How do you write unit tests in Go?
Writing unit tests in Go is straightforward due to the built-in testing
package. Here's a step-by-step approach to writing unit tests:
-
Create a Test File: For a source file named
foo.go
, create a test file namedfoo_test.go
in the same package. -
Write Test Functions: Inside
foo_test.go
, write functions that start withTest
followed by the name of the function being tested. These functions take a*testing.T
argument. For example:func TestFoo(t *testing.T) { // Test code here }
-
Assertions: Use
t.Error
ort.Errorf
to log errors and fail the test.t.Fatal
ort.Fatalf
can be used to stop the test immediately if something critical fails.if result != expected { t.Errorf("expected %v, but got %v", expected, result) }
-
Run Tests: Execute tests using the
go test
command in the terminal from the directory containing the test files. -
Test Coverage: To get test coverage, run
go test -cover
. -
Table-Driven Tests: Use a table-driven approach to reduce code duplication and test multiple scenarios efficiently.
func TestFoo(t *testing.T) { tests := []struct { input int expected int }{ {1, 2}, {2, 4}, {-1, -2}, } for _, tt := range tests { result := foo(tt.input) if result != tt.expected { t.Errorf("foo(%d) = %d, want %d", tt.input, result, tt.expected) } } }
What are the best practices for writing effective unit tests in Go?
Adhering to best practices in writing unit tests in Go can significantly improve the quality and maintainability of your tests. Here are some key practices:
- Keep Tests Simple and Focused: Each test should cover one specific behavior or scenario. This makes tests easier to understand and maintain.
-
Use Descriptive Names: Name your test functions and variables clearly to convey their purpose. For example,
TestFooReturnsDoubleOfInput
is more descriptive thanTestFoo
. - Utilize Helper Functions: If you find yourself repeating the same setup or assertion logic across multiple tests, move it to a helper function to reduce duplication.
- Test for Error Conditions: Do not only test happy paths; also test how your function behaves with invalid inputs or in error conditions.
-
Parallel Testing: Use
t.Parallel()
to run tests in parallel, which can significantly speed up your test suite, especially for larger projects.func TestFoo(t *testing.T) { t.Parallel() // Test code here }
- Mock Dependencies: Use mocking to isolate the function being tested from external dependencies, allowing for more controlled and reliable tests.
- Achieve High Test Coverage: Aim for high test coverage, but remember that 100% coverage is not always necessary. Focus on critical paths and edge cases.
- Continuous Integration: Integrate your tests into a CI/CD pipeline to ensure tests run automatically on every code change.
How can you use mocking in Go to improve your unit tests?
Mocking in Go can help isolate the unit under test by replacing its dependencies with controlled, fake objects. Here's how you can utilize mocking to enhance your unit tests:
-
Choose a Mocking Library: Popular Go mocking libraries include
GoMock
,testify/mock
, andgomock
. For example, withtestify/mock
:import ( "testing" "github.com/stretchr/testify/mock" ) type MockDependency struct { mock.Mock } func (m *MockDependency) SomeMethod(input string) string { args := m.Called(input) return args.String(0) }
- Create Mock Objects: Define mock objects that mimic the interface of the dependency you wish to mock. Set up expected behavior for these mocks before running the test.
-
Setup Expectations: Before executing the function under test, set up the expected behavior of the mock using the library’s API.
mockDependency := new(MockDependency) mockDependency.On("SomeMethod", "input").Return("output")
- Run the Test: Execute the function you're testing with the mock dependency. The mock will behave as predefined.
-
Assert Mock Calls: After the test, verify that the mock was called as expected.
mockDependency.AssertCalled(t, "SomeMethod", "input")
- Benefits: Mocking allows you to test the behavior of your code in isolation, making tests faster and more reliable. It also allows testing of error scenarios without affecting real services.
What tools can help you manage and run unit tests efficiently in Go?
Several tools can help you manage and run unit tests efficiently in Go. Here’s a list of some popular ones:
-
Go Test Command: The built-in
go test
command is versatile and can be used with various flags to customize the test execution. For example,go test -v
for verbose output orgo test -coverprofile=coverage.out
to generate a coverage report. -
Ginkgo: An advanced testing framework that offers a more readable DSL and better support for integration and end-to-end testing. It can be used in conjunction with
Gomega
for matchers and assertions. -
Testify: A popular suite of test-related packages that includes
require
for test assertions,assert
for fluent assertions,suite
for test organization, andmock
for mocking dependencies. - GoConvey: An alternative testing framework that provides a more interactive and visual way of writing tests and viewing results, which is particularly useful for TDD.
- Delve: A debugger for Go that can be used to step through tests, helping you diagnose and resolve issues more efficiently.
- Coveralls: A tool that can be integrated into your CI pipeline to track and report on test coverage over time, helping to ensure your test suite remains effective.
- CircleCI, Travis CI, or GitHub Actions: These continuous integration services can automate the running of your tests, providing immediate feedback on the health of your codebase after every commit.
Using these tools in combination can help streamline your testing process, improve test coverage, and make your test suite more maintainable and efficient.
The above is the detailed content of How do you write unit tests in Go?. For more information, please follow other related articles on the PHP Chinese website!
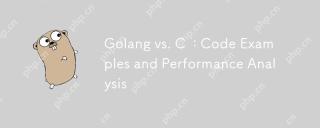
Golang is suitable for rapid development and concurrent programming, while C is more suitable for projects that require extreme performance and underlying control. 1) Golang's concurrency model simplifies concurrency programming through goroutine and channel. 2) C's template programming provides generic code and performance optimization. 3) Golang's garbage collection is convenient but may affect performance. C's memory management is complex but the control is fine.
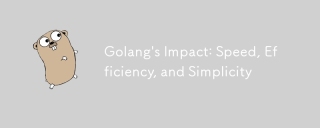
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
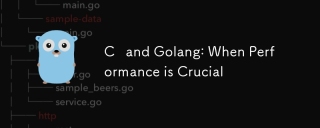
C is more suitable for scenarios where direct control of hardware resources and high performance optimization is required, while Golang is more suitable for scenarios where rapid development and high concurrency processing are required. 1.C's advantage lies in its close to hardware characteristics and high optimization capabilities, which are suitable for high-performance needs such as game development. 2.Golang's advantage lies in its concise syntax and natural concurrency support, which is suitable for high concurrency service development.
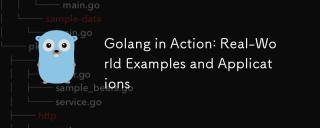
Golang excels in practical applications and is known for its simplicity, efficiency and concurrency. 1) Concurrent programming is implemented through Goroutines and Channels, 2) Flexible code is written using interfaces and polymorphisms, 3) Simplify network programming with net/http packages, 4) Build efficient concurrent crawlers, 5) Debugging and optimizing through tools and best practices.
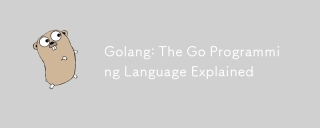
The core features of Go include garbage collection, static linking and concurrency support. 1. The concurrency model of Go language realizes efficient concurrent programming through goroutine and channel. 2. Interfaces and polymorphisms are implemented through interface methods, so that different types can be processed in a unified manner. 3. The basic usage demonstrates the efficiency of function definition and call. 4. In advanced usage, slices provide powerful functions of dynamic resizing. 5. Common errors such as race conditions can be detected and resolved through getest-race. 6. Performance optimization Reuse objects through sync.Pool to reduce garbage collection pressure.
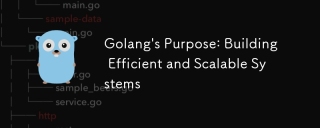
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
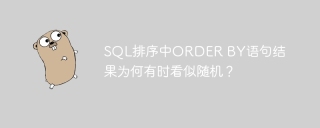
Confused about the sorting of SQL query results. In the process of learning SQL, you often encounter some confusing problems. Recently, the author is reading "MICK-SQL Basics"...
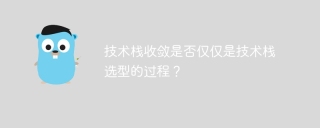
The relationship between technology stack convergence and technology selection In software development, the selection and management of technology stacks are a very critical issue. Recently, some readers have proposed...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Zend Studio 13.0.1
Powerful PHP integrated development environment

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
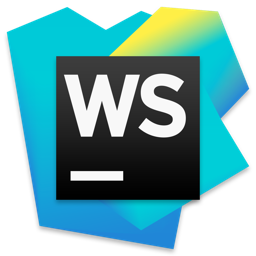
WebStorm Mac version
Useful JavaScript development tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
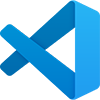
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft