PHP SPL Data Structures: The Ultimate Guide for Developers
php editor Xigua brings you "PHP SPL Data Structure: The Ultimate Guide for Developers". This guide will introduce in detail the usage and characteristics of various data structures in the PHP Standard Library (SPL) to help developers more Understand and apply these powerful tools well to improve code efficiency and quality. Whether you are a beginner or an experienced developer, this guide will provide you with comprehensive and clear guidance to help you master the essence of SPL data structure.
SPL The Array class (SplArray
) is an extended PHP array implementation that provides additional functionality such as iterator support, key comparators, and Various array manipulation methods (such as merge
, reduce
, and shuffle
).
Example:
$arr = new SplArray(); $arr[] = 1; $arr[] = 2; $arr[] = 3; // 迭代数组 foreach ($arr as $item) { echo $item . php_EOL; }
SPL stack
The stack is a linear data structure that follows the last-in-first-out (LIFO) principle. The SPL stack class (SplStack
) provides a stack implementation that supports pushing (push
), popping (pop
) and taking the top of the stack ( peek
) operation.
Example:
$stack = new SplStack(); $stack->push(1); $stack->push(2); $stack->push(3); // 出栈元素 $top = $stack->pop(); echo "已出栈的元素:$top" . PHP_EOL;
SPL Queue
Queue is a linear data structure that follows the first-in-first-out (FIFO) principle. The SPL queue class (SplQueue
) provides a queue implementation that supports enqueuing (enqueue
), dequeuing (dequeue
) and taking the head of the queue ( front
) operation.
Example:
$queue = new SplQueue(); $queue->enqueue(1); $queue->enqueue(2); $queue->enqueue(3); // 出队元素 $front = $queue->dequeue(); echo "已出队的元素:$front" . PHP_EOL;
SPL Stack
A stack (also known as a minimum priority queue) is a data structure in which elements are sorted by priority , with the lowest priority element at the top of the stack. The SPL stack class (SplHeap
) provides a stack implementation that supports insertion, deletion and minimum element operations.
Example:
$heap = new SplHeap(); $heap->insert(10); $heap->insert(5); $heap->insert(15); // 取最小元素 $min = $heap->extract(); echo "最小元素:$min" . PHP_EOL;
SPL Hash Table
SPL hash table class (SplObjectStorage
) provides a hash table implementation based on key-value pairs. It allows storing objects of any type as values and using the objects themselves as keys.
Example:
$storage = new SplObjectStorage(); $obj1 = new MyClass(); $obj2 = new MyClass(); $storage->attach($obj1, "value1"); $storage->attach($obj2, "value2"); // 检索值 $value = $storage[$obj1]; echo "对象 $obj1 对应的值:$value" . PHP_EOL;
SPL ordered set
SPL OrderedSet class (SplTreeSet
) provides a tree-based collection implementation that supports insertion, deletion and search operations of elements. The elements in the collection are sorted in natural order, or can be sorted using a custom comparator.
Example:
$set = new SplTreeSet(); $set->insert(1); $set->insert(3); $set->insert(2); // 查找元素 if ($set->contains(2)) { echo "集合中包含元素 2" . PHP_EOL; }
SPL Doubly Linked List
SPL Two-way Linked List class (SplDoublyLinkedList
) provides a doubly linked list implementation that supports insertion, deletion and traversal operations. Elements in a linked list can be traversed forward or backward.
Example:
$list = new SplDoublyLinkedList(); $list->push(1); $list->push(2); $list->push(3); // 向后遍历链表 $prev = null; foreach ($list as $item) { echo $item . " "; // 保存当前节点的指针 $prev = $list->current(); // 移动到下一个节点 $list->next(); }
in conclusion
The SPL data structure provides PHP developers with a set of powerful and easy-to-use tools for organizing and manipulating data. By understanding and mastering these data structures, developers can improve the efficiency and maintainability of their code.
The above is the detailed content of PHP SPL Data Structures: The Ultimate Guide for Developers. For more information, please follow other related articles on the PHP Chinese website!
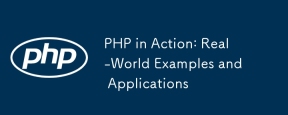
PHP is widely used in e-commerce, content management systems and API development. 1) E-commerce: used for shopping cart function and payment processing. 2) Content management system: used for dynamic content generation and user management. 3) API development: used for RESTful API development and API security. Through performance optimization and best practices, the efficiency and maintainability of PHP applications are improved.
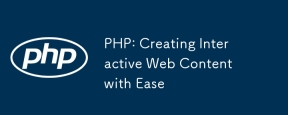
PHP makes it easy to create interactive web content. 1) Dynamically generate content by embedding HTML and display it in real time based on user input or database data. 2) Process form submission and generate dynamic output to ensure that htmlspecialchars is used to prevent XSS. 3) Use MySQL to create a user registration system, and use password_hash and preprocessing statements to enhance security. Mastering these techniques will improve the efficiency of web development.
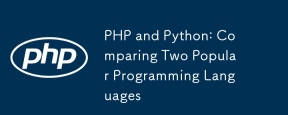
PHP and Python each have their own advantages, and choose according to project requirements. 1.PHP is suitable for web development, especially for rapid development and maintenance of websites. 2. Python is suitable for data science, machine learning and artificial intelligence, with concise syntax and suitable for beginners.
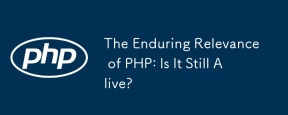
PHP is still dynamic and still occupies an important position in the field of modern programming. 1) PHP's simplicity and powerful community support make it widely used in web development; 2) Its flexibility and stability make it outstanding in handling web forms, database operations and file processing; 3) PHP is constantly evolving and optimizing, suitable for beginners and experienced developers.
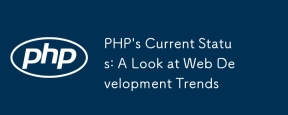
PHP remains important in modern web development, especially in content management and e-commerce platforms. 1) PHP has a rich ecosystem and strong framework support, such as Laravel and Symfony. 2) Performance optimization can be achieved through OPcache and Nginx. 3) PHP8.0 introduces JIT compiler to improve performance. 4) Cloud-native applications are deployed through Docker and Kubernetes to improve flexibility and scalability.
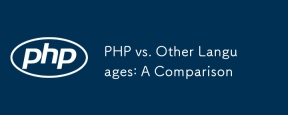
PHP is suitable for web development, especially in rapid development and processing dynamic content, but is not good at data science and enterprise-level applications. Compared with Python, PHP has more advantages in web development, but is not as good as Python in the field of data science; compared with Java, PHP performs worse in enterprise-level applications, but is more flexible in web development; compared with JavaScript, PHP is more concise in back-end development, but is not as good as JavaScript in front-end development.
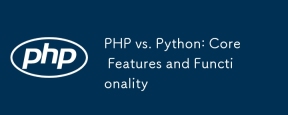
PHP and Python each have their own advantages and are suitable for different scenarios. 1.PHP is suitable for web development and provides built-in web servers and rich function libraries. 2. Python is suitable for data science and machine learning, with concise syntax and a powerful standard library. When choosing, it should be decided based on project requirements.
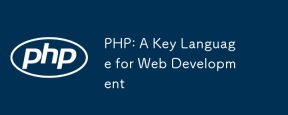
PHP is a scripting language widely used on the server side, especially suitable for web development. 1.PHP can embed HTML, process HTTP requests and responses, and supports a variety of databases. 2.PHP is used to generate dynamic web content, process form data, access databases, etc., with strong community support and open source resources. 3. PHP is an interpreted language, and the execution process includes lexical analysis, grammatical analysis, compilation and execution. 4.PHP can be combined with MySQL for advanced applications such as user registration systems. 5. When debugging PHP, you can use functions such as error_reporting() and var_dump(). 6. Optimize PHP code to use caching mechanisms, optimize database queries and use built-in functions. 7


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
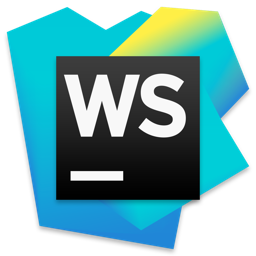
WebStorm Mac version
Useful JavaScript development tools
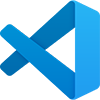
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool