编码器是一种常用的位置传感器,可以用来测量旋转和线性运动的位移,并将其转换为数字信号。编码器的绝对定位功能可以让我们精确地知道物体的位置,因此在许多领域都有广泛的应用,比如机器人、汽车、医疗仪器等等。
理解编码器绝对定位的方法有很多种,其中比较常见的有以下几种:
- 二进制编码方法
二进制编码方法是一种将物理运动转换为数字信号的方式。编码器通过一个位置传感器来检测物体是否移动,并根据物体运动的位置改变其输出的数字编码。每个数字编码对应的是一个唯一的物理位置,因此我们可以通过读取编码器的输出来确定物体的位置。
下面是一个用Arduino实现的二进制编码器示例代码:
const int encoderPinA = 2; const int encoderPinB = 3; volatile int encoderPos = 0; volatile bool aSet = false; volatile bool bSet = false; void setup() { pinMode(encoderPinA, INPUT); pinMode(encoderPinB, INPUT); attachInterrupt(digitalPinToInterrupt(encoderPinA), updateEncoderA, CHANGE); attachInterrupt(digitalPinToInterrupt(encoderPinB), updateEncoderB, CHANGE); } void loop() { // 读取编码器当前位置 int newPos = encoderPos; Serial.println(newPos); } void updateEncoderA() { aSet = digitalRead(encoderPinA); if (aSet && !bSet) { encoderPos++; } else if (!aSet && bSet) { encoderPos--; } bSet = digitalRead(encoderPinB); } void updateEncoderB() { bSet = digitalRead(encoderPinB); if (bSet && !aSet) { encoderPos--; } else if (!bSet && aSet) { encoderPos++; } aSet = digitalRead(encoderPinA); }
- 格雷码编码方法
格雷码是一种二进制编码的变体,它的优点在于只有一个位置的变化会导致一个编码位的变化。格雷码编码器的输出与二进制编码器类似,但在对编码进行解码之前需要将其转换为二进制表示。这可以通过查找一个转换表来完成,或使用特定的解码器芯片来自动完成转换。
下面是一个使用Shift Register 74HC595实现的格雷码编码器示例代码:
const int encoderPinClock = 4; const int encoderPinData = 5; const int encoderPinLatch = 6; unsigned int encoderValue = 0; void setup() { pinMode(encoderPinClock, OUTPUT); pinMode(encoderPinData, OUTPUT); pinMode(encoderPinLatch, OUTPUT); } void loop() { // 读取编码器当前位置 unsigned int newPos = 0; for (int i = 0; i < 16; i++) { digitalWrite(encoderPinLatch, LOW); shiftOut(encoderPinData, encoderPinClock, MSBFIRST, 1 << i); digitalWrite(encoderPinLatch, HIGH); delayMicroseconds(10); newPos |= digitalRead(encoderPinData) << i; } encoderValue = newPos; Serial.println(encoderValue); }
- PWM编码方法
PWM编码方法利用了脉冲宽度调制的原理,将编码器的输出信号转换为脉冲信号。每个脉冲宽度对应一个位置,因此我们可以通过读取脉冲宽度来确定位置。
下面是一个使用ESP32的PWM模块实现的PWM编码器示例代码:
const int encoderPin = 5; volatile int encoderPos = 0; volatile unsigned long lastPulseTime = 0; void IRAM_ATTR pulseHandler() { unsigned long pulseTime = micros(); if (pulseTime - lastPulseTime > 10) { if (digitalRead(encoderPin) == HIGH) { encoderPos--; } else { encoderPos++; } lastPulseTime = pulseTime; } } void setup() { pinMode(encoderPin, INPUT); attachInterrupt(encoderPin, pulseHandler, CHANGE); ledcSetup(0, 5000, 8); ledcAttachPin(encoderPin, 0); } void loop() { // 读取编码器当前位置 int newPos = map(ledcRead(0), 0, 255, -100, 100); encoderPos = newPos; Serial.println(encoderPos); }
总结
以上是三种常见的编码器绝对定位方法的代码示例。通过理解编码器的工作原理,我们可以更好地了解如何应用它来实现精确定位,从而在机器人、汽车、医疗仪器等领域提高生产效率和质量。
The above is the detailed content of Different ways to understand absolute positioning of encoders. For more information, please follow other related articles on the PHP Chinese website!

The function of HTML is to define the structure and content of a web page, and its purpose is to provide a standardized way to display information. 1) HTML organizes various parts of the web page through tags and attributes, such as titles and paragraphs. 2) It supports the separation of content and performance and improves maintenance efficiency. 3) HTML is extensible, allowing custom tags to enhance SEO.

The future trends of HTML are semantics and web components, the future trends of CSS are CSS-in-JS and CSSHoudini, and the future trends of JavaScript are WebAssembly and Serverless. 1. HTML semantics improve accessibility and SEO effects, and Web components improve development efficiency, but attention should be paid to browser compatibility. 2. CSS-in-JS enhances style management flexibility but may increase file size. CSSHoudini allows direct operation of CSS rendering. 3.WebAssembly optimizes browser application performance but has a steep learning curve, and Serverless simplifies development but requires optimization of cold start problems.

The roles of HTML, CSS and JavaScript in web development are: 1. HTML defines the web page structure, 2. CSS controls the web page style, and 3. JavaScript adds dynamic behavior. Together, they build the framework, aesthetics and interactivity of modern websites.

The future of HTML is full of infinite possibilities. 1) New features and standards will include more semantic tags and the popularity of WebComponents. 2) The web design trend will continue to develop towards responsive and accessible design. 3) Performance optimization will improve the user experience through responsive image loading and lazy loading technologies.

The roles of HTML, CSS and JavaScript in web development are: HTML is responsible for content structure, CSS is responsible for style, and JavaScript is responsible for dynamic behavior. 1. HTML defines the web page structure and content through tags to ensure semantics. 2. CSS controls the web page style through selectors and attributes to make it beautiful and easy to read. 3. JavaScript controls web page behavior through scripts to achieve dynamic and interactive functions.

HTMLisnotaprogramminglanguage;itisamarkuplanguage.1)HTMLstructuresandformatswebcontentusingtags.2)ItworkswithCSSforstylingandJavaScriptforinteractivity,enhancingwebdevelopment.

HTML is the cornerstone of building web page structure. 1. HTML defines the content structure and semantics, and uses, etc. tags. 2. Provide semantic markers, such as, etc., to improve SEO effect. 3. To realize user interaction through tags, pay attention to form verification. 4. Use advanced elements such as, combined with JavaScript to achieve dynamic effects. 5. Common errors include unclosed labels and unquoted attribute values, and verification tools are required. 6. Optimization strategies include reducing HTTP requests, compressing HTML, using semantic tags, etc.

HTML is a language used to build web pages, defining web page structure and content through tags and attributes. 1) HTML organizes document structure through tags, such as,. 2) The browser parses HTML to build the DOM and renders the web page. 3) New features of HTML5, such as, enhance multimedia functions. 4) Common errors include unclosed labels and unquoted attribute values. 5) Optimization suggestions include using semantic tags and reducing file size.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
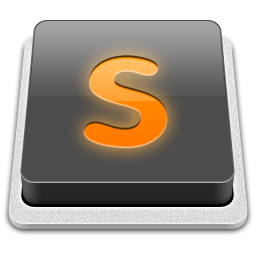
SublimeText3 Mac version
God-level code editing software (SublimeText3)

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

Atom editor mac version download
The most popular open source editor