Introduction to Thread in C#, specific code examples are required
In C#, Thread (thread) is an independent execution path for executing code. By using threads, we can execute multiple tasks in parallel and improve the performance and responsiveness of the program. This article will introduce the basic concepts, usage and related code examples of Thread threads in C#.
1. The basic concept of threads
Threads are the basic execution units in the operating system. In C#, the Thread class is the primary tool for creating and manipulating threads. Threads can perform multiple tasks at the same time, allowing the program to respond to multiple requests at the same time. Threads can perform calculations or perform time-consuming operations in the background of the program without blocking the main thread of the program, thereby improving program performance and user experience.
2. Create and start threads
In C#, use the Thread class to create and start new threads. The following is a simple code example:
using System; using System.Threading; namespace ThreadExample { class Program { // 创建线程执行的方法 static void ThreadMethod() { Console.WriteLine("Thread is running..."); // 线程执行的逻辑代码 for (int i = 0; i < 10; i++) { Console.WriteLine($"Thread: {i}"); Thread.Sleep(1000); // 模拟线程执行耗时操作 } Console.WriteLine("Thread finished."); } static void Main(string[] args) { // 创建新线程并启动 Thread thread = new Thread(ThreadMethod); thread.Start(); // 主线程的逻辑代码 for (int i = 0; i < 5; i++) { Console.WriteLine($"Main: {i}"); Thread.Sleep(500); } // 等待新线程执行完毕 thread.Join(); Console.WriteLine("All threads finished."); } } }
In the above example, we first define a static method ThreadMethod() as the entry point for new thread execution. The Thread.Sleep() method is used to simulate time-consuming operations in threads. In the Main() method, we create a new thread and start it through the Start() method. At the same time, there is also some logic code in the main thread. By calling the Join() method, you can ensure that the program exits after the new thread completes execution.
3. Thread status and control
In the life cycle of a thread, a thread has different states, including: not started, running, blocked, terminated, etc. You can use the ThreadState enumeration type to obtain the state of the thread. In addition, we can also use some methods to control the execution of threads, including: pause, resume, cancel, etc. The following is a sample code:
using System; using System.Threading; namespace ThreadControlExample { class Program { static void Main(string[] args) { // 创建并启动新线程 Thread thread1 = new Thread(ThreadMethod); thread1.Start(); // 暂停线程 Thread.Sleep(2000); // 恢复线程 thread1.Resume(); // 等待线程执行完毕 thread1.Join(); // 创建并启动新线程 Thread thread2 = new Thread(ThreadMethod); thread2.Start(); // 取消线程 thread2.Abort(); Console.WriteLine("Thread control finished."); } static void ThreadMethod() { try { Console.WriteLine("Thread is running..."); for (int i = 0; i < 10; i++) { Console.WriteLine($"Thread: {i}"); Thread.Sleep(1000); } Console.WriteLine("Thread finished."); } catch (ThreadAbortException ex) { Console.WriteLine("Thread aborted."); } } } }
In the example, we pause the execution of the thread through the Sleep() method. Then use the Resume() method to resume the execution of the thread. The Abort() method is used to cancel the execution of a thread. In the ThreadMethod() method, we handle the situation when the thread is canceled by catching the ThreadAbortException exception.
Summary:
The Thread class in C# provides powerful functions to create and operate threads. In multi-threaded programming, we can use threads to execute multiple tasks in parallel to improve program performance and user experience. This article introduces the basic concepts of threads, methods of creating and starting threads, and the status and control of threads. I hope these contents can provide some help for you to understand and use Thread threads in C#.
The above is the detailed content of Overview of Thread threads in C#. For more information, please follow other related articles on the PHP Chinese website!
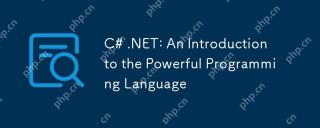
The combination of C# and .NET provides developers with a powerful programming environment. 1) C# supports polymorphism and asynchronous programming, 2) .NET provides cross-platform capabilities and concurrent processing mechanisms, which makes them widely used in desktop, web and mobile application development.
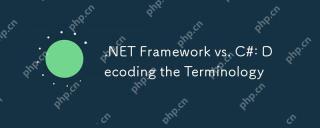
.NETFramework is a software framework, and C# is a programming language. 1..NETFramework provides libraries and services, supporting desktop, web and mobile application development. 2.C# is designed for .NETFramework and supports modern programming functions. 3..NETFramework manages code execution through CLR, and the C# code is compiled into IL and runs by CLR. 4. Use .NETFramework to quickly develop applications, and C# provides advanced functions such as LINQ. 5. Common errors include type conversion and asynchronous programming deadlocks. VisualStudio tools are required for debugging.
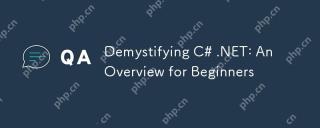
C# is a modern, object-oriented programming language developed by Microsoft, and .NET is a development framework provided by Microsoft. C# combines the performance of C and the simplicity of Java, and is suitable for building various applications. The .NET framework supports multiple languages, provides garbage collection mechanisms, and simplifies memory management.
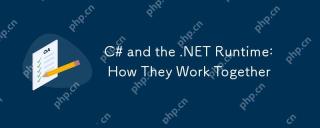
C# and .NET runtime work closely together to empower developers to efficient, powerful and cross-platform development capabilities. 1) C# is a type-safe and object-oriented programming language designed to integrate seamlessly with the .NET framework. 2) The .NET runtime manages the execution of C# code, provides garbage collection, type safety and other services, and ensures efficient and cross-platform operation.
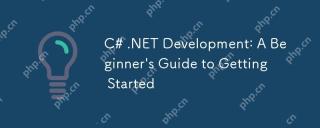
To start C#.NET development, you need to: 1. Understand the basic knowledge of C# and the core concepts of the .NET framework; 2. Master the basic concepts of variables, data types, control structures, functions and classes; 3. Learn advanced features of C#, such as LINQ and asynchronous programming; 4. Be familiar with debugging techniques and performance optimization methods for common errors. With these steps, you can gradually penetrate the world of C#.NET and write efficient applications.
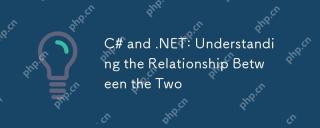
The relationship between C# and .NET is inseparable, but they are not the same thing. C# is a programming language, while .NET is a development platform. C# is used to write code, compile into .NET's intermediate language (IL), and executed by the .NET runtime (CLR).
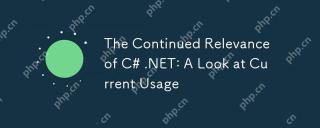
C#.NET is still important because it provides powerful tools and libraries that support multiple application development. 1) C# combines .NET framework to make development efficient and convenient. 2) C#'s type safety and garbage collection mechanism enhance its advantages. 3) .NET provides a cross-platform running environment and rich APIs, improving development flexibility.
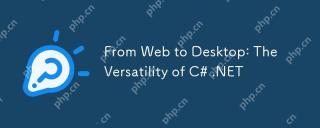
C#.NETisversatileforbothwebanddesktopdevelopment.1)Forweb,useASP.NETfordynamicapplications.2)Fordesktop,employWindowsFormsorWPFforrichinterfaces.3)UseXamarinforcross-platformdevelopment,enablingcodesharingacrossWindows,macOS,Linux,andmobiledevices.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.