


Why are arrays generally more memory-efficient than lists for storing numerical data?
Arrays are generally more memory-efficient than lists for storing numerical data due to their fixed-size nature and direct memory access. 1) Arrays store elements in a contiguous block, reducing overhead from pointers or metadata. 2) Lists, often implemented as dynamic arrays or linked structures, may waste memory due to extra allocation for growth or pointers. 3) Numpy arrays in Python demonstrate lower memory usage than lists for numerical data. 4) However, arrays' fixed size can be less flexible than lists, impacting their efficiency when frequent resizing is needed.
Arrays are generally more memory-efficient than lists for storing numerical data due to their fixed-size nature and direct memory access. Let's dive deeper into this and explore the nuances of memory efficiency in the context of arrays and lists.
When we talk about arrays, we're essentially dealing with a contiguous block of memory where each element is stored one after the other. This contiguous storage allows for efficient memory usage because there's no overhead for pointers or metadata that you'd typically find in dynamic data structures like lists. Each element in an array is directly accessible via an index, which translates to quick memory access and efficient cache usage.
Now, let's contrast this with lists. In many programming languages, lists are implemented as dynamic arrays or linked structures. In the case of dynamic arrays, while they offer similar memory efficiency to static arrays when full, they often need to allocate extra space to accommodate potential growth, which can lead to memory wastage. Linked list implementations, on the other hand, store each element along with a pointer to the next element, which introduces additional memory overhead.
Let's illustrate this with a Python example, where we'll compare the memory usage of an array (using numpy
) and a list:
import numpy as np import sys # Creating an array of 1000 integers array = np.array([i for i in range(1000)], dtype=np.int32) print(f"Memory used by numpy array: {sys.getsizeof(array)} bytes") # Creating a list of 1000 integers list_data = [i for i in range(1000)] print(f"Memory used by list: {sys.getsizeof(list_data)} bytes")
Running this code, you'll likely see that the numpy array uses less memory than the list. This is because numpy arrays are optimized for numerical data and store elements in a compact, contiguous block, whereas the list has additional overhead due to its dynamic nature.
However, it's important to consider the trade-offs. Arrays, with their fixed size, can be less flexible than lists. If you need to frequently add or remove elements, the overhead of resizing an array can outweigh its memory efficiency. Lists, on the other hand, offer more flexibility at the cost of memory efficiency.
From a performance perspective, arrays can offer better cache locality due to their contiguous memory allocation. This can lead to faster data access and processing, especially in numerical computations or when dealing with large datasets.
In my experience, I've found that the choice between arrays and lists often depends on the specific requirements of the project. For applications involving heavy numerical computations, like scientific computing or data analysis, arrays (e.g., numpy arrays in Python) are often the go-to choice due to their memory efficiency and performance benefits. However, for more general-purpose programming where flexibility is key, lists might be more appropriate despite their higher memory usage.
To wrap up, while arrays are generally more memory-efficient for storing numerical data, the decision between arrays and lists should consider not just memory efficiency but also factors like performance requirements, data manipulation needs, and the overall design of your application. Always profile your code and understand the specific demands of your use case to make an informed choice.
The above is the detailed content of Why are arrays generally more memory-efficient than lists for storing numerical data?. For more information, please follow other related articles on the PHP Chinese website!
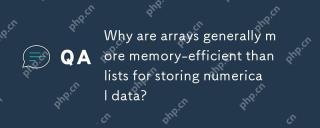
Arraysaregenerallymorememory-efficientthanlistsforstoringnumericaldataduetotheirfixed-sizenatureanddirectmemoryaccess.1)Arraysstoreelementsinacontiguousblock,reducingoverheadfrompointersormetadata.2)Lists,oftenimplementedasdynamicarraysorlinkedstruct
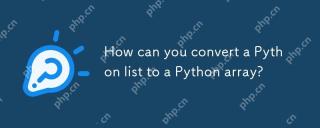
ToconvertaPythonlisttoanarray,usethearraymodule:1)Importthearraymodule,2)Createalist,3)Usearray(typecode,list)toconvertit,specifyingthetypecodelike'i'forintegers.Thisconversionoptimizesmemoryusageforhomogeneousdata,enhancingperformanceinnumericalcomp
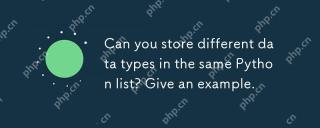
Python lists can store different types of data. The example list contains integers, strings, floating point numbers, booleans, nested lists, and dictionaries. List flexibility is valuable in data processing and prototyping, but it needs to be used with caution to ensure the readability and maintainability of the code.
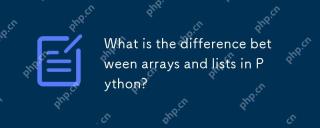
Pythondoesnothavebuilt-inarrays;usethearraymoduleformemory-efficienthomogeneousdatastorage,whilelistsareversatileformixeddatatypes.Arraysareefficientforlargedatasetsofthesametype,whereaslistsofferflexibilityandareeasiertouseformixedorsmallerdatasets.

ThemostcommonlyusedmoduleforcreatingarraysinPythonisnumpy.1)Numpyprovidesefficienttoolsforarrayoperations,idealfornumericaldata.2)Arrayscanbecreatedusingnp.array()for1Dand2Dstructures.3)Numpyexcelsinelement-wiseoperationsandcomplexcalculationslikemea

ToappendelementstoaPythonlist,usetheappend()methodforsingleelements,extend()formultipleelements,andinsert()forspecificpositions.1)Useappend()foraddingoneelementattheend.2)Useextend()toaddmultipleelementsefficiently.3)Useinsert()toaddanelementataspeci
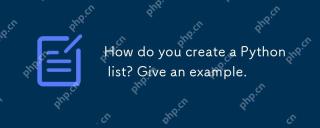
TocreateaPythonlist,usesquarebrackets[]andseparateitemswithcommas.1)Listsaredynamicandcanholdmixeddatatypes.2)Useappend(),remove(),andslicingformanipulation.3)Listcomprehensionsareefficientforcreatinglists.4)Becautiouswithlistreferences;usecopy()orsl
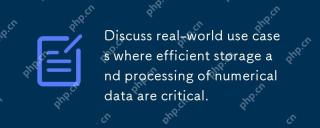
In the fields of finance, scientific research, medical care and AI, it is crucial to efficiently store and process numerical data. 1) In finance, using memory mapped files and NumPy libraries can significantly improve data processing speed. 2) In the field of scientific research, HDF5 files are optimized for data storage and retrieval. 3) In medical care, database optimization technologies such as indexing and partitioning improve data query performance. 4) In AI, data sharding and distributed training accelerate model training. System performance and scalability can be significantly improved by choosing the right tools and technologies and weighing trade-offs between storage and processing speeds.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
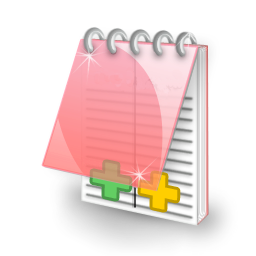
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
