I've spent hours trying to understand the underlying logic but making no progress. The code below returns a deadlock after the first iteration. If I close the writer before io.copy
the deadlock goes away but nothing is printed (because the writing end of the pipe is closed before reading)
func main() { reader, writer := io.pipe() c := make(chan string) go func() { for i := 0; i < 5; i++ { text := fmt.sprintf("hello %vth time", i+1) c <- text } close(c) }() for msg := range c { msg = fmt.sprintf("\nreceived from channel -> %v\n", msg) go fmt.fprint(writer, msg) io.copy(os.stdout, reader) writer.close() } }
This is the error after running the code
received from channel -> hello 1th time fatal error: all goroutines are asleep - deadlock! goroutine 1 [select]: io.(*pipe).read(0xc000130120, {0xc00013e000, 0x8000, 0xc00011e001?}) /usr/lib/go/src/io/pipe.go:57 +0xb1 io.(*PipeReader).Read(0x0?, {0xc00013e000?, 0xc00011e050?, 0x10?}) /usr/lib/go/src/io/pipe.go:136 +0x25 io.copyBuffer({0x4bde98, 0xc00011e050}, {0x4bddb8, 0xc00012e018}, {0x0, 0x0, 0x0}) /usr/lib/go/src/io/io.go:427 +0x1b2 io.Copy(...) /usr/lib/go/src/io/io.go:386 os.genericReadFrom(0x101c00002c500?, {0x4bddb8, 0xc00012e018}) /usr/lib/go/src/os/file.go:161 +0x67 os.(*File).ReadFrom(0xc00012e008, {0x4bddb8, 0xc00012e018}) /usr/lib/go/src/os/file.go:155 +0x1b0 io.copyBuffer({0x4bde38, 0xc00012e008}, {0x4bddb8, 0xc00012e018}, {0x0, 0x0, 0x0}) /usr/lib/go/src/io/io.go:413 +0x14b io.Copy(...) /usr/lib/go/src/io/io.go:386 main.pipetest() /home/stranger/source-code/golang/ipctest/pipes/main.go:39 +0x1ae main.main() /home/stranger/source-code/golang/ipctest/pipes/main.go:10 +0x17 goroutine 18 [chan send]: main.pipetest.func1() /home/stranger/source-code/golang/ipctest/pipes/main.go:29 +0x85 created by main.pipetest /home/stranger/source-code/golang/ipctest/pipes/main.go:26 +0x17a exit status 2
Correct answer
io.copy
Keep trying to copy until the reader reaches eof (in this case, when the pipe is closed ). Since you call writer.close()
after
after io.copy ends, io.copy
will never be seen eof, and hangs forever.
Another problem with your code is that you are trying to close the pipe multiple times (each time the loop code repeats). In general, a close
able object should be closed only once and is assumed to be unavailable after close
d. If you need to reuse them, you should create a new instance.
This is a working revision of the code:
func main() { c := make(chan string) go func() { for i := 0; i < 5; i++ { text := fmt.Sprintf("hello %vth time", i+1) c <- text } close(c) }() for msg := range c { msg = fmt.Sprintf("\nreceived from channel -> %v\n", msg) // Create a new pipe for this message. reader, writer := io.Pipe() go func() { fmt.Fprint(writer, msg) // Close the pipe after writing the message. writer.Close() }() io.Copy(os.Stdout, reader) } }
The above is the detailed content of io.Pipe and deadlock when trying to write/read. For more information, please follow other related articles on the PHP Chinese website!
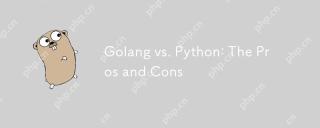
Golangisidealforbuildingscalablesystemsduetoitsefficiencyandconcurrency,whilePythonexcelsinquickscriptinganddataanalysisduetoitssimplicityandvastecosystem.Golang'sdesignencouragesclean,readablecodeanditsgoroutinesenableefficientconcurrentoperations,t
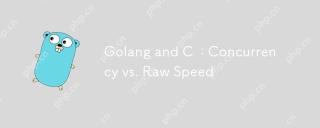
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
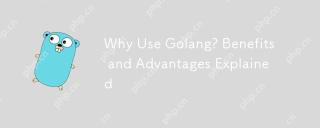
Reasons for choosing Golang include: 1) high concurrency performance, 2) static type system, 3) garbage collection mechanism, 4) rich standard libraries and ecosystems, which make it an ideal choice for developing efficient and reliable software.
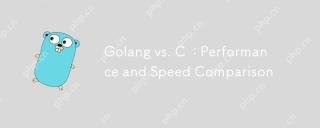
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
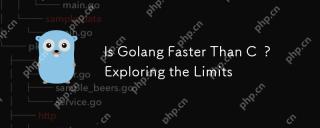
Golang performs better in compilation time and concurrent processing, while C has more advantages in running speed and memory management. 1.Golang has fast compilation speed and is suitable for rapid development. 2.C runs fast and is suitable for performance-critical applications. 3. Golang is simple and efficient in concurrent processing, suitable for concurrent programming. 4.C Manual memory management provides higher performance, but increases development complexity.
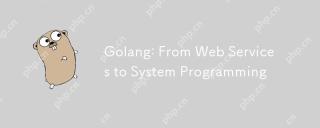
Golang's application in web services and system programming is mainly reflected in its simplicity, efficiency and concurrency. 1) In web services, Golang supports the creation of high-performance web applications and APIs through powerful HTTP libraries and concurrent processing capabilities. 2) In system programming, Golang uses features close to hardware and compatibility with C language to be suitable for operating system development and embedded systems.
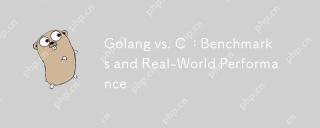
Golang and C have their own advantages and disadvantages in performance comparison: 1. Golang is suitable for high concurrency and rapid development, but garbage collection may affect performance; 2.C provides higher performance and hardware control, but has high development complexity. When making a choice, you need to consider project requirements and team skills in a comprehensive way.
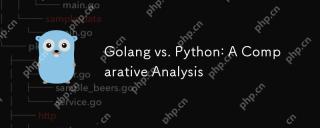
Golang is suitable for high-performance and concurrent programming scenarios, while Python is suitable for rapid development and data processing. 1.Golang emphasizes simplicity and efficiency, and is suitable for back-end services and microservices. 2. Python is known for its concise syntax and rich libraries, suitable for data science and machine learning.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.