When using OAuth 2.0 Resource Server JWT, you sometimes encounter permission (authorization) issues. This is a challenge that many developers often encounter during the development process. OAuth 2.0 is an open standard for authorization that allows users to authorize third-party applications to access their protected resources. JWT (JSON Web Token) is a way of passing claims between web applications. However, in practice, some permission problems may occur, such as authorization failure, failure to obtain access tokens, etc. This article will analyze these issues and provide some solutions to help developers better use OAuth 2.0 resource server JWT.
Question content
I am trying to use oauth 2.0 resource server jwt to configure access authorization using jwt in a simple application.
The whole authentication part works fine, but I'm having trouble with authorization. All protected endpoints give 403 forbidden
errors even though the correct permissions are present in the token.
I tried using the default scope (scope_) attribute and changing the configuration to role (role_), but the problem persists.
Does anyone know how to solve it?
Full source code: https://github.com/gustavosc1/spring-security-jwt
Generate token example: eyjhbgcioijsuzi1nij9.eyjpc3mioijzchjpbmctc2vjdxjpdhktand0iiwic3viijoidxnlcm5hbwuilcjlehaioje3mdu0ndmyotqsimlhdci6mtcwntqwnzi5ncwicm9szxmi oijst0xfx0fetuloin0. peivwrthx_7mr6eefqbid5dplhffzcvd7scmmt3f7rk7sk1i6kerpqi5ubdvaefnzsjq6vka5nadltsbqidfzogmoixjktfhsc5zrnyyrhikvnwcwb3wrgdd1ezgihaldfjxwxsyypau njwdxznirkl93e6mg1uao5piy9p- 9yp8jer7o31wkdr1coskzk3gqw42uecib9h1rrlkx9pdk7pf9rtfssfcwc-ntvismrycreco9rialqfydpdzeojimcbqveyboqfhn2woepgdm8mr5zsdhgdq e1ivsibfbcj_0486zuqiksxp2kniljhl2b5qnan0 7fjpvslk--ccsg
Security configuration:
@configuration @enablewebsecurity //@enablemethodsecurity(prepostenabled = true) public class securityconfig { @value("${jwt.public.key}") private rsapublickey key; @value("${jwt.private.key}") private rsaprivatekey priv; @bean securityfilterchain filterchain(httpsecurity http) throws exception { http.csrf(csrf -> csrf.disable()) .authorizehttprequests( auth -> auth .requestmatchers("/authenticate").permitall() .requestmatchers("/register").permitall() .requestmatchers("/private").hasanyrole("admin")) .httpbasic(customizer.withdefaults()) // https://docs-spring-io.translate.goog/spring-security/reference/servlet/oauth2/resource-server/jwt.html?_x_tr_sl=en&_x_tr_tl=pt&_x_tr_hl=pt-br&_x_tr_pto=sc .oauth2resourceserver( conf -> conf.jwt( jwt -> jwt.decoder(jwtdecoder()) .jwtauthenticationconverter(jwtauthenticationconverter()))); return http.build(); } @bean public jwtauthenticationconverter jwtauthenticationconverter() { jwtgrantedauthoritiesconverter grantedauthoritiesconverter = new jwtgrantedauthoritiesconverter(); grantedauthoritiesconverter.setauthoritiesclaimname("roles"); grantedauthoritiesconverter.setauthorityprefix("role_"); jwtauthenticationconverter jwtauthenticationconverter = new jwtauthenticationconverter(); jwtauthenticationconverter.setjwtgrantedauthoritiesconverter(grantedauthoritiesconverter); return jwtauthenticationconverter; } @bean jwtdecoder jwtdecoder() { return nimbusjwtdecoder.withpublickey(this.key).build(); } @bean jwtencoder jwtencoder() { jwk jwk = new rsakey.builder(this.key).privatekey(this.priv).build(); jwksource<securitycontext> jwks = new immutablejwkset<>(new jwkset(jwk)); return new nimbusjwtencoder(jwks); } @bean passwordencoder passwordencoder() { return new bcryptpasswordencoder(); } }
userdetailsserviceimpl:
@service public class userdetailsserviceimpl implements userdetailsservice { private final userrepository userrepository; public userdetailsserviceimpl(userrepository userrepository) { this.userrepository = userrepository; } @override public userdetails loaduserbyusername(string username) throws usernamenotfoundexception { optional<user> useroptional = userrepository.findbyusername(username); user user = useroptional.orelsethrow(() -> new usernamenotfoundexception("user not found with username: " + username)); return new userauthenticated(user.getusername(), user.getpassword()); } }
User verified:
public class userauthenticated implements userdetails { private string username; private string password; public userauthenticated(string username, string password) { this.username = username; this.password = password; } @override public string getusername() { return username; } @override public string getpassword() { return password; } @override public collection<? extends grantedauthority> getauthorities() { return list.of(() -> "role_admin"); } @override public boolean isaccountnonexpired() { return true; } @override public boolean isaccountnonlocked() { return true; } @override public boolean iscredentialsnonexpired() { return true; } @override public boolean isenabled() { return true; } }
jwtservice:
@service public class jwtservice { private final jwtencoder encoder; public jwtservice(jwtencoder encoder) { this.encoder = encoder; } public string generatetoken(authentication authentication) { instant now = instant.now(); long expiry = 36000l; string scope = authentication .getauthorities().stream() .map(grantedauthority::getauthority) .collect(collectors .joining(" ")); jwtclaimsset claims = jwtclaimsset.builder() .issuer("spring-security-jwt") .issuedat(now) .expiresat(now.plusseconds(expiry)) .subject(authentication.getname()) .claim("roles", scope) .build(); return encoder.encode( jwtencoderparameters.from(claims)) .gettokenvalue(); } }
Private Controller:
@RestController @RequestMapping("private") public class PrivateController { @GetMapping //@PreAuthorize("hasAuthority('ROLE_ADMIN')") public String getMessage() { return "Hello from private API controller"; } }
Workaround
The provided token was generated and the roles
field was set to ROLE_ADMIN
. In jwtAuthenticationConverter()
, you try to use setAuthorityPrefix
with ROLE
, resulting in ROLE_ROLE_ADMIN
.
To correct this problem, modify this line to grantedAuthoritiesConverter.setAuthorityPrefix("");
.
After making this adjustment, the issue should be resolved. If you encounter any other issues please let me know.
Notice:
If you omit this step, the default prefix will be SCOPE
, causing your role to become SCOPE_ROLE_ADMIN
.
The above is the detailed content of Permissions (Authorization) Issues Using OAuth 2.0 Resource Server JWT. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SublimeText3 Linux new version
SublimeText3 Linux latest version
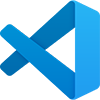
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
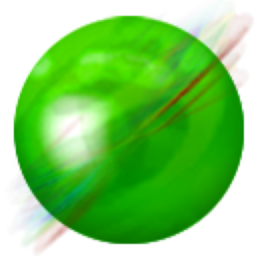
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
