


Use php to develop Websocket to implement real-time product recommendation function
Websocket is a full-duplex communication protocol that can achieve real-time communication between the browser and the server. In e-commerce websites, Websocket can be used to implement real-time product recommendation functions to help users better find the products they need.
This article will introduce how to use PHP to develop Websocket to implement real-time product recommendation function, and provide specific code examples.
1. Requirements
Before starting development, we need to meet the following requirements:
- PHP version: 5.3 or above
- Operating system: Linux, Windows, Mac, etc.
- Extension: swoole extension (https://www.swoole.com/) 4.3.0 or above
2. Install the swoole extension
First, we need to install the swoole extension. swoole provides basic classes and event-driven models for developing Websocket, which greatly simplifies the development of Websocket.
In a Linux environment, you can install the swoole extension through the following command:
pecl install swoole
In a Windows environment, you can install it from the swoole official website (https://windows.php.net/downloads/pecl/ releases/swoole/) Download the corresponding version of the swoole extension, extract it to the php extension directory, and add the following lines to the php.ini file:
extension=swoole.so
3. Develop Websocket
Next, we start developing Websocket. First, create a websocket.php file to handle Websocket connections.
<?php // 创建Websocket服务器 $server = new swoole_websocket_server("0.0.0.0", 9501); // 监听WebSocket连接打开事件 $server->on('open', function (swoole_websocket_server $server, $request) { echo "WebSocket连接建立成功! "; }); // 监听WebSocket消息事件 $server->on('message', function (swoole_websocket_server $server, $frame) { echo "WebSocket收到消息:{$frame->data} "; // 处理推荐商品逻辑 // ... // 返回推荐商品列表 $server->push($frame->fd, json_encode([ ['name' => '商品1', 'price' => 10], ['name' => '商品2', 'price' => 20], ['name' => '商品3', 'price' => 30], ])); }); // 监听WebSocket连接关闭事件 $server->on('close', function ($server, $fd) { echo "WebSocket连接关闭! "; }); // 启动Websocket服务器 $server->start();
The above code creates a Websocket server, monitors connection requests from the client, and triggers corresponding callback functions when events such as successful connection establishment, message reception, and connection closure occur.
In the message event received, we can write the logic of product recommendation and return the recommendation results to the client.
4. Client uses Websocket
In the client, we need to use JavaScript to create a Websocket connection and send a message to obtain recommended products.
// 创建Websocket连接 var ws = new WebSocket("ws://127.0.0.1:9501"); // 监听Websocket连接打开事件 ws.onopen = function() { console.log("Websocket连接建立成功!"); // 发送消息 ws.send("Hello, Server!"); }; // 监听Websocket消息事件 ws.onmessage = function(event) { var data = JSON.parse(event.data); console.log("推荐商品列表:", data); }; // 监听Websocket连接关闭事件 ws.onclose = function() { console.log("Websocket连接关闭!"); };
In the above code, we create a WebSocket connection, listen for connection opening, message and connection closing events, and send messages after the connection is successfully established to obtain recommended products.
5. Summary
This article introduces how to use PHP to develop Websocket to realize the real-time product recommendation function, and provides specific code implementation.
Through Websocket, we can achieve real-time communication and data exchange to provide better services for users of e-commerce websites. At the same time, Websocket can also be used in other fields, such as online games, video conferencing, etc.
The above is the detailed content of Use php to develop Websocket to implement real-time product recommendation function. For more information, please follow other related articles on the PHP Chinese website!
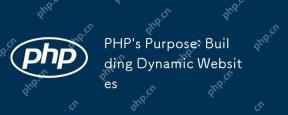
PHP is used to build dynamic websites, and its core functions include: 1. Generate dynamic content and generate web pages in real time by connecting with the database; 2. Process user interaction and form submissions, verify inputs and respond to operations; 3. Manage sessions and user authentication to provide a personalized experience; 4. Optimize performance and follow best practices to improve website efficiency and security.

PHP uses MySQLi and PDO extensions to interact in database operations and server-side logic processing, and processes server-side logic through functions such as session management. 1) Use MySQLi or PDO to connect to the database and execute SQL queries. 2) Handle HTTP requests and user status through session management and other functions. 3) Use transactions to ensure the atomicity of database operations. 4) Prevent SQL injection, use exception handling and closing connections for debugging. 5) Optimize performance through indexing and cache, write highly readable code and perform error handling.
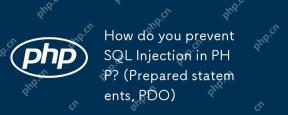
Using preprocessing statements and PDO in PHP can effectively prevent SQL injection attacks. 1) Use PDO to connect to the database and set the error mode. 2) Create preprocessing statements through the prepare method and pass data using placeholders and execute methods. 3) Process query results and ensure the security and performance of the code.
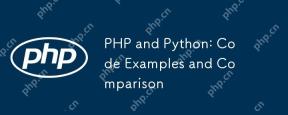
PHP and Python have their own advantages and disadvantages, and the choice depends on project needs and personal preferences. 1.PHP is suitable for rapid development and maintenance of large-scale web applications. 2. Python dominates the field of data science and machine learning.
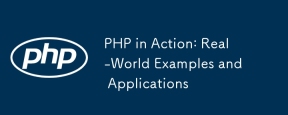
PHP is widely used in e-commerce, content management systems and API development. 1) E-commerce: used for shopping cart function and payment processing. 2) Content management system: used for dynamic content generation and user management. 3) API development: used for RESTful API development and API security. Through performance optimization and best practices, the efficiency and maintainability of PHP applications are improved.
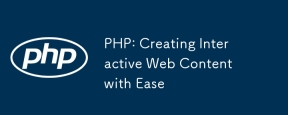
PHP makes it easy to create interactive web content. 1) Dynamically generate content by embedding HTML and display it in real time based on user input or database data. 2) Process form submission and generate dynamic output to ensure that htmlspecialchars is used to prevent XSS. 3) Use MySQL to create a user registration system, and use password_hash and preprocessing statements to enhance security. Mastering these techniques will improve the efficiency of web development.
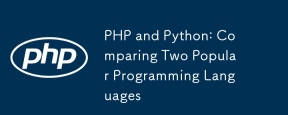
PHP and Python each have their own advantages, and choose according to project requirements. 1.PHP is suitable for web development, especially for rapid development and maintenance of websites. 2. Python is suitable for data science, machine learning and artificial intelligence, with concise syntax and suitable for beginners.
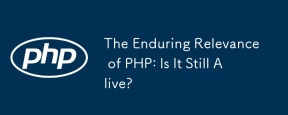
PHP is still dynamic and still occupies an important position in the field of modern programming. 1) PHP's simplicity and powerful community support make it widely used in web development; 2) Its flexibility and stability make it outstanding in handling web forms, database operations and file processing; 3) PHP is constantly evolving and optimizing, suitable for beginners and experienced developers.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
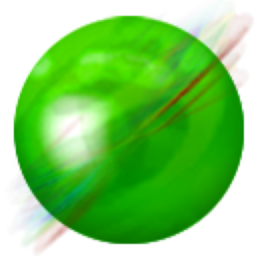
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
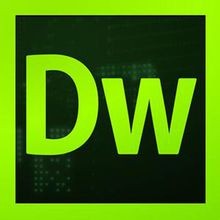
Dreamweaver CS6
Visual web development tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

SublimeText3 Linux new version
SublimeText3 Linux latest version