


How to use WebSocket and JavaScript to achieve online whiteboard collaboration
How to use WebSocket and JavaScript to achieve online whiteboard collaboration
Part 1: Overview
Online whiteboard collaboration refers to multiple users connecting through the network in real time Carry out drawing, annotation and other operations on the same whiteboard, and be able to see the operations of other users. In this article, we will introduce how to use WebSocket and JavaScript to implement online whiteboard collaboration.
Part 2: Introduction to WebSocket
WebSocket is a network protocol for two-way communication between the client and the server. Compared with the traditional HTTP protocol, WebSocket has lower latency and higher real-time performance, which is very suitable for realizing real-time collaboration functions.
Part 3: Establishing a WebSocket connection
In JavaScript, we can use the WebSocket API to establish a WebSocket connection with the server. The following is a simple example:
let socket = new WebSocket("ws://example.com/socket");
In this example, we use new WebSocket
to create a WebSocket object and pass in the server address. The address here starts with ws://
, which means the WebSocket protocol is used.
Part 4: Handling WebSocket Events
The WebSocket object has some events, and we can handle these events by registering event listeners. The following are examples of some commonly used events and their processing methods:
// 建立连接 socket.onopen = function(event) { console.log("WebSocket连接已建立"); }; // 收到消息 socket.onmessage = function(event) { let message = event.data; // 接收到的消息数据 console.log("收到消息:" + message); }; // 连接关闭 socket.onclose = function(event) { console.log("WebSocket连接已关闭"); }; // 发生错误 socket.onerror = function(event) { console.error("WebSocket错误"); };
In these event processing functions, we can handle different events accordingly. For example, in the event processing function that receives the message, we can parse the received message and process it.
Part 5: Implement whiteboard collaboration
When implementing the whiteboard collaboration function, we can define some protocols and message formats. For example, we can agree to use messages in JSON format and encapsulate drawing and annotation instructions into corresponding messages.
The following is a simple whiteboard collaboration example:
// 接收到消息时的处理函数 socket.onmessage = function(event) { let message = JSON.parse(event.data); // 根据消息类型进行处理 switch(message.type) { case "draw": drawOnWhiteboard(message.data); // 绘画指令 break; case "annotate": annotateOnWhiteboard(message.data); // 标注指令 break; // 其他类型的消息处理... } }; // 发送绘画指令 function sendDrawCommand(data) { let message = { type: "draw", data: data }; socket.send(JSON.stringify(message)); } // 发送标注指令 function sendAnnotateCommand(data) { let message = { type: "annotate", data: data }; socket.send(JSON.stringify(message)); }
In this example, we define the drawOnWhiteboard
and annotateOnWhiteboard
functions to handle drawing and Mark the command and send the message of the corresponding command through the sendDrawCommand
and sendAnnotateCommand
functions.
Part 6: Summary
By using WebSocket and JavaScript, we can realize the function of online whiteboard collaboration. WebSocket provides two-way communication capabilities, and JavaScript can easily handle WebSocket events and send messages. By properly designing protocols and message formats, we can implement whiteboard applications for real-time collaboration. I hope this article will help you understand how to use WebSocket and JavaScript to achieve online whiteboard collaboration.
The above is the detailed content of How to use WebSocket and JavaScript to achieve online whiteboard collaboration. For more information, please follow other related articles on the PHP Chinese website!
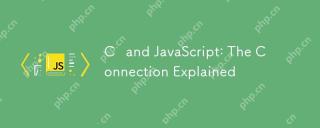
C and JavaScript achieve interoperability through WebAssembly. 1) C code is compiled into WebAssembly module and introduced into JavaScript environment to enhance computing power. 2) In game development, C handles physics engines and graphics rendering, and JavaScript is responsible for game logic and user interface.
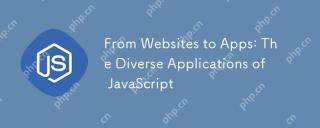
JavaScript is widely used in websites, mobile applications, desktop applications and server-side programming. 1) In website development, JavaScript operates DOM together with HTML and CSS to achieve dynamic effects and supports frameworks such as jQuery and React. 2) Through ReactNative and Ionic, JavaScript is used to develop cross-platform mobile applications. 3) The Electron framework enables JavaScript to build desktop applications. 4) Node.js allows JavaScript to run on the server side and supports high concurrent requests.
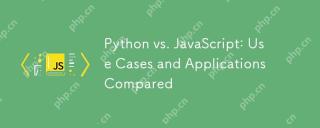
Python is more suitable for data science and automation, while JavaScript is more suitable for front-end and full-stack development. 1. Python performs well in data science and machine learning, using libraries such as NumPy and Pandas for data processing and modeling. 2. Python is concise and efficient in automation and scripting. 3. JavaScript is indispensable in front-end development and is used to build dynamic web pages and single-page applications. 4. JavaScript plays a role in back-end development through Node.js and supports full-stack development.
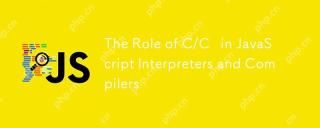
C and C play a vital role in the JavaScript engine, mainly used to implement interpreters and JIT compilers. 1) C is used to parse JavaScript source code and generate an abstract syntax tree. 2) C is responsible for generating and executing bytecode. 3) C implements the JIT compiler, optimizes and compiles hot-spot code at runtime, and significantly improves the execution efficiency of JavaScript.
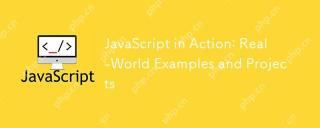
JavaScript's application in the real world includes front-end and back-end development. 1) Display front-end applications by building a TODO list application, involving DOM operations and event processing. 2) Build RESTfulAPI through Node.js and Express to demonstrate back-end applications.
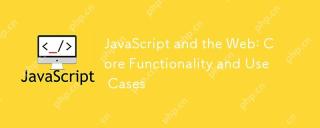
The main uses of JavaScript in web development include client interaction, form verification and asynchronous communication. 1) Dynamic content update and user interaction through DOM operations; 2) Client verification is carried out before the user submits data to improve the user experience; 3) Refreshless communication with the server is achieved through AJAX technology.
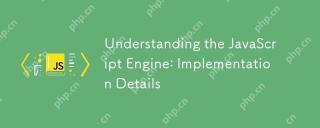
Understanding how JavaScript engine works internally is important to developers because it helps write more efficient code and understand performance bottlenecks and optimization strategies. 1) The engine's workflow includes three stages: parsing, compiling and execution; 2) During the execution process, the engine will perform dynamic optimization, such as inline cache and hidden classes; 3) Best practices include avoiding global variables, optimizing loops, using const and lets, and avoiding excessive use of closures.

Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor
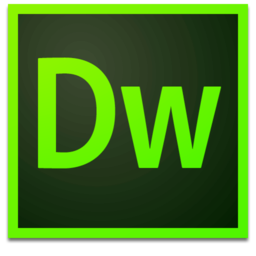
Dreamweaver Mac version
Visual web development tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
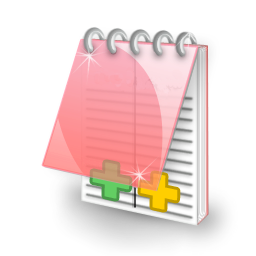
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function