


Learn and master Golang's concurrent programming technology from scratch
Introduction to concurrent programming in Golang: Mastering concurrency technology from scratch requires specific code examples
Introduction:
In today's rapidly developing field of computer science, how to fully Exploiting the parallel capabilities of multi-core processors has become an important topic. Concurrent programming, the execution of multiple tasks simultaneously, has become an important feature of modern programming languages. In this regard, Golang, as a programming language that supports efficient concurrent programming, has been widely favored by developers. This article will introduce the basic concurrent programming concepts of Golang from scratch, and help readers better understand and master the concurrency technology in Golang through specific code examples.
1. Golang’s concurrency model
Golang adopts the CSP (Communicating Sequential Process) model to implement concurrent programming. In this model, different concurrent entities collaborate through communication rather than shared memory. This can effectively avoid some common problems in multi-threaded programming, such as data competition. Golang provides some keywords (such as goroutine, channel, etc.) to simplify concurrent programming, making it easier for developers to use concurrency technology.
2. Goroutine in Golang
Goroutine is the basic unit for achieving concurrency in Golang. Goroutine is a lightweight thread that can execute blocks of code concurrently. Compared with traditional threads, the overhead of creating and destroying Goroutines is smaller, so more Goroutines can be created to process tasks in parallel. The following is a simple sample code that shows how to use Goroutine to execute tasks concurrently:
package main import ( "fmt" "time" ) func task1() { for i := 0; i < 10; i++ { fmt.Println("Task 1: ", i) time.Sleep(time.Millisecond * 500) } } func task2() { for i := 0; i < 10; i++ { fmt.Println("Task 2: ", i) time.Sleep(time.Millisecond * 500) } } func main() { go task1() go task2() // 等待两个任务完成 time.Sleep(time.Second * 6) }
In this code, the task1
and task2
functions represent two needs Concurrently executed tasks. By using the go
keyword, we can start both tasks at the same time in the main
function. Since Golang's scheduler can intelligently manage the execution of concurrent tasks, these two tasks will be executed concurrently. Finally, we wait for the completion of the two tasks by calling the time.Sleep
method. Run the program and you can see the results of the two tasks being executed alternately.
3. Channel in Golang
Channel is a mechanism for concurrent communication in Golang, which can be used to transfer data between Goroutines. Channel can be used to both send data and receive data. Goroutine can send data to another Goroutine through Channel, and can also receive data sent by other Goroutine through Channel.
The following is a sample code that uses Channel for concurrent communication:
package main import "fmt" func process(ch chan int) { for i := 0; i < 5; i++ { ch <- i // 发送数据到channel } close(ch) // 关闭channel } func main() { ch := make(chan int) // 创建一个channel go process(ch) // 启动一个Goroutine来处理数据 for { value, ok := <-ch // 从channel接收数据 if !ok { break } fmt.Println("Received:", value) } }
In this code, the process
function uses ch i<code> to the channel. In the
main function, we receive data from the channel through
value, ok := . When the channel is closed, the value of ok will be
false. We can check whether there is still data to receive by checking the value of
ok.
In concurrent programming, synchronization operations are very important. Golang provides a variety of synchronization methods, such as using
WaitGroup,
Mutex, etc. in the
sync package. These synchronization mechanisms can help us control the execution order of concurrent tasks and avoid problems such as data competition.
sync.WaitGroup to implement concurrent tasks:
package main import ( "fmt" "sync" "time" ) func task(id int, wg *sync.WaitGroup) { defer wg.Done() fmt.Println("Task", id, "is running...") time.Sleep(time.Second) fmt.Println("Task", id, "is done.") } func main() { var wg sync.WaitGroup for i := 1; i <= 3; i++ { wg.Add(1) go task(i, &wg) } wg.Wait() fmt.Println("All tasks are done.") }In this code, we create a
sync.WaitGroupExample
wg to manage the execution of concurrent tasks. In the
task function, we use
wg.Done() to indicate task completion, and pass
wg.Wait()## in the main
function #Wait for all tasks to be completed. When you run the program, you can see that the tasks are executed concurrently in order, and "All tasks are done." is printed after waiting for all tasks to be completed. Summary:
The above is the detailed content of Learn and master Golang's concurrent programming technology from scratch. For more information, please follow other related articles on the PHP Chinese website!
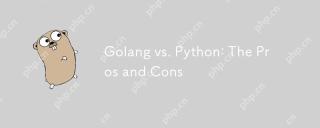
Golangisidealforbuildingscalablesystemsduetoitsefficiencyandconcurrency,whilePythonexcelsinquickscriptinganddataanalysisduetoitssimplicityandvastecosystem.Golang'sdesignencouragesclean,readablecodeanditsgoroutinesenableefficientconcurrentoperations,t
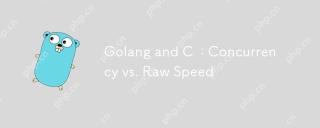
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
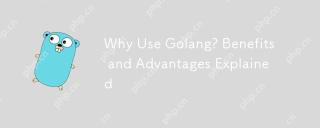
Reasons for choosing Golang include: 1) high concurrency performance, 2) static type system, 3) garbage collection mechanism, 4) rich standard libraries and ecosystems, which make it an ideal choice for developing efficient and reliable software.
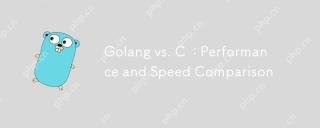
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
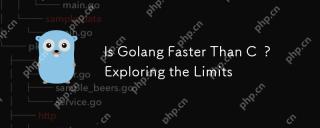
Golang performs better in compilation time and concurrent processing, while C has more advantages in running speed and memory management. 1.Golang has fast compilation speed and is suitable for rapid development. 2.C runs fast and is suitable for performance-critical applications. 3. Golang is simple and efficient in concurrent processing, suitable for concurrent programming. 4.C Manual memory management provides higher performance, but increases development complexity.
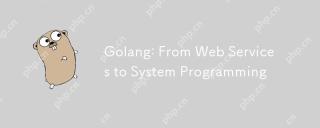
Golang's application in web services and system programming is mainly reflected in its simplicity, efficiency and concurrency. 1) In web services, Golang supports the creation of high-performance web applications and APIs through powerful HTTP libraries and concurrent processing capabilities. 2) In system programming, Golang uses features close to hardware and compatibility with C language to be suitable for operating system development and embedded systems.
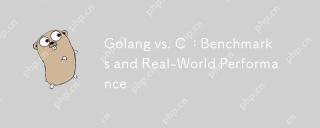
Golang and C have their own advantages and disadvantages in performance comparison: 1. Golang is suitable for high concurrency and rapid development, but garbage collection may affect performance; 2.C provides higher performance and hardware control, but has high development complexity. When making a choice, you need to consider project requirements and team skills in a comprehensive way.
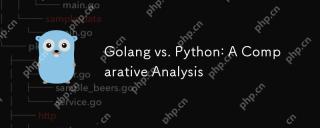
Golang is suitable for high-performance and concurrent programming scenarios, while Python is suitable for rapid development and data processing. 1.Golang emphasizes simplicity and efficiency, and is suitable for back-end services and microservices. 2. Python is known for its concise syntax and rich libraries, suitable for data science and machine learning.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
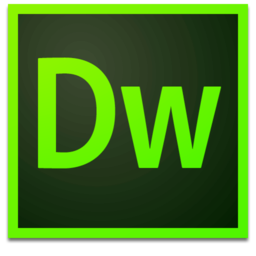
Dreamweaver Mac version
Visual web development tools
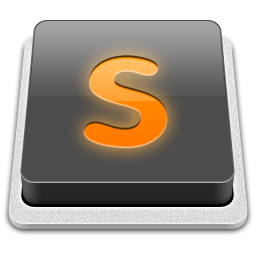
SublimeText3 Mac version
God-level code editing software (SublimeText3)

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
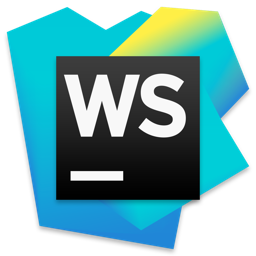
WebStorm Mac version
Useful JavaScript development tools