


Django installation tutorial: Build an efficient Web application from scratch, specific code examples are required
Introduction:
Django is an efficient Web written in Python Application development framework. It provides a way to quickly build stable, secure and scalable web applications. This article will introduce in detail how to install and configure Django from scratch, and provide specific code examples to help beginners get started smoothly.
1. Install Python and pip
Django is developed based on Python, so you need to install Python on your computer first. You can download the latest version of Python from the official website (https://www.python.org/downloads/) and follow the installation wizard to complete the installation.
After installing Python, you need to install pip, which is Python's package management tool. Enter the following command on the command line:
$ python -m ensurepip --upgrade
$ python -m pip install --upgrade pip
2. Install Django
After pip is installed, we can use it to install Django. Enter the following command on the command line:
$ pip install django
3. Create a Django project
After installing Django, we can start creating a new Django project. Enter the following command at the command line:
$ django-admin startproject myproject
This will create a folder named "myproject" in the current directory and generate the basic structure of the Django project in it.
4. Run the Django development server
Enter the project folder "myproject" and enter the following command in the command line:
$ python manage.py runserver
This will start the Django development server and listen locally by default 8000 port. Enter "http://localhost:8000" in your browser and you will see Django's default welcome page.
5. Create a Django application
In addition to the structure of the project itself, we can also create applications in the Django project. Enter the following command at the command line:
$ python manage.py startapp myapp
This will create an application named "myapp" in the project and generate the basic structure of the application within it.
6. Create a model
Model is a class used in Django to define the database structure. In the "models.py" file of the "myapp" application, we can define our models. The following is the code for an example model:
from django.db import models class Book(models.Model): title = models.CharField(max_length=100) author = models.CharField(max_length=100) publication_date = models.DateField() def __str__(self): return self.title
This model defines a class named "Book", which has three attributes: title, author, and publication_date. We can also specify what is displayed when printing the object in the console by overriding the __str__() method.
7. Database migration
After defining the model, we need to tell Django that our database structure has changed. Enter the following command on the command line:
$ python manage.py makemigrations
This will generate a series of database migration files to record database changes. Then enter the following command:
$ python manage.py migrate
This will perform the actual change operation of the database based on the migration file.
8. Create views and URLs
Views are functions used in Django to process user requests. In the "myapp" application, we can define our views in the "views.py" file. The following is the code for a sample view:
from django.shortcuts import render from django.http import HttpResponse def index(request): return HttpResponse("Hello, world!")
This view function receives a request object and returns a response object containing the text "Hello, world!"
In order to make our view accessible, we also need to add the corresponding URL configuration in the "urls.py" file of the "myproject" project. Here is an example URL configuration code:
from django.urls import path from myapp.views import index urlpatterns = [ path('', index, name='index'), ]
This will map the empty path to the "index" view function we defined earlier.
9. Run the Django development server
After completing the above steps, we can run the Django development server again to view our application. Enter the following command in the command line:
$ python manage.py runserver
Then enter "http://localhost:8000" in the browser, you will see the "Hello, world!" text we defined earlier.
Conclusion:
This article introduces the installation and configuration process of Django and provides some specific code examples. I hope that through this tutorial, beginners can successfully build their own Django project and understand the basic usage of Django. Of course, in addition to what is mentioned in this article, Django has many other powerful functions and tools that require further learning and practice.
The above is the detailed content of Learn how to build efficient web applications in Django from scratch. For more information, please follow other related articles on the PHP Chinese website!
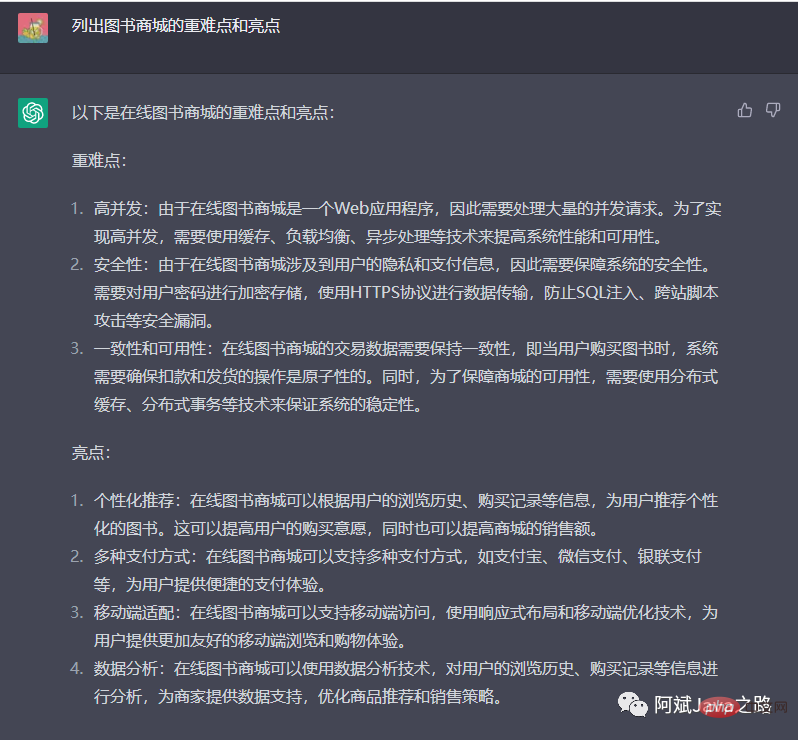
已经火了很久了,身边的同事也用它来进行一些调研,资源检索,工作汇报等方面都有很大的的效率提升。很多人问ChatGPT会不会取代程序员?我的回答是:不会!ChatGPT并不是我们的敌人,相反的是,它是我们的好帮手。未来人和人的竞争,可能就会从原先的我懂得更多,我实操经验更丰富,变成了我比你更会用工具,我比你更懂得提问,我比你更会发挥机器人的最大特性,所以,为了不掉队,你还不准备体验下ChatGPT吗?快速体验面试官经常会问你的项目有啥重难点?很多人不会回答,直接看看ChatGPT怎么说,真的太牛了
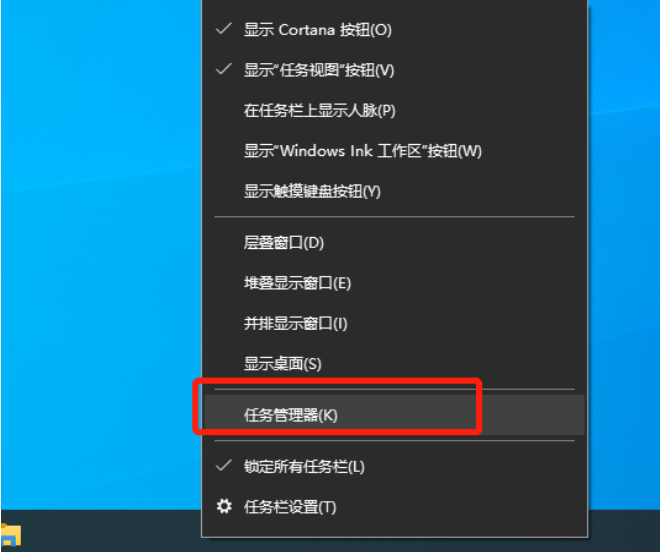
电脑上广告弹窗太多了怎么办,有的小伙伴不想重装系统,下面就和大家讲讲关闭win10广告的方法吧,大家可以借鉴一下。1、右键点击电脑桌面下方任务栏,在弹出的菜单中选择并打开“任务管理器”。2、右键点击需要关闭的启动项,选择“禁用”。对应软件的开机启动项就关闭成功了。弹窗拦截设置1、打开毒霸,在首页点击左下方的“弹窗拦截”。2、点击“扫描”,对电脑进行全面扫描找出带有弹窗的软件。3、勾选需要拦截的软件,然后点击“一键拦截”。4、一键拦截后,对应的软件弹窗问题就已被拦截了。综上所述,如果大家电脑win
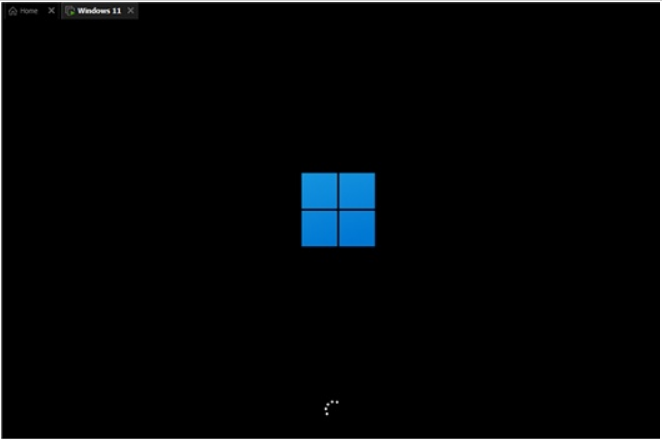
微软近日透露了将推出win11系统,很多用户都在期待新系统呢。网上已经有泄露关于win11的镜像安装系统。大家不知道如何安装的话,可以使用U盘来进行安装。小编现在就给大家带来了win11的U盘安装教程。1、首先准备一个8G以上大小的u盘,将它制作成系统盘。2、接着下载win11系统镜像文件,将它放入u盘中,大家可以直接点击右侧的链接进行下载。3、下载完成后装载该iso文件。4、装载完成之后会进入新的文件夹,在其中找到并运行win11的安装程序。5、在列表中选择“win11”然后点击“下一步”。6
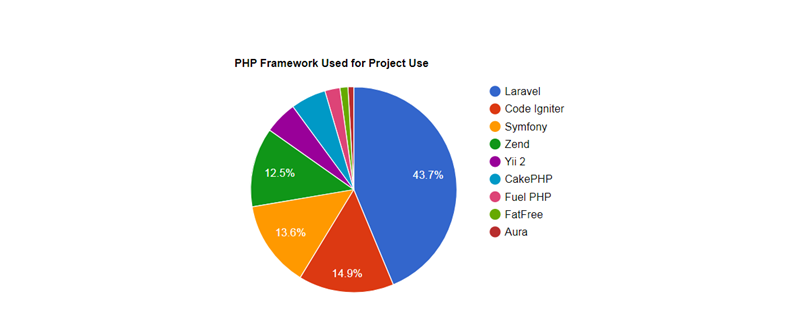
如果想快速进行php web开发,选择一个好用的php开发框架至关重要,一个好的php开发框架可以让开发工作变得更加快捷、安全和有效。那2023年最流行的php开发框架有哪些呢?这些php开发框架排名如何?
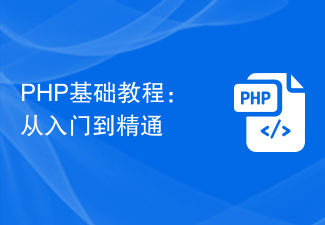
PHP是一种广泛使用的开源服务器端脚本语言,它可以处理Web开发中所有的任务。PHP在网页开发中的应用广泛,尤其是在动态数据处理上表现优异,因此被众多开发者喜爱和使用。在本篇文章中,我们将一步步地讲解PHP基础知识,帮助初学者从入门到精通。一、基本语法PHP是一种解释性语言,其代码类似于HTML、CSS和JavaScript。每个PHP语句都以分号;结束,注
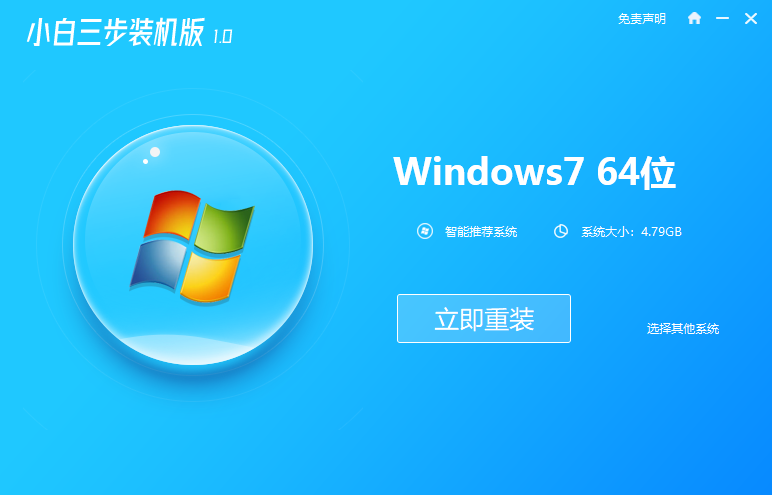
xp系统曾经是使用最多的系统,不过随着硬件的不断升级,xp系统已经不能发挥硬件的性能,所以很多朋友就想升级win7系统,下面就和大家分享一下老电脑升级win7系统的方法吧。1、在小白一键重装系统官网中下载小白三步装机版软件并打开,软件会自动帮助我们匹配合适的系统,然后点击立即重装。2、接下来软件就会帮助我们直接下载系统镜像,只需要耐心等候即可。3、下载完成后软件会帮助我们直接进行在线重装Windows系统,请根据提示操作。4、安装完成后会提示我们重启,选择立即重启。5、重启后在PE菜单中选择Xi
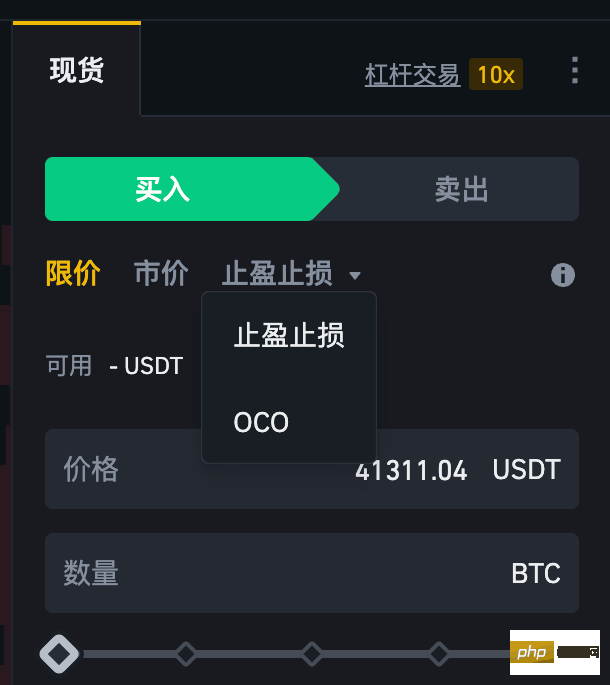
二选一订单(OneCancelstheOther,简称OCO)可让您同时下达两个订单。它结合了限价单和限价止损单,但只能执行其中一个。换句话说,只要其中的限价单被部分或全部成交、止盈止损单被触发,另一个订单将自动取消。请注意,取消其中一个订单也会同时取消另一个订单。在币安交易平台进行交易时,您可以将二选一订单作为交易自动化的基本形式。这个功能可让您选择同时下达两个限价单,从而有助于止盈和最大程度减少潜在损失。如何使用二选一订单?登录您的币安帐户之后,请前往基本交易界面,找到下图所示的交易区域。点
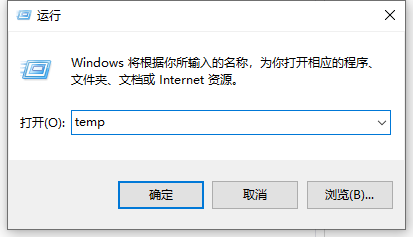
在win10的系统盘中,很多网友会看到一个temp文件夹,里面占用的内存非常大,占用了c盘很多空间。有网友想删除temp文件夹,但是不知道能不能删,win10如何删除temp文件夹。下面小编就教下大家win10删除temp文件夹的方法。首先,Temp是指系统临时文件夹。而很多收藏夹,浏览网页的临时文件都放在这里,这是根据你操作的过程临时保存下来的。如有需要,可以手动删除的。如何删除temp文件夹?具体步骤如下:方法一:1、按下【Win+R】组合键打开运行,在运行框中输入temp,点击确定;2、此


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
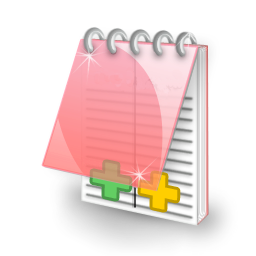
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SublimeText3 Chinese version
Chinese version, very easy to use

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
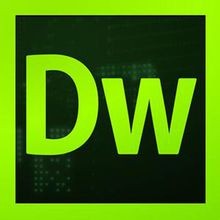
Dreamweaver CS6
Visual web development tools
