Application challenges and solutions for databases in Go language
Challenges and solutions for using databases in Go language
With the rapid development of the Internet, databases have become one of the core technologies for storing and managing data. As an efficient and easy-to-use programming language, Go language is also becoming increasingly popular in database applications. However, there are also some challenges when using databases in Go language. This article will explore these challenges and give corresponding solutions.
- Database driver selection
The Go language provides some commonly used database drivers, such as MySQL, PostgreSQL, SQLite, etc., and developers are faced with the problem of choice when using them. Different database drivers have different interfaces and functional features, and should be selected based on actual needs.
Solution: When choosing a database driver, you can consider the following factors:
- Stability and activity of the driver: Choose a database that is maintained by an active community and has undergone long-term Verify stable driver.
- Ease of use of API: Choosing a driver with a simple and easy-to-use interface can reduce the workload of development and maintenance.
- Functional features: Consider whether certain specific functions are needed based on actual needs, such as transaction processing, connection pooling, etc.
- Connection Management
When using a database, connections are essential. However, too many or too few connections can affect system performance. Too many connections may lead to a waste of resources, and too few connections may cause the system to respond untimely.
Solution: Correctly managing database connections is an important part of ensuring system performance. The following strategies can be adopted:
- Use connection pool: By using the connection pool, you can effectively manage database connections, avoid frequent creation and destruction of connections, and improve system performance.
- Use connection timeout: Set the maximum usage time of the connection. Connections that are not released after this time will be recycled to avoid the connection taking too long and affecting the availability of the system.
- Reasonable connection number setting: Set the number of database connections reasonably according to actual needs and system load to avoid too many or too few.
- Database operations
Database operations include operations such as add, delete, modify, and query. How to perform database operations efficiently is one of the challenges we need to face.
Solution:
- Use transactions: In scenarios where data consistency needs to be ensured, using transactions can ensure the atomicity of a series of operations and ensure the integrity of the data. .
- Batch operation: Batch operation can reduce the number of database connections and improve operation efficiency. For example, you can use MySQL's
INSERT INTO ... VALUES (...), (...), (...)
syntax to insert multiple pieces of data at once. - Use indexes: Adding indexes to fields that require frequent queries can speed up queries.
- Error handling
During database operation, it is inevitable to encounter various errors. How to handle these errors correctly and ensure the reliability of the system is an issue that needs to be considered.
Solution: When performing database operations, you should pay attention to the following aspects:
- Error handling: When performing database operations, you must correctly handle possible errors. mistake. You can use the error mechanism in the Go language for error handling, and adopt different processing logic according to different error types.
- Transaction rollback: When an error occurs, the transaction needs to be rolled back in time to ensure data consistency.
- Logging: For the occurrence of errors, logs need to be recorded for troubleshooting and problem location.
To sum up, there are some challenges when using databases in Go language, but there are also corresponding solutions. The correct selection of database drivers, reasonable management of connections, efficient database operation and correct error handling will have a positive impact on the performance and reliability of the system. At the same time, database operations should be continuously tuned and optimized according to specific business needs and system pressure to improve system performance and stability.
Sample code:
import ( "database/sql" "log" _ "github.com/go-sql-driver/mysql" ) func main() { db, err := sql.Open("mysql", "user:password@tcp(127.0.0.1:3306)/database") if err != nil { log.Fatal(err) } defer db.Close() // Insert data _, err = db.Exec("INSERT INTO users (name, age) VALUES (?, ?)", "Alice", 28) if err != nil { log.Fatal(err) } // Query data rows, err := db.Query("SELECT * FROM users") if err != nil { log.Fatal(err) } defer rows.Close() for rows.Next() { var name string var age int err := rows.Scan(&name, &age) if err != nil { log.Fatal(err) } log.Println(name, age) } // Update data _, err = db.Exec("UPDATE users SET age = ? WHERE name = ?", 29, "Alice") if err != nil { log.Fatal(err) } // Delete data _, err = db.Exec("DELETE FROM users WHERE name = ?", "Alice") if err != nil { log.Fatal(err) } }
The above is a simple example of Go language using MySQL database. By using appropriate drivers and correct operating methods, you can simply implement database addition, deletion, modification, and query operations, as well as error handling and logging. In actual applications, more complex and flexible database operations need to be performed based on actual needs.
Summary:
Through reasonable selection of database drivers, correct management of connections, efficient operation of the database, and correct handling of errors, the challenges faced by using databases in the Go language can be solved. At the same time, rationally tuning database operations and optimizing according to actual needs can improve system performance and stability. Through continuous learning and practice, you will be able to flexibly use database technology to develop efficient and reliable applications.
The above is the detailed content of Application challenges and solutions for databases in Go language. For more information, please follow other related articles on the PHP Chinese website!
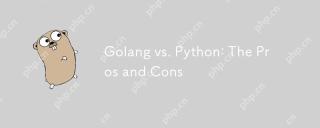
Golangisidealforbuildingscalablesystemsduetoitsefficiencyandconcurrency,whilePythonexcelsinquickscriptinganddataanalysisduetoitssimplicityandvastecosystem.Golang'sdesignencouragesclean,readablecodeanditsgoroutinesenableefficientconcurrentoperations,t
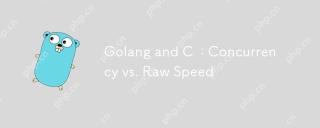
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
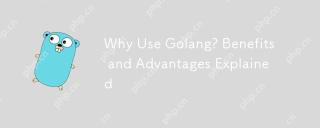
Reasons for choosing Golang include: 1) high concurrency performance, 2) static type system, 3) garbage collection mechanism, 4) rich standard libraries and ecosystems, which make it an ideal choice for developing efficient and reliable software.
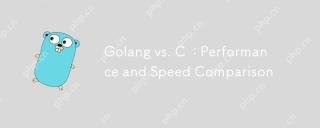
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
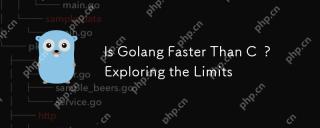
Golang performs better in compilation time and concurrent processing, while C has more advantages in running speed and memory management. 1.Golang has fast compilation speed and is suitable for rapid development. 2.C runs fast and is suitable for performance-critical applications. 3. Golang is simple and efficient in concurrent processing, suitable for concurrent programming. 4.C Manual memory management provides higher performance, but increases development complexity.
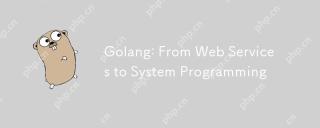
Golang's application in web services and system programming is mainly reflected in its simplicity, efficiency and concurrency. 1) In web services, Golang supports the creation of high-performance web applications and APIs through powerful HTTP libraries and concurrent processing capabilities. 2) In system programming, Golang uses features close to hardware and compatibility with C language to be suitable for operating system development and embedded systems.
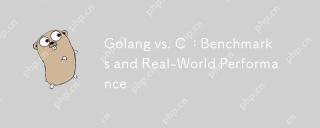
Golang and C have their own advantages and disadvantages in performance comparison: 1. Golang is suitable for high concurrency and rapid development, but garbage collection may affect performance; 2.C provides higher performance and hardware control, but has high development complexity. When making a choice, you need to consider project requirements and team skills in a comprehensive way.
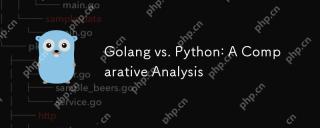
Golang is suitable for high-performance and concurrent programming scenarios, while Python is suitable for rapid development and data processing. 1.Golang emphasizes simplicity and efficiency, and is suitable for back-end services and microservices. 2. Python is known for its concise syntax and rich libraries, suitable for data science and machine learning.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
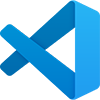
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SublimeText3 English version
Recommended: Win version, supports code prompts!

Atom editor mac version download
The most popular open source editor