Writing exponential function expressions in C language
How to write exponential function expressions in C language
The exponential function is an important function in advanced mathematics. It can be used to solve various practical problems, such as physics Exponential growth and decay in science, interest rate calculation in economic models, etc. In C language, we can use mathematical library functions and custom functions to implement the calculation of exponential function expressions.
1. Use math library functions to implement exponential function expressions
C language provides the math.h library, which defines many mathematics-related functions, including the exponential function exp(). The following is an example code that uses the exp() function in the math.h library to calculate an exponential function expression:
#include <stdio.h> #include <math.h> int main() { double x, result; printf("请输入指数函数的底数x:"); scanf("%lf", &x); result = exp(x); printf("e^%.2f = %.2f ", x, result); return 0; }
In the above code, first we pass #include <math.h> </math.h>
The math.h library is introduced, and then the exp(x)
function is used to calculate the result of the exponential function expression, and finally the calculation result is output through the printf function.
2. Use custom functions to implement exponential function expressions
In addition to using functions in the math.h library, we can also customize functions to implement the calculation of exponential function expressions. The following is a sample code that uses a custom function to calculate an exponential function expression:
#include <stdio.h> double exponent(double x, int power) { double result = 1.0; if (power >= 0) { for (int i = 0; i < power; i++) { result *= x; } } else { for (int i = 0; i < -power; i++) { result /= x; } } return result; } int main() { double x, result; int power; printf("请输入指数函数的底数x:"); scanf("%lf", &x); printf("请输入指数函数的幂次power:"); scanf("%d", &power); result = exponent(x, power); printf("%.2f^%d = %.2f ", x, power, result); return 0; }
In the above code, we define an exponent() function to calculate the result of an exponential function expression. This function contains two parameters x and power, which represent the base and power of the exponential function respectively. Inside the function, we use a loop to implement the calculation of the exponential function. When the power is a positive number, the multiplication operator * is used; when the power is a negative number, the division operator / is used.
Through the above code examples, we can see that whether we use the math.h library function or a custom function, we can realize the calculation of exponential function expressions. According to specific needs and programming habits, we can choose a method that suits us to implement the calculation of exponential function expressions.
The above is the detailed content of Writing exponential function expressions in C language. For more information, please follow other related articles on the PHP Chinese website!
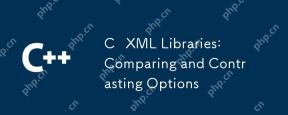
There are four commonly used XML libraries in C: TinyXML-2, PugiXML, Xerces-C, and RapidXML. 1.TinyXML-2 is suitable for environments with limited resources, lightweight but limited functions. 2. PugiXML is fast and supports XPath query, suitable for complex XML structures. 3.Xerces-C is powerful, supports DOM and SAX resolution, and is suitable for complex processing. 4. RapidXML focuses on performance and parses extremely fast, but does not support XPath queries.
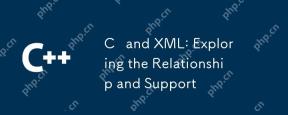
C interacts with XML through third-party libraries (such as TinyXML, Pugixml, Xerces-C). 1) Use the library to parse XML files and convert them into C-processable data structures. 2) When generating XML, convert the C data structure to XML format. 3) In practical applications, XML is often used for configuration files and data exchange to improve development efficiency.
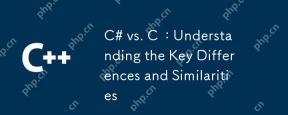
The main differences between C# and C are syntax, performance and application scenarios. 1) The C# syntax is more concise, supports garbage collection, and is suitable for .NET framework development. 2) C has higher performance and requires manual memory management, which is often used in system programming and game development.
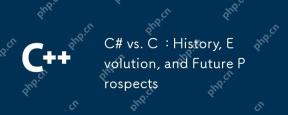
The history and evolution of C# and C are unique, and the future prospects are also different. 1.C was invented by BjarneStroustrup in 1983 to introduce object-oriented programming into the C language. Its evolution process includes multiple standardizations, such as C 11 introducing auto keywords and lambda expressions, C 20 introducing concepts and coroutines, and will focus on performance and system-level programming in the future. 2.C# was released by Microsoft in 2000. Combining the advantages of C and Java, its evolution focuses on simplicity and productivity. For example, C#2.0 introduced generics and C#5.0 introduced asynchronous programming, which will focus on developers' productivity and cloud computing in the future.
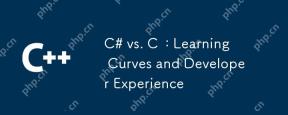
There are significant differences in the learning curves of C# and C and developer experience. 1) The learning curve of C# is relatively flat and is suitable for rapid development and enterprise-level applications. 2) The learning curve of C is steep and is suitable for high-performance and low-level control scenarios.
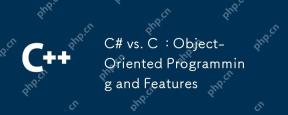
There are significant differences in how C# and C implement and features in object-oriented programming (OOP). 1) The class definition and syntax of C# are more concise and support advanced features such as LINQ. 2) C provides finer granular control, suitable for system programming and high performance needs. Both have their own advantages, and the choice should be based on the specific application scenario.
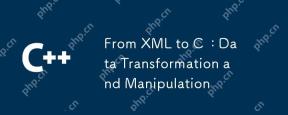
Converting from XML to C and performing data operations can be achieved through the following steps: 1) parsing XML files using tinyxml2 library, 2) mapping data into C's data structure, 3) using C standard library such as std::vector for data operations. Through these steps, data converted from XML can be processed and manipulated efficiently.
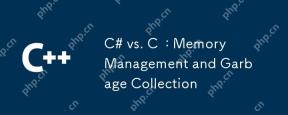
C# uses automatic garbage collection mechanism, while C uses manual memory management. 1. C#'s garbage collector automatically manages memory to reduce the risk of memory leakage, but may lead to performance degradation. 2.C provides flexible memory control, suitable for applications that require fine management, but should be handled with caution to avoid memory leakage.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor
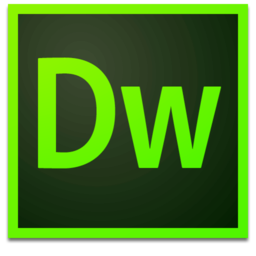
Dreamweaver Mac version
Visual web development tools
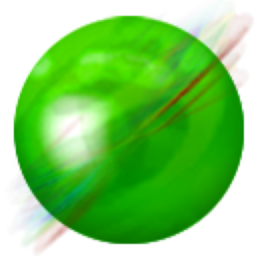
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software