


In-depth understanding of Java data structures: the key to improving programming skills
Full analysis of Java data structures: Understanding these data structures to improve your programming skills requires specific code examples
Introduction:
In computer science, data structures Refers to the way data is organized and stored in computer memory. In programming, understanding different data structures is crucial to optimizing algorithms and making programs more efficient. This article will introduce several common Java data structures and provide specific code examples to help readers understand and apply these data structures.
1. Array
An array is a linear data structure that can store multiple elements in a single variable. Each element is accessed by index, which starts from zero. Arrays in Java can store elements of the same type.
The following is a sample code showing how to declare, initialize and access elements in an array:
int[] myArray = new int[5]; // 声明一个长度为5的整数数组 myArray[0] = 10; myArray[1] = 20; myArray[2] = 30; myArray[3] = 40; myArray[4] = 50; System.out.println(myArray[0]); // 输出:10 System.out.println(myArray[4]); // 输出:50
2. Linked List (LinkedList)
The linked list is a dynamic data structure that can be inserted at runtime and delete elements. A linked list consists of nodes, each node contains a data element and a reference to the next node.
The following is a sample code that shows how to create, add and delete nodes in a linked list:
class Node { int data; Node next; public Node(int data) { this.data = data; this.next = null; } } class LinkedList { Node head; public void addNode(int data) { Node newNode = new Node(data); if (head == null) { head = newNode; } else { Node temp = head; while (temp.next != null) { temp = temp.next; } temp.next = newNode; } } public void deleteNode(int data) { Node temp = head; Node prev = null; if (temp != null && temp.data == data) { head = temp.next; return; } while (temp != null && temp.data != data) { prev = temp; temp = temp.next; } if (temp == null) { return; } prev.next = temp.next; } } public class Main { public static void main(String[] args) { LinkedList linkedList = new LinkedList(); linkedList.addNode(10); linkedList.addNode(20); linkedList.addNode(30); linkedList.addNode(40); linkedList.deleteNode(20); Node temp = linkedList.head; while (temp != null) { System.out.println(temp.data); temp = temp.next; } } }
3. Stack
The stack is a last-in-first-out (LIFO) data structure . It can add and remove elements using push and pop operations.
The following is a sample code showing how to use the stack:
import java.util.Stack; public class Main { public static void main(String[] args) { Stack<Integer> stack = new Stack<>(); stack.push(10); stack.push(20); stack.push(30); System.out.println(stack.pop()); // 输出:30 System.out.println(stack.peek()); // 输出:20 } }
4. Queue (Queue)
The queue is a first-in, first-out (FIFO) data structure. It can add and remove elements by using add (enqueue) and poll (dequeue) operations.
The following is a sample code showing how to use a queue:
import java.util.LinkedList; import java.util.Queue; public class Main { public static void main(String[] args) { Queue<Integer> queue = new LinkedList<>(); queue.add(10); queue.add(20); queue.add(30); System.out.println(queue.poll()); // 输出:10 System.out.println(queue.peek()); // 输出:20 } }
5. Hash table (HashMap)
The hash table is a data structure of key-value pairs that uses a hash function to Data is stored in array. Keys provide quick access and update of values.
The following is a sample code showing how to use a hash table:
import java.util.HashMap; public class Main { public static void main(String[] args) { HashMap<String, Integer> hashMap = new HashMap<>(); hashMap.put("apple", 10); hashMap.put("banana", 20); hashMap.put("orange", 30); System.out.println(hashMap.get("apple")); // 输出:10 System.out.println(hashMap.containsKey("banana")); // 输出:true } }
6. Tree (Tree)
Tree is a non-linear data structure consisting of nodes and edges. Each node of the tree can have multiple child nodes.
The following is a sample code showing how to create and traverse a tree:
class Node { int data; Node left, right; public Node(int data) { this.data = data; left = right = null; } } class BinaryTree { Node root; public BinaryTree() { root = null; } public void inorderTraversal(Node node) { if (node == null) { return; } inorderTraversal(node.left); System.out.println(node.data); inorderTraversal(node.right); } } public class Main { public static void main(String[] args) { BinaryTree tree = new BinaryTree(); tree.root = new Node(1); tree.root.left = new Node(2); tree.root.right = new Node(3); tree.root.left.left = new Node(4); tree.inorderTraversal(tree.root); } }
Summary:
This article introduces several common Java data structures and provides specific code examples to help readers understand and apply these data structures. By becoming proficient in these data structures, we can better optimize algorithms and improve program efficiency. Continuously learning and applying data structures will help improve your programming skills.
The above is the detailed content of In-depth understanding of Java data structures: the key to improving programming skills. For more information, please follow other related articles on the PHP Chinese website!
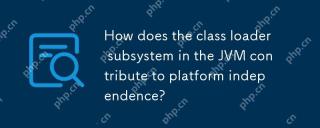
The class loader ensures the consistency and compatibility of Java programs on different platforms through unified class file format, dynamic loading, parent delegation model and platform-independent bytecode, and achieves platform independence.
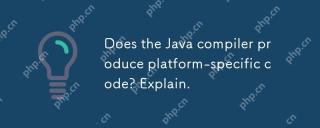
The code generated by the Java compiler is platform-independent, but the code that is ultimately executed is platform-specific. 1. Java source code is compiled into platform-independent bytecode. 2. The JVM converts bytecode into machine code for a specific platform, ensuring cross-platform operation but performance may be different.
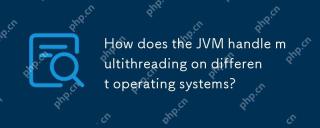
Multithreading is important in modern programming because it can improve program responsiveness and resource utilization and handle complex concurrent tasks. JVM ensures the consistency and efficiency of multithreads on different operating systems through thread mapping, scheduling mechanism and synchronization lock mechanism.
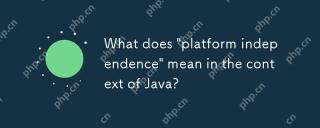
Java's platform independence means that the code written can run on any platform with JVM installed without modification. 1) Java source code is compiled into bytecode, 2) Bytecode is interpreted and executed by the JVM, 3) The JVM provides memory management and garbage collection functions to ensure that the program runs on different operating systems.
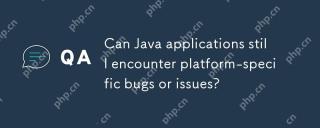
Javaapplicationscanindeedencounterplatform-specificissuesdespitetheJVM'sabstraction.Reasonsinclude:1)Nativecodeandlibraries,2)Operatingsystemdifferences,3)JVMimplementationvariations,and4)Hardwaredependencies.Tomitigatethese,developersshould:1)Conduc
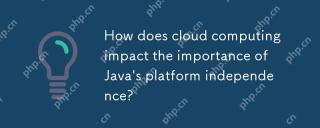
Cloud computing significantly improves Java's platform independence. 1) Java code is compiled into bytecode and executed by the JVM on different operating systems to ensure cross-platform operation. 2) Use Docker and Kubernetes to deploy Java applications to improve portability and scalability.
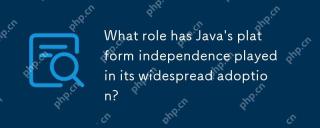
Java'splatformindependenceallowsdeveloperstowritecodeonceandrunitonanydeviceorOSwithaJVM.Thisisachievedthroughcompilingtobytecode,whichtheJVMinterpretsorcompilesatruntime.ThisfeaturehassignificantlyboostedJava'sadoptionduetocross-platformdeployment,s
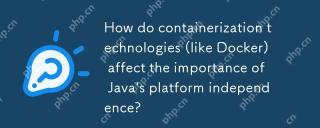
Containerization technologies such as Docker enhance rather than replace Java's platform independence. 1) Ensure consistency across environments, 2) Manage dependencies, including specific JVM versions, 3) Simplify the deployment process to make Java applications more adaptable and manageable.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
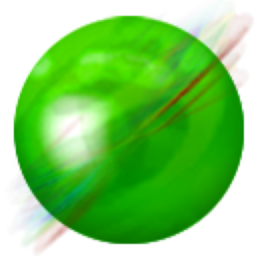
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
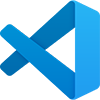
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

SublimeText3 Linux new version
SublimeText3 Linux latest version