Tips and key points of implementing matrix transposition in numpy
Matrix transposition is a frequently used operation in the fields of data analysis and scientific computing. In numpy, matrix transpose is very simple. This article will introduce the techniques and key points of implementing matrix transposition in numpy, and provide specific code examples.
Point 1: T method of numpy array
Array objects in numpy can be transposed using the T method. The T method is the transpose operation of the matrix, which returns an array with the opposite shape of the original array.
The following is a sample code that uses the T method to transpose a matrix:
import numpy as np # 创建一个2x3的矩阵 matrix = np.array([[1, 2, 3], [4, 5, 6]]) # 输出原始矩阵 print("原始矩阵:") print(matrix) # 使用T方法进行矩阵转置 transposed_matrix = matrix.T # 输出转置后的矩阵 print("转置后的矩阵:") print(transposed_matrix)
Run the above code, you will get the following output:
原始矩阵: [[1 2 3] [4 5 6]] 转置后的矩阵: [[1 4] [2 5] [3 6]]
Point 2: numpy's transpose Function
In addition to using the T method of the array object to transpose the matrix, numpy also provides the transpose function, which can also implement the transpose operation of the matrix.
The following is a sample code that uses the transpose function to transpose a matrix:
import numpy as np # 创建一个2x3的矩阵 matrix = np.array([[1, 2, 3], [4, 5, 6]]) # 输出原始矩阵 print("原始矩阵:") print(matrix) # 使用transpose函数进行矩阵转置 transposed_matrix = np.transpose(matrix) # 输出转置后的矩阵 print("转置后的矩阵:") print(transposed_matrix)
Run the above code, you will get the same output as before.
Point 3: Application of matrix transpose
Matrix transposition is widely used in data analysis and scientific computing. For example, you can use matrix transpose to calculate the inner product of a matrix, matrix multiplication, and so on.
The following is a sample code that uses matrix transpose to calculate the inner product of a matrix:
import numpy as np # 创建两个3x3的矩阵 matrix1 = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9]]) matrix2 = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9]]) # 计算矩阵的内积 inner_product = np.dot(matrix1, matrix2.T) # 输出内积结果 print("矩阵的内积:") print(inner_product)
Run the above code, you will get the following output:
矩阵的内积: [[14 32 50] [32 77 122] [50 122 194]]
Conclusion
This article introduces the techniques and key points of implementing matrix transposition in numpy. We can use the T method or transpose function of the array object to implement the transpose operation of the matrix. Matrix transpose is widely used in data analysis and scientific computing, and can easily perform inner product, matrix multiplication and other operations. I hope this article will help readers understand the techniques and key points of matrix transposition in numpy.
The above is the detailed content of Tips and key points of matrix transpose in numpy. For more information, please follow other related articles on the PHP Chinese website!
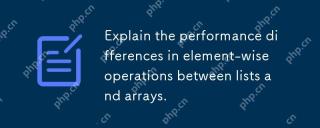
Arraysarebetterforelement-wiseoperationsduetofasteraccessandoptimizedimplementations.1)Arrayshavecontiguousmemoryfordirectaccess,enhancingperformance.2)Listsareflexiblebutslowerduetopotentialdynamicresizing.3)Forlargedatasets,arrays,especiallywithlib
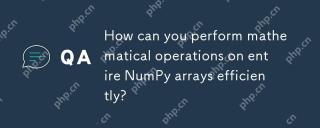
Mathematical operations of the entire array in NumPy can be efficiently implemented through vectorized operations. 1) Use simple operators such as addition (arr 2) to perform operations on arrays. 2) NumPy uses the underlying C language library, which improves the computing speed. 3) You can perform complex operations such as multiplication, division, and exponents. 4) Pay attention to broadcast operations to ensure that the array shape is compatible. 5) Using NumPy functions such as np.sum() can significantly improve performance.
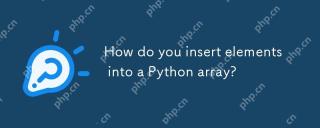
In Python, there are two main methods for inserting elements into a list: 1) Using the insert(index, value) method, you can insert elements at the specified index, but inserting at the beginning of a large list is inefficient; 2) Using the append(value) method, add elements at the end of the list, which is highly efficient. For large lists, it is recommended to use append() or consider using deque or NumPy arrays to optimize performance.
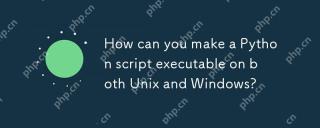
TomakeaPythonscriptexecutableonbothUnixandWindows:1)Addashebangline(#!/usr/bin/envpython3)andusechmod xtomakeitexecutableonUnix.2)OnWindows,ensurePythonisinstalledandassociatedwith.pyfiles,oruseabatchfile(run.bat)torunthescript.
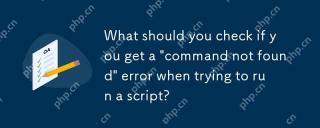
When encountering a "commandnotfound" error, the following points should be checked: 1. Confirm that the script exists and the path is correct; 2. Check file permissions and use chmod to add execution permissions if necessary; 3. Make sure the script interpreter is installed and in PATH; 4. Verify that the shebang line at the beginning of the script is correct. Doing so can effectively solve the script operation problem and ensure the coding process is smooth.
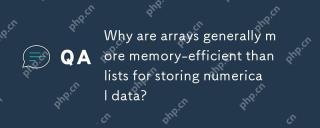
Arraysaregenerallymorememory-efficientthanlistsforstoringnumericaldataduetotheirfixed-sizenatureanddirectmemoryaccess.1)Arraysstoreelementsinacontiguousblock,reducingoverheadfrompointersormetadata.2)Lists,oftenimplementedasdynamicarraysorlinkedstruct
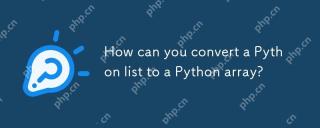
ToconvertaPythonlisttoanarray,usethearraymodule:1)Importthearraymodule,2)Createalist,3)Usearray(typecode,list)toconvertit,specifyingthetypecodelike'i'forintegers.Thisconversionoptimizesmemoryusageforhomogeneousdata,enhancingperformanceinnumericalcomp
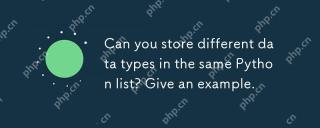
Python lists can store different types of data. The example list contains integers, strings, floating point numbers, booleans, nested lists, and dictionaries. List flexibility is valuable in data processing and prototyping, but it needs to be used with caution to ensure the readability and maintainability of the code.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Linux new version
SublimeText3 Linux latest version

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
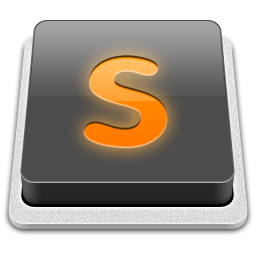
SublimeText3 Mac version
God-level code editing software (SublimeText3)

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
