


How to use LinkedList.removeFirst() method to delete elements from the head of linked list in Java?
The LinkedList class in Java is a class that implements a linked list data structure. It provides many useful methods to operate linked lists. Among them, the removeFirst() method can be used to delete elements from the head of the linked list. The following will introduce how to use the LinkedList.removeFirst() method and give specific code examples.
Before using the LinkedList.removeFirst() method, we first need to create a LinkedList object and then add some elements to it. The following is a simple code example:
import java.util.LinkedList; public class LinkedListExample { public static void main(String[] args) { // 创建一个LinkedList对象 LinkedList<String> linkedList = new LinkedList<String>(); // 向链表中添加元素 linkedList.add("Apple"); linkedList.add("Banana"); linkedList.add("Orange"); linkedList.add("Grape"); // 输出链表的内容 System.out.println("链表的内容:" + linkedList); // 使用removeFirst()方法删除链表头部的元素 String firstElement = linkedList.removeFirst(); // 输出删除的元素 System.out.println("删除的元素:" + firstElement); // 输出删除元素后的链表内容 System.out.println("删除后的链表内容:" + linkedList); } }
Run the above code, the output is as follows:
链表的内容:[Apple, Banana, Orange, Grape] 删除的元素:Apple 删除后的链表内容:[Banana, Orange, Grape]
In this example, we use the LinkedList class to create a linked list object linkedList and add it to it four elements. Then, we use the removeFirst() method to delete the element at the head of the linked list and save the deleted element in the firstElement variable. Finally, we output the deleted elements and the contents of the linked list after the deleted elements.
It should be noted that when calling the removeFirst() method, if the linked list is empty, a NoSuchElementException will be thrown. Therefore, before calling the removeFirst() method, you should first determine whether the linked list is empty. You can use the isEmpty() method to determine. If the linked list is empty, you can choose to take corresponding processing methods, such as outputting a prompt message or performing other operations.
To sum up, using the LinkedList.removeFirst() method in Java can conveniently delete elements from the head of the linked list. Through this simple method, we can flexibly operate linked lists, making our code more efficient and easier to maintain.
The above is the detailed content of How to use LinkedList.removeFirst() method to delete elements from the head of linked list in Java?. For more information, please follow other related articles on the PHP Chinese website!
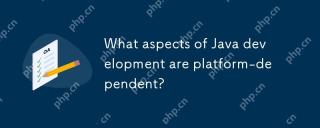
Javadevelopmentisnotentirelyplatform-independentduetoseveralfactors.1)JVMvariationsaffectperformanceandbehavioracrossdifferentOS.2)NativelibrariesviaJNIintroduceplatform-specificissues.3)Filepathsandsystempropertiesdifferbetweenplatforms.4)GUIapplica
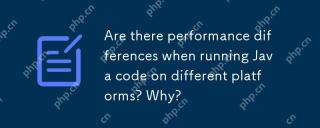
Java code will have performance differences when running on different platforms. 1) The implementation and optimization strategies of JVM are different, such as OracleJDK and OpenJDK. 2) The characteristics of the operating system, such as memory management and thread scheduling, will also affect performance. 3) Performance can be improved by selecting the appropriate JVM, adjusting JVM parameters and code optimization.
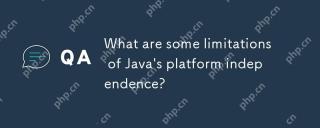
Java'splatformindependencehaslimitationsincludingperformanceoverhead,versioncompatibilityissues,challengeswithnativelibraryintegration,platform-specificfeatures,andJVMinstallation/maintenance.Thesefactorscomplicatethe"writeonce,runanywhere"
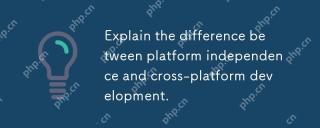
Platformindependenceallowsprogramstorunonanyplatformwithoutmodification,whilecross-platformdevelopmentrequiressomeplatform-specificadjustments.Platformindependence,exemplifiedbyJava,enablesuniversalexecutionbutmaycompromiseperformance.Cross-platformd
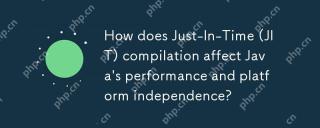
JITcompilationinJavaenhancesperformancewhilemaintainingplatformindependence.1)Itdynamicallytranslatesbytecodeintonativemachinecodeatruntime,optimizingfrequentlyusedcode.2)TheJVMremainsplatform-independent,allowingthesameJavaapplicationtorunondifferen
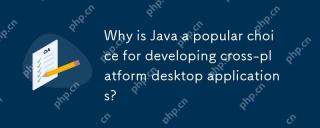
Javaispopularforcross-platformdesktopapplicationsduetoits"WriteOnce,RunAnywhere"philosophy.1)ItusesbytecodethatrunsonanyJVM-equippedplatform.2)LibrarieslikeSwingandJavaFXhelpcreatenative-lookingUIs.3)Itsextensivestandardlibrarysupportscompr
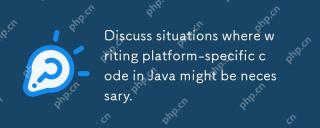
Reasons for writing platform-specific code in Java include access to specific operating system features, interacting with specific hardware, and optimizing performance. 1) Use JNA or JNI to access the Windows registry; 2) Interact with Linux-specific hardware drivers through JNI; 3) Use Metal to optimize gaming performance on macOS through JNI. Nevertheless, writing platform-specific code can affect the portability of the code, increase complexity, and potentially pose performance overhead and security risks.
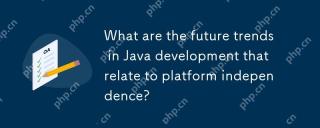
Java will further enhance platform independence through cloud-native applications, multi-platform deployment and cross-language interoperability. 1) Cloud native applications will use GraalVM and Quarkus to increase startup speed. 2) Java will be extended to embedded devices, mobile devices and quantum computers. 3) Through GraalVM, Java will seamlessly integrate with languages such as Python and JavaScript to enhance cross-language interoperability.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!
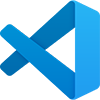
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
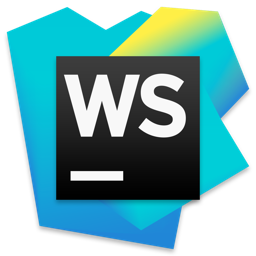
WebStorm Mac version
Useful JavaScript development tools
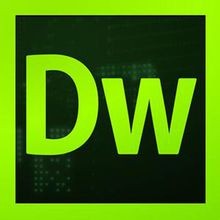
Dreamweaver CS6
Visual web development tools
