


How to use JavaScript and WebSocket to implement a real-time online questionnaire survey system
Introduction:
With the continuous development of the Internet, more and more questionnaires have begun to migrate Go online. In order to obtain user feedback in real time, a real-time online questionnaire system becomes a needed tool. This article will introduce how to use JavaScript and WebSocket to implement a simple real-time online questionnaire system, and give specific code examples.
1. Technology selection
When implementing the real-time online questionnaire survey system, we chose to use JavaScript and WebSocket as the key technologies for implementation. JavaScript is a cross-platform, object-oriented scripting language that can be used for browser-side script development. WebSocket is a protocol for full-duplex communication on a single TCP connection, which enables the server to actively push data to the client.
2. System Architecture
The architecture of the real-time online questionnaire survey system is mainly divided into two parts: front-end and back-end.
1. Front-end part
The front-end part mainly includes user interface and JavaScript code. The user interface is responsible for displaying the questionnaire and receiving user feedback, while the JavaScript code is responsible for establishing a WebSocket connection with the backend and receiving the questionnaire results in real time.
2. Backend part
The backend part is mainly responsible for receiving questionnaire answers submitted by users and broadcasting the questionnaire results to all connected clients. The backend can use any server that supports WebSocket, such as Node.js, Java, Python, etc.
3. Implementation steps
1. Front-end implementation
First, we need to introduce the WebSocket-related JavaScript library into the HTML page, as shown below:
<script> var socket = new WebSocket("ws://localhost:8080"); // 连接WebSocket服务器 socket.onopen = function() { // 连接建立成功 }; socket.onmessage = function(event) { // 接收到服务器发送的数据 var data = JSON.parse(event.data); // 处理问卷调查结果 }; socket.onclose = function(event) { // 连接关闭 }; </script>
In the above code , we create a WebSocket instance and establish a connection by specifying the server's address and port. We then handle interactions with the server by listening for events such as onopen
, onmessage
, and onclose
.
2. Backend implementation
Next, we need to implement the WebSocket server on the backend to receive the questionnaire answers submitted by the user and broadcast them to all connected clients. Taking Node.js as an example, we can use the ws
library to quickly build a WebSocket server.
First, we need to install the ws
library:
$ npm install ws
Then, write the server code in Node.js as follows:
const WebSocket = require('ws'); const wss = new WebSocket.Server({ port: 8080 }); wss.on('connection', function(ws) { ws.on('message', function(message) { // 接收到客户端发送的数据 // 处理问卷答案 // 广播问卷调查结果给所有连接的客户端 wss.clients.forEach(function(client) { if (client.readyState === WebSocket.OPEN) { client.send(JSON.stringify(result)); } }); }); });
In In the above code, we first create a WebSocket.Server instance and listen to the specified port. Then, we handle the client's connection request by listening to the connection
event. After the connection is successfully established, we listen to the message
event to process the message sent by the client. After receiving the message, we processed the questionnaire answers and broadcast the questionnaire results by looping through all connected clients.
4. Summary
Through JavaScript and WebSocket, we can easily implement a real-time online questionnaire survey system. The front end is responsible for displaying the questionnaire and receiving feedback from users, while the back end processes the questionnaire answers submitted by users and broadcasts the questionnaire results to all connected clients in real time. Through WebSocket's full-duplex communication, we can obtain user feedback in real time to better analyze and optimize products.
The above is a simple implementation example of a real-time online questionnaire system. I hope it will be helpful to you in implementing a similar system. Of course, in practical applications, safety, stability, and the improvement of other functions also need to be considered. I wish your online questionnaire system can achieve good results!
The above is the detailed content of How to use JavaScript and WebSocket to implement a real-time online questionnaire survey system. For more information, please follow other related articles on the PHP Chinese website!
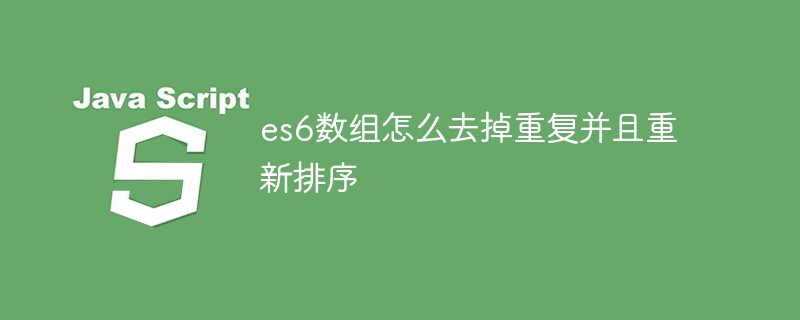
去掉重复并排序的方法:1、使用“Array.from(new Set(arr))”或者“[…new Set(arr)]”语句,去掉数组中的重复元素,返回去重后的新数组;2、利用sort()对去重数组进行排序,语法“去重数组.sort()”。
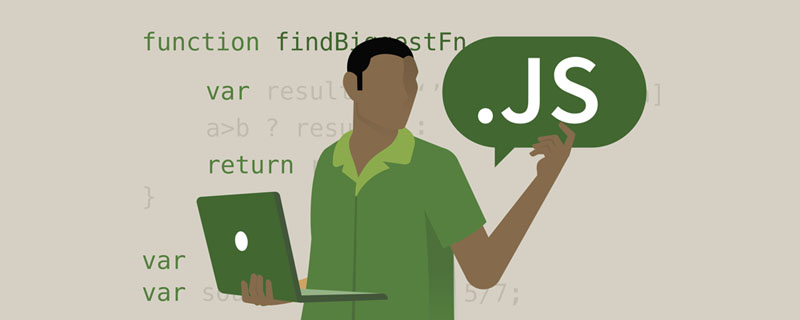
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于Symbol类型、隐藏属性及全局注册表的相关问题,包括了Symbol类型的描述、Symbol不会隐式转字符串等问题,下面一起来看一下,希望对大家有帮助。
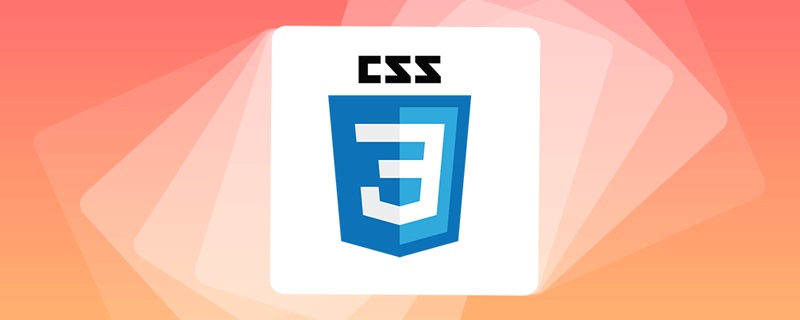
怎么制作文字轮播与图片轮播?大家第一想到的是不是利用js,其实利用纯CSS也能实现文字轮播与图片轮播,下面来看看实现方法,希望对大家有所帮助!
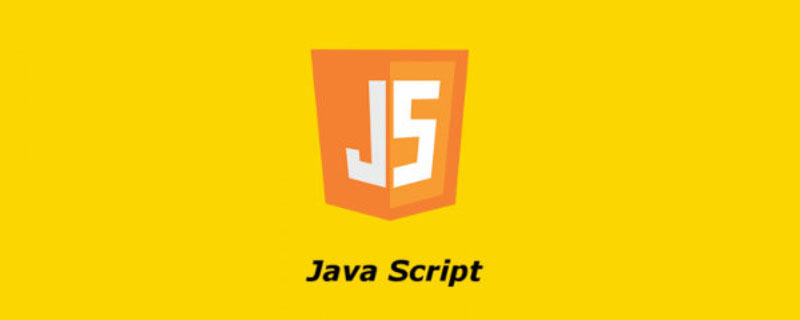
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于对象的构造函数和new操作符,构造函数是所有对象的成员方法中,最早被调用的那个,下面一起来看一下吧,希望对大家有帮助。
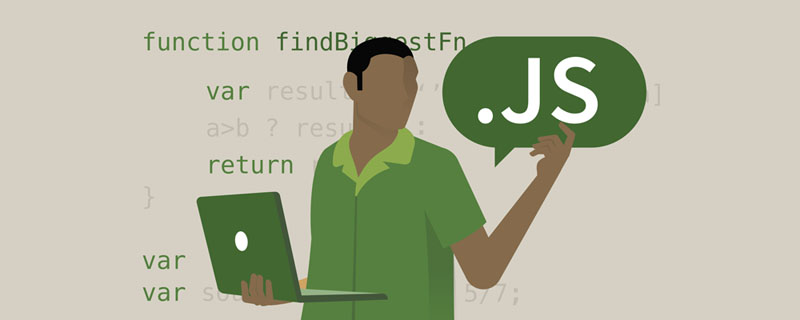
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于面向对象的相关问题,包括了属性描述符、数据描述符、存取描述符等等内容,下面一起来看一下,希望对大家有帮助。
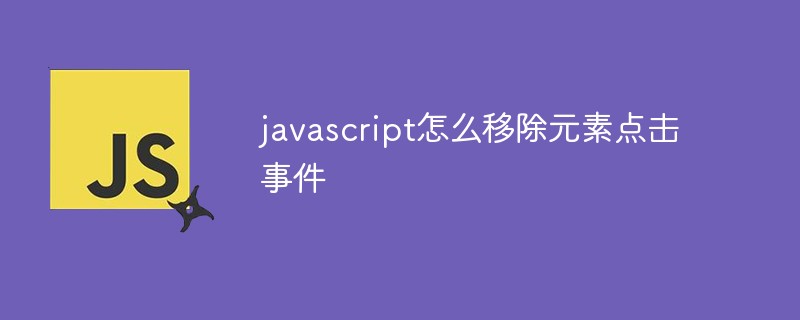
方法:1、利用“点击元素对象.unbind("click");”方法,该方法可以移除被选元素的事件处理程序;2、利用“点击元素对象.off("click");”方法,该方法可以移除通过on()方法添加的事件处理程序。
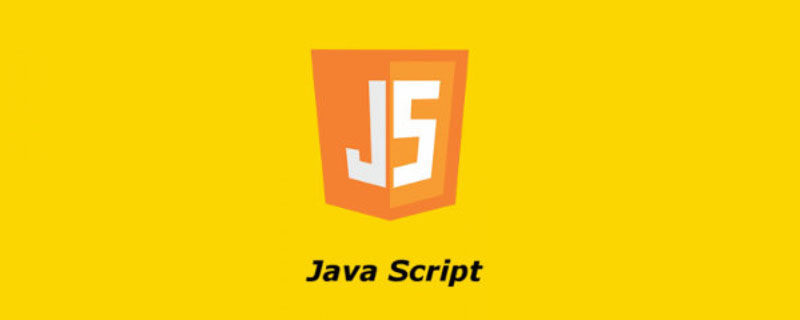
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于BOM操作的相关问题,包括了window对象的常见事件、JavaScript执行机制等等相关内容,下面一起来看一下,希望对大家有帮助。
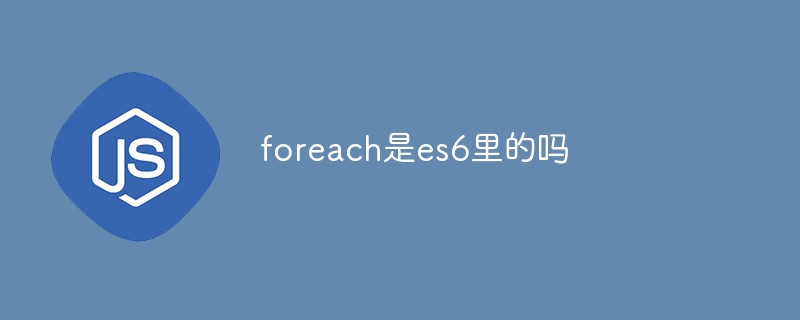
foreach不是es6的方法。foreach是es3中一个遍历数组的方法,可以调用数组的每个元素,并将元素传给回调函数进行处理,语法“array.forEach(function(当前元素,索引,数组){...})”;该方法不处理空数组。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
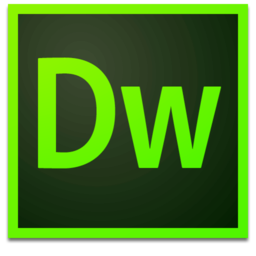
Dreamweaver Mac version
Visual web development tools

SublimeText3 Chinese version
Chinese version, very easy to use

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
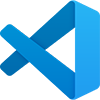
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
