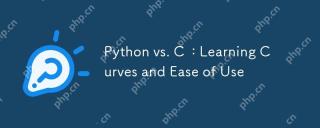
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
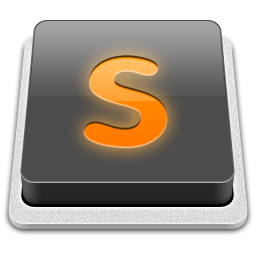
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

Atom editor mac version download
The most popular open source editor
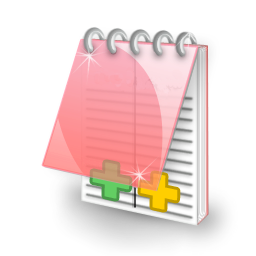
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.