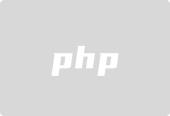
C Development Suggestions: How to Design Exception Safety in C Code
When developing C, exception safety is a crucial consideration. Exceptions refer to some errors or unexpected situations that may occur during the running of the program, while exception safety refers to the ability of the program to handle exceptions correctly without causing resource leaks or data inconsistencies. This article will give some suggestions on the design of exception safety in C code to help developers write more robust and reliable code.
- Use RAII to manage resources
RAII (Resource Acquisition Is Initialization) is a resource management technology that ensures that resources are acquired in the constructor of the object and released in the destructor. Correct release of resources. By using RAII technology, you can avoid the problem of resources not being released correctly due to exceptions. For example, when using smart pointers to manage dynamically allocated memory, or when using resources such as file handles and database connections, RAII can be used to simplify resource management.
- Exception safe function design
Exception handling should be considered when designing functions. There are three exception safety guarantee levels, which are:
- Strong exception safety (no-throw guarantee): When a function throws an exception, it will not leak resources or destroy the integrity of the data. This requires the use of a transaction mechanism to ensure that the operation can be rolled back to the original state when the operation fails.
- Basic exception safety (basic guarantee): When a function throws an exception, it will not leak resources, but it may cause partial damage to the data. This requires the use of appropriate data structures and algorithms to ensure data validity.
- Weak exception safety (nothrow guarantee): Functions may leak resources or destroy data integrity. In this case, additional steps need to be taken to handle the exception.
- Stack expansion strategy
Stack expansion refers to the process of how the system handles exceptions when an exception occurs during program running. In C, when an exception is thrown, the destructors of the objects on the stack are called one by one in the order in which they were created. In order to ensure exception safety, resource allocation should be placed in the appropriate object and the resources should be released when the object is destroyed. At the same time, you should avoid throwing exceptions in the constructor to prevent resource leaks.
- Use exception-safe standard libraries and third-party libraries
The C standard library and some third-party libraries have usually considered exception safety, and you can use the functions they provide to simplify the exception handling of your code. For example, use exception classes and exception-safe containers in the standard library to handle exceptions, or use exception-safe interfaces provided by third-party libraries.
- Proper handling and throwing of exceptions
When writing code, exceptions should be handled explicitly and thrown when needed. For code where exceptions may occur, try-catch statements should be used where appropriate to catch and handle exceptions. When handling exceptions, different handling should be carried out according to the specific situation, such as rollback operation, resource release, etc. At the same time, avoid throwing exceptions again in exception handling code to prevent nesting of exceptions.
- Use assertions for error handling
In addition to exception handling, it is also a good habit to use assertions for error handling. Assertions are some logical expressions added to the program to determine whether the program meets expected conditions. If the assertion fails, it means that there is an error in the program, and the problem can be discovered and located in time during the development and debugging stages.
To sum up, the exception safety design of C code needs to comprehensively consider factors such as resource management, function design, stack expansion, exception handling, and the use of standard libraries and assertions. Reasonable use of RAII, following exception-safe function design principles, and correctly handling and throwing exceptions can effectively improve the robustness and reliability of the code. Through reasonable exception handling strategies, various abnormal situations in C development can be better dealt with and the maintainability and scalability of the code can be improved.
The above is the detailed content of C++ development advice: How to design exception safety in C++ code. For more information, please follow other related articles on the PHP Chinese website!
Statement:The content of this article is voluntarily contributed by netizens, and the copyright belongs to the original author. This site does not assume corresponding legal responsibility. If you find any content suspected of plagiarism or infringement, please contact admin@php.cn