I am trying to read a large file one line at a time. I found a question on Quora covering this topic, but I'm missing some connections to make the whole thing come together.
var Lazy=require("lazy"); new Lazy(process.stdin) .lines .forEach( function(line) { console.log(line.toString()); } ); process.stdin.resume();
What I'm trying to figure out is how to read from a file one line at a time, rather than reading from STDIN like in this example.
I have tried before:
fs.open('./VeryBigFile.csv', 'r', '0666', Process); function Process(err, fd) { if (err) throw err; // DO lazy read }
But it doesn't work. I know in a pinch I could fall back to something like PHP, but I want to figure this out.
I think the other answer won't work because the file is much larger than the memory of the server I'm running it on.
Correct answer
Since Node.js v0.12 and Node.js v4.0.0, there is a stable readline core module. This is the simplest way to read lines from a file without any external modules:
<code>const fs = require('fs'); const readline = require('readline'); async function processLineByLine() { const fileStream = fs.createReadStream('input.txt'); const rl = readline.createInterface({ input: fileStream, crlfDelay: Infinity }); // Note: we use the crlfDelay option to recognize all instances of CR LF // ('\r\n') in input.txt as a single line break. for await (const line of rl) { // Each line in input.txt will be successively available here as `line`. console.log(`Line from file: ${line}`); } } processLineByLine(); </code>
or:
var lineReader = require('readline').createInterface({ input: require('fs').createReadStream('file.in') }); lineReader.on('line', function (line) { console.log('Line from file:', line); }); lineReader.on('close', function () { console.log('all done, son'); });
The last line is read correctly even without the final \n
(starting with Node v0.12 or later).
UPDATE: This example has been added to Node's official API documentation.
The above is the detailed content of Reading a file one line at a time in node.js?. For more information, please follow other related articles on the PHP Chinese website!
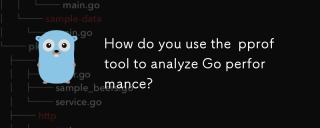
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
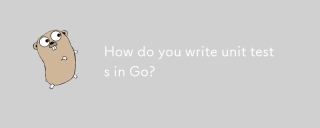
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
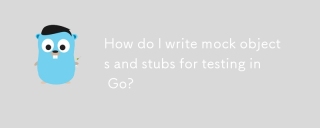
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
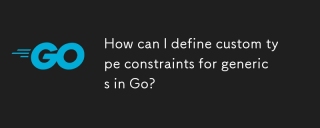
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices
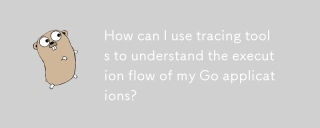
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization
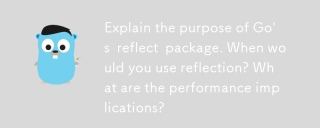
The article discusses Go's reflect package, used for runtime manipulation of code, beneficial for serialization, generic programming, and more. It warns of performance costs like slower execution and higher memory use, advising judicious use and best
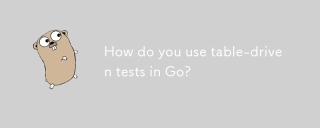
The article discusses using table-driven tests in Go, a method that uses a table of test cases to test functions with multiple inputs and outcomes. It highlights benefits like improved readability, reduced duplication, scalability, consistency, and a
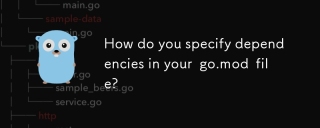
The article discusses managing Go module dependencies via go.mod, covering specification, updates, and conflict resolution. It emphasizes best practices like semantic versioning and regular updates.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
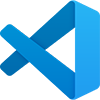
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

Zend Studio 13.0.1
Powerful PHP integrated development environment
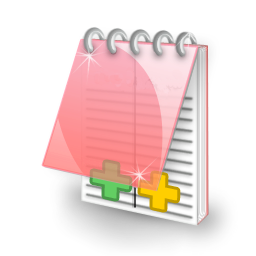
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
