


Explain the purpose of Go's reflect package. When would you use reflection? What are the performance implications?
The reflect
package in Go is a powerful tool that provides the ability to examine and modify the structure and behavior of values at runtime. Its primary purpose is to enable metaprogramming, allowing programmers to write code that can manipulate other code dynamically.
You would use reflection in Go in scenarios where you need to inspect or modify values of unknown types at runtime, which is not possible with static typing alone. Common use cases include serialization and deserialization of data, creating generic functions or data structures, and implementing interfaces dynamically.
However, using reflection comes with performance implications. Reflection is slower than direct method calls and type assertions because it involves additional runtime checks and indirections. It can also lead to increased memory usage since the reflection system needs to keep track of type information at runtime. Therefore, it should be used judiciously and only when necessary.
What specific scenarios benefit from using Go's reflect package?
Several specific scenarios can benefit from using Go's reflect
package:
- Serialization and Deserialization: When dealing with data that needs to be converted to and from various formats like JSON or XML, reflection can help automate the process by examining the structure of Go types at runtime.
- Generic Programming: Although Go does not support traditional generics, reflection can be used to create pseudo-generic functions and data structures. For example, a function that can sort slices of any comparable type can be implemented using reflection.
- Dependency Injection: Reflection can be useful in frameworks that need to inject dependencies into structs, allowing for more flexible and modular code.
- Testing and Debugging: Reflection can be used to write more comprehensive test suites or debugging tools, by allowing you to inspect and modify values within your program at runtime.
- Dynamic Interface Implementation: Reflection allows you to check if a value implements a certain interface at runtime, which can be useful in scenarios where you need to handle objects of different types in a generic way.
How does reflection in Go affect the performance of your applications?
Reflection in Go can significantly impact the performance of your applications in several ways:
- Increased Execution Time: Operations performed using reflection, such as type assertions and method calls, are slower than their non-reflective counterparts. This is because reflection requires additional runtime checks and type lookups.
- Higher Memory Usage: Reflection requires maintaining type information at runtime, which can lead to increased memory usage. This is particularly relevant in long-running applications where memory efficiency is crucial.
- Garbage Collection Overhead: The use of reflection can increase the frequency of garbage collection, as the reflect package creates temporary values and type descriptors that need to be managed by the garbage collector.
- Loss of Compile-Time Safety: Since reflection bypasses compile-time type checking, it can lead to runtime errors that would otherwise be caught at compile time, potentially affecting the reliability and performance of your application.
Are there any best practices to follow when using Go's reflect package to minimize performance issues?
To minimize performance issues when using Go's reflect
package, consider the following best practices:
- Use Reflection Sparingly: Only use reflection when absolutely necessary. If possible, prefer static typing and compile-time checks to ensure better performance and maintainability.
- Cache Reflection Results: If you need to use reflection repeatedly on the same types or values, cache the results of reflection operations. This can help avoid redundant type lookups and improve performance.
- Avoid Deep Reflection: Try to minimize the depth of reflection, especially in loops. Deep reflection can lead to significant performance degradation due to the cumulative effect of runtime checks.
- Profile and Optimize: Use Go's profiling tools to identify performance bottlenecks caused by reflection. Optimize your code based on profiling results, possibly by reducing the use of reflection or finding alternative approaches.
- Consider Alternatives: Before resorting to reflection, explore alternative solutions that might achieve the same goal without the performance overhead. For example, interfaces can often be used instead of reflection for type-safe generic programming.
- Document Reflection Usage: Clearly document where and why reflection is being used in your codebase. This helps other developers understand the trade-offs and maintain the code more effectively.
By following these best practices, you can mitigate the performance impact of using Go's reflect
package and ensure that your applications remain efficient and maintainable.
The above is the detailed content of Explain the purpose of Go's reflect package. When would you use reflection? What are the performance implications?. For more information, please follow other related articles on the PHP Chinese website!
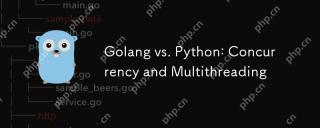
Golang is more suitable for high concurrency tasks, while Python has more advantages in flexibility. 1.Golang efficiently handles concurrency through goroutine and channel. 2. Python relies on threading and asyncio, which is affected by GIL, but provides multiple concurrency methods. The choice should be based on specific needs.
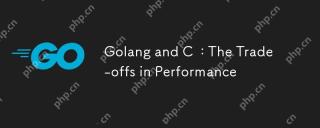
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
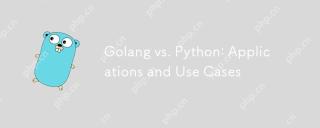
ChooseGolangforhighperformanceandconcurrency,idealforbackendservicesandnetworkprogramming;selectPythonforrapiddevelopment,datascience,andmachinelearningduetoitsversatilityandextensivelibraries.

Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
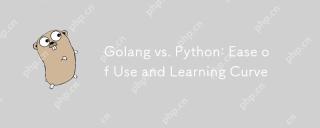
In what aspects are Golang and Python easier to use and have a smoother learning curve? Golang is more suitable for high concurrency and high performance needs, and the learning curve is relatively gentle for developers with C language background. Python is more suitable for data science and rapid prototyping, and the learning curve is very smooth for beginners.
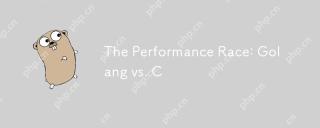
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
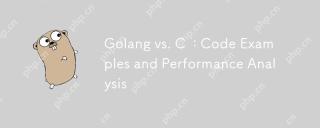
Golang is suitable for rapid development and concurrent programming, while C is more suitable for projects that require extreme performance and underlying control. 1) Golang's concurrency model simplifies concurrency programming through goroutine and channel. 2) C's template programming provides generic code and performance optimization. 3) Golang's garbage collection is convenient but may affect performance. C's memory management is complex but the control is fine.
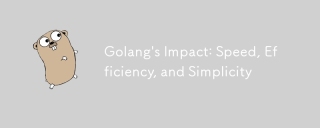
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SublimeText3 Chinese version
Chinese version, very easy to use
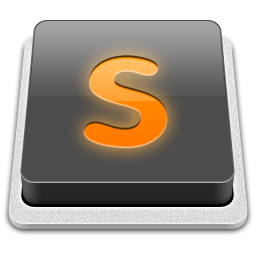
SublimeText3 Mac version
God-level code editing software (SublimeText3)