Practical application cases of Golang generic programming, specific code examples are required
Introduction:
With the development of cloud computing, big data and artificial intelligence, The challenges faced by software development engineers are increasing day by day. The generic features of programming languages can provide more efficient and flexible solutions, and Golang, as a modern programming language, has finally introduced support for generic programming in version 1.18. In this article, we will share some practical application cases of Golang generic programming and provide specific code examples.
- Simplify the implementation of containers
In traditional Golang programming, we usually need to write different implementation codes for different types of containers (such as slices, linked lists, dictionaries). Generic programming can make it easier for us to implement these containers, thereby reducing code duplication.
Sample code:
package main import "fmt" type Stack[T any] []T func (s *Stack[T]) Push(value T) { *s = append(*s, value) } func (s *Stack[T]) Pop() T { top := (*s)[len(*s)-1] *s = (*s)[:len(*s)-1] return top } func main() { stack := Stack[int]{} stack.Push(1) stack.Push(2) stack.Push(3) fmt.Println(stack.Pop()) // 输出:3 fmt.Println(stack.Pop()) // 输出:2 fmt.Println(stack.Pop()) // 输出:1 }
In the above code, we define a generic Stack container, where T represents any type. By using generic features, we can handle different types of data in the same implementation and reduce the writing of duplicate code.
- Improve the versatility of the algorithm
Generic programming can also improve the versatility of the algorithm so that it can be applied to different types of data. This is especially useful for some common algorithms, such as sorting algorithms and search algorithms.
Sample code:
package main import "fmt" type Comparable[T any] interface { LessThan(other T) bool } type Sortable[T Comparable[T]] []T func (s Sortable[T]) Sort() { for i := 0; i < len(s)-1; i++ { for j := i + 1; j < len(s); j++ { if s[j].LessThan(s[i]) { s[i], s[j] = s[j], s[i] } } } } type Person struct { Name string Age int } func (p Person) LessThan(other Person) bool { return p.Age < other.Age } func main() { people := Sortable[Person]{ {Name: "Alice", Age: 30}, {Name: "Bob", Age: 25}, {Name: "Charlie", Age: 35}, } people.Sort() fmt.Println(people) // 输出:[{Bob 25} {Alice 30} {Charlie 35}] }
In the above code, we define a Comparable interface, in which the LessThan method is used to compare two objects. Then, we defined a Sortable container, where T is the implementation of Comparable. Through such a definition, we can pass different types of data to Sortable's Sort method and implement custom sorting.
Conclusion:
The practical application cases of Golang generic programming cover the implementation of containers and the improvement of algorithm versatility. By using Golang's generic features, we can write common code more conveniently, improving development efficiency and code reusability. The above examples are only part of the applications of generic programming. In actual projects in the future, we can also benefit from the support of Golang's generic programming features in more problems.
The above is the detailed content of Practical cases of golang generic programming. For more information, please follow other related articles on the PHP Chinese website!
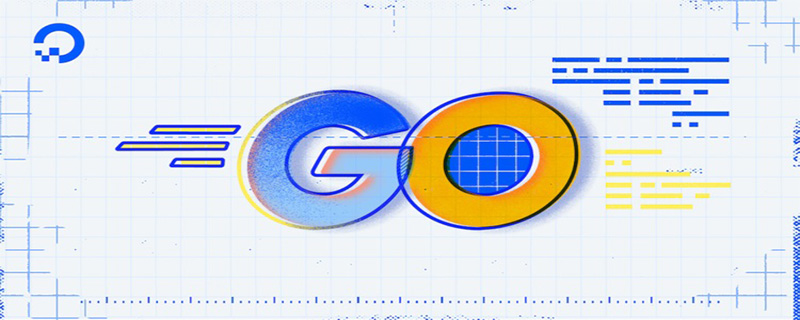
go语言有缩进。在go语言中,缩进直接使用gofmt工具格式化即可(gofmt使用tab进行缩进);gofmt工具会以标准样式的缩进和垂直对齐方式对源代码进行格式化,甚至必要情况下注释也会重新格式化。
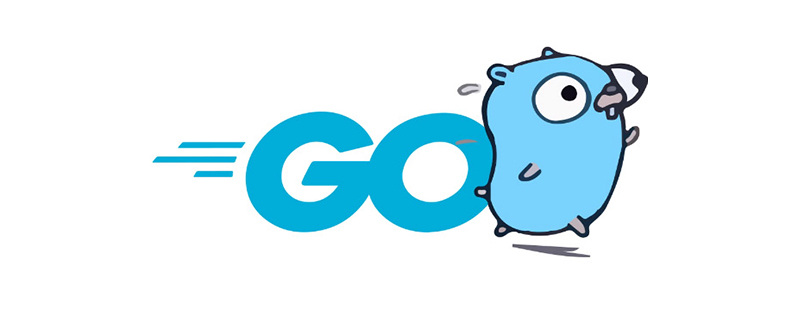
本篇文章带大家了解一下golang 的几种常用的基本数据类型,如整型,浮点型,字符,字符串,布尔型等,并介绍了一些常用的类型转换操作。
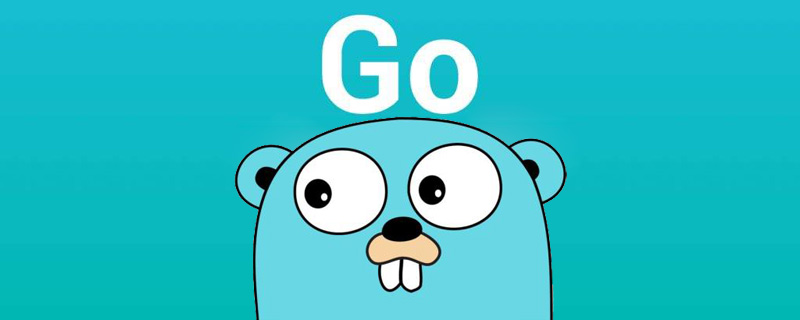
go语言叫go的原因:想表达这门语言的运行速度、开发速度、学习速度(develop)都像gopher一样快。gopher是一种生活在加拿大的小动物,go的吉祥物就是这个小动物,它的中文名叫做囊地鼠,它们最大的特点就是挖洞速度特别快,当然可能不止是挖洞啦。
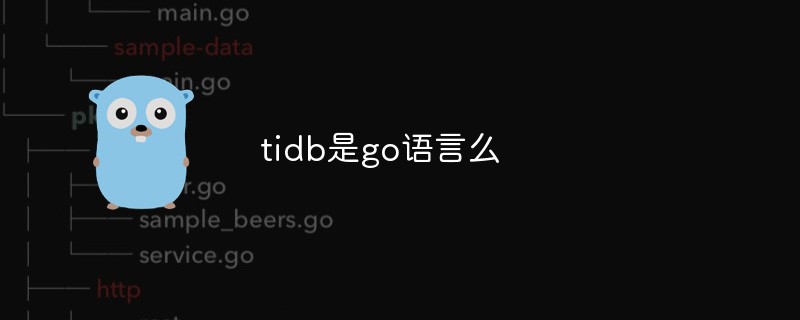
是,TiDB采用go语言编写。TiDB是一个分布式NewSQL数据库;它支持水平弹性扩展、ACID事务、标准SQL、MySQL语法和MySQL协议,具有数据强一致的高可用特性。TiDB架构中的PD储存了集群的元信息,如key在哪个TiKV节点;PD还负责集群的负载均衡以及数据分片等。PD通过内嵌etcd来支持数据分布和容错;PD采用go语言编写。
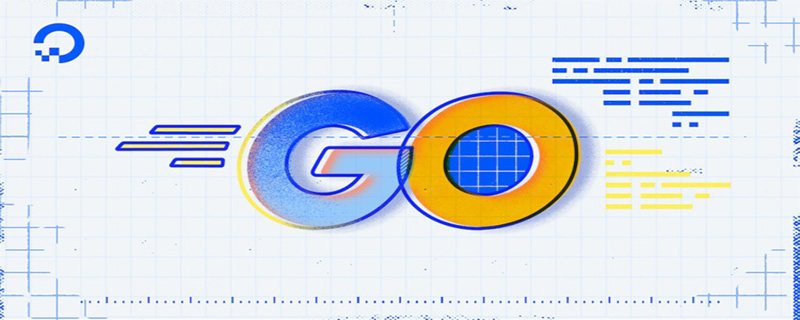
在写 Go 的过程中经常对比这两种语言的特性,踩了不少坑,也发现了不少有意思的地方,下面本篇就来聊聊 Go 自带的 HttpClient 的超时机制,希望对大家有所帮助。
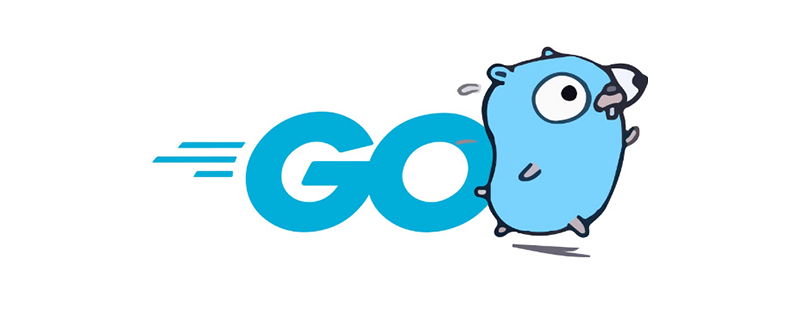
go语言需要编译。Go语言是编译型的静态语言,是一门需要编译才能运行的编程语言,也就说Go语言程序在运行之前需要通过编译器生成二进制机器码(二进制的可执行文件),随后二进制文件才能在目标机器上运行。
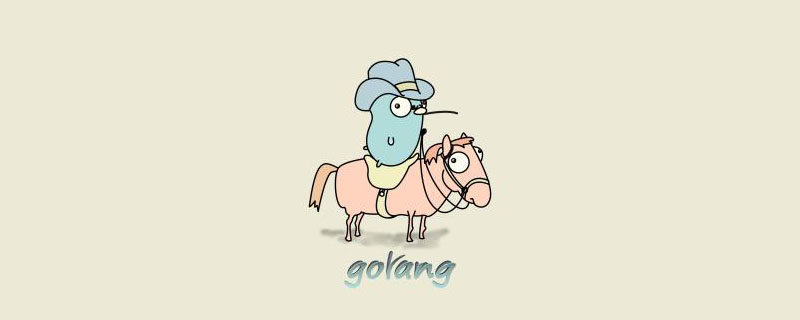
删除map元素的两种方法:1、使用delete()函数从map中删除指定键值对,语法“delete(map, 键名)”;2、重新创建一个新的map对象,可以清空map中的所有元素,语法“var mapname map[keytype]valuetype”。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
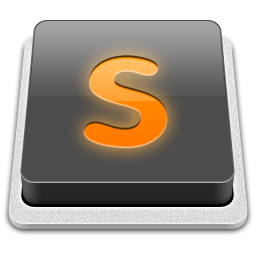
SublimeText3 Mac version
God-level code editing software (SublimeText3)

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

Zend Studio 13.0.1
Powerful PHP integrated development environment
