php editor Shinichi introduced an interesting technique to unmarshal from JSON keys containing single quotes. This tip can help developers be more flexible when processing JSON data and avoid parsing errors caused by the inclusion of single quotes. By using some simple tricks and functions, developers can easily handle this situation and ensure correct parsing and processing of JSON data. This technique is very useful for developers who often deal with JSON data, and can improve development efficiency and code quality.
Question content
I am very confused about this. I need to load some data serialized in json (from a French database) where some keys have single quotes.
This is a simplified version:
package main import ( "encoding/json" "fmt" ) type product struct { name string `json:"nom"` cost int64 `json:"prix d'achat"` } func main() { var p product err := json.unmarshal([]byte(`{"nom":"savon", "prix d'achat": 170}`), &p) fmt.printf("product cost: %d\nerror: %s\n", p.cost, err) } // product cost: 0 // error: %!s(<nil>)
Unmarshaling does not result in an error, but "prix d'achat" (p.cost
) is not parsed correctly.
When I unmarshal to map[string]any
, the "prix d'achat" key parses as I expect:
package main import ( "encoding/json" "fmt" ) func main() { blob := map[string]any{} err := json.Unmarshal([]byte(`{"nom":"savon", "prix d'achat": 170}`), &blob) fmt.Printf("blob: %f\nerror: %s\n", blob["prix d'achat"], err) } // blob: 170.000000 // error: %!s(<nil>)
I checked the json.marshal
documentation on struct tags but can't find any issues with the data I'm trying to process.
Am I missing something obvious here? How to parse json keys containing single quotes using struct tags?
Thank you very much for your insights!
Workaround
I didn't find anything in the documentation, but the json encoder treats single quotes as reserved characters in tag names.
func isvalidtag(s string) bool { if s == "" { return false } for _, c := range s { switch { case strings.containsrune("!#$%&()*+-./:;<=>?@[]^_{|}~ ", c): // backslash and quote chars are reserved, but // otherwise any punctuation chars are allowed // in a tag name. case !unicode.isletter(c) && !unicode.isdigit(c): return false } } return true }
I think it's reasonable to ask the question here. In the meantime, you will have to implement json.unmarshaler and/or json.marshaler. This is a start:
func (p *Product) UnmarshalJSON(b []byte) error { type product Product // revent recursion var _p product if err := json.Unmarshal(b, &_p); err != nil { return err } *p = Product(_p) return unmarshalFieldsWithSingleQuotes(p, b) } func unmarshalFieldsWithSingleQuotes(dest interface{}, b []byte) error { // Look through the JSON tags. If there is one containing single quotes, // unmarshal b again, into a map this time. Then unmarshal the value // at the map key corresponding to the tag, if any. var m map[string]json.RawMessage t := reflect.TypeOf(dest).Elem() v := reflect.ValueOf(dest).Elem() for i := 0; i < t.NumField(); i++ { tag := t.Field(i).Tag.Get("json") if !strings.Contains(tag, "'") { continue } if m == nil { if err := json.Unmarshal(b, &m); err != nil { return err } } if j, ok := m[tag]; ok { if err := json.Unmarshal(j, v.Field(i).Addr().Interface()); err != nil { return err } } } return nil }
Try it on the playground: https://www.php.cn/link/9b47b8678d84ea8a0f9fe6c4ec599918一个>
The above is the detailed content of Unmarshal from JSON keys containing single quotes. For more information, please follow other related articles on the PHP Chinese website!
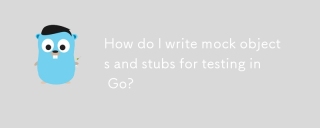
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
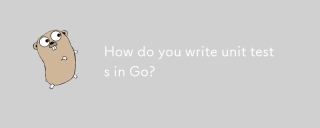
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
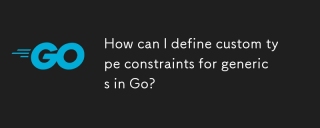
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices
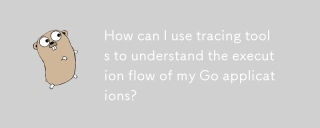
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization
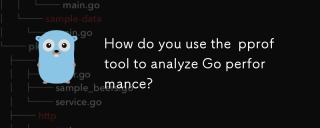
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
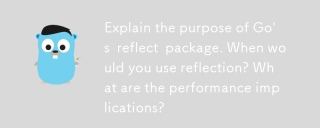
The article discusses Go's reflect package, used for runtime manipulation of code, beneficial for serialization, generic programming, and more. It warns of performance costs like slower execution and higher memory use, advising judicious use and best
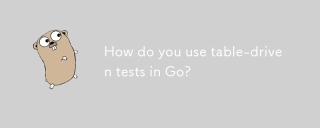
The article discusses using table-driven tests in Go, a method that uses a table of test cases to test functions with multiple inputs and outcomes. It highlights benefits like improved readability, reduced duplication, scalability, consistency, and a
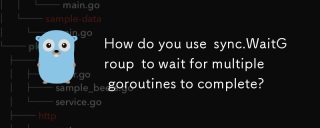
The article explains how to use sync.WaitGroup in Go to manage concurrent operations, detailing initialization, usage, common pitfalls, and best practices.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
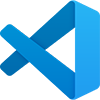
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

Notepad++7.3.1
Easy-to-use and free code editor
