How to use Laravel to develop an online travel platform
With the rapid development of the tourism industry, more and more people choose to book travel products and services online. In order to meet this demand, it has become crucial to develop an efficient and reliable online travel platform. As a popular PHP framework, Laravel provides rich functions and convenient development experience, and is very suitable for developing online travel platforms.
This article will take the development of an online travel platform as an example to introduce how to use the Laravel framework for development, and provide specific code examples for reference.
- Environment setup
First, make sure your server environment has been set up and necessary software such as PHP, Composer and MySQL installed. Then, create a new Laravel project through Composer.
composer create-project --prefer-dist laravel/laravel travel-booking
- Database Design
Before you start writing code, you need to design the database structure. A typical online travel platform may include the following key models: users, travel products, orders, etc. Database tables can be easily created through Laravel's migration tool.
php artisan make:migration create_users_table --create=users php artisan make:migration create_tour_products_table --create=tour_products php artisan make:migration create_orders_table --create=orders
Edit these generated migration files, add the corresponding fields, and execute the migration instructions to apply the table structure to the database.
php artisan migrate
- Model and data filling
Define the model class corresponding to the database table, and define related relationships and attribute methods in the model, such as User model, TourProduct model, Order model, etc. At the same time, you can use Laravel's data filling function to quickly generate test data.
php artisan make:model User php artisan make:model TourProduct php artisan make:model Order
Edit the generated model file, define its association with other models and business logic, and use the factory class to generate test data.
php artisan make:factory UserFactory php artisan make:factory TourProductFactory php artisan make:factory OrderFactory
Edit the generated factory file, define the logic for generating test data, and use the Seeder class to populate the data into the database.
php artisan make:seeder UserSeeder php artisan make:seeder TourProductSeeder php artisan make:seeder OrderSeeder
Edit the generated Seeder file, add the data to be filled, and call the corresponding Seeder in the DatabaseSeeder class.
php artisan db:seed
- Routing and Controller
Configure routing to achieve access to different pages. In the routes/web.php file, configure the required routing rules.
Route::get('/', 'HomeController@index'); Route::get('/products', 'ProductController@index'); Route::get('/products/{id}', 'ProductController@show'); Route::post('/order', 'OrderController@store');
Configure corresponding controllers for different routes. The controller is responsible for accepting requests, processing business logic, and returning corresponding pages or data.
php artisan make:controller HomeController php artisan make:controller ProductController php artisan make:controller OrderController
Edit the generated controller file to interact with the database, obtain data through the model, and process related business logic.
- Views and styles
In the resources/views directory, create the corresponding view file to display the page content. You can use the Blade template engine to easily embed PHP code and variables.
resources/views/home.blade.php resources/views/products/index.blade.php resources/views/products/show.blade.php resources/views/orders/confirmation.blade.php
In the view file, you can use the form building tool provided by Laravel to generate a form to facilitate users to enter data.
In addition, in the public directory, you can place related style files and JavaScript scripts to control the appearance and interactive effects of the page.
- Front-end and back-end interaction
Through AJAX and other technologies, front-end and back-end data interaction is realized. The front-end page sends a request to the back-end controller, and the controller processes the request and returns the corresponding data.
You can use Laravel's response operation to return data to the front end in JSON format and process it accordingly.
- User authentication and authority control
Online travel platforms usually require user authentication and authority control functions. You can use the authentication and authorization mechanism provided by Laravel to quickly implement user registration, login, and role permission management.
php artisan make:auth
Execute the above instructions, Laravel will automatically generate controller, model and other files related to user authentication.
Edit the generated file, customize the user model and authentication method, configure role permissions, etc.
- Caching and Performance Optimization
In order to improve system performance, you can use Laravel's caching mechanism to save some frequently accessed data into the cache and reduce the number of database lookups.
You can use Laravel's cache operation to save data to the cache.
- Deployment and Maintenance
After completing development, the project can be deployed to the production environment. Project deployment, migration, and maintenance can be easily achieved using Laravel's commands.
php artisan config:cache php artisan route:cache php artisan optimize
The above are the main steps and code examples for using Laravel to develop an online travel platform. Of course, there are many details that need to be considered and improved during the actual development process. I hope this article is helpful to you, and I wish you good luck in developing a travel platform!
The above is the detailed content of How to develop an online travel platform using Laravel. For more information, please follow other related articles on the PHP Chinese website!
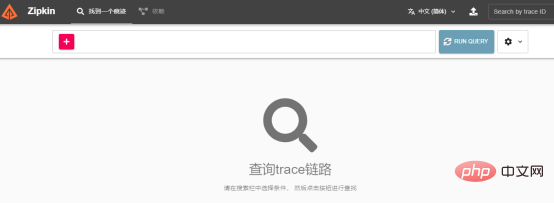
译者 | 李睿审校 | 孙淑娟随着Python越来越受欢迎,其局限性也越来越明显。一方面,编写Python应用程序并将其分发给没有安装Python的人员可能非常困难。解决这一问题的最常见方法是将程序与其所有支持库和文件以及Python运行时打包在一起。有一些工具可以做到这一点,例如PyInstaller,但它们需要大量的缓存才能正常工作。更重要的是,通常可以从生成的包中提取Python程序的源代码。在某些情况下,这会破坏交易。第三方项目Nuitka提供了一个激进的解决方案。它将Python程序编
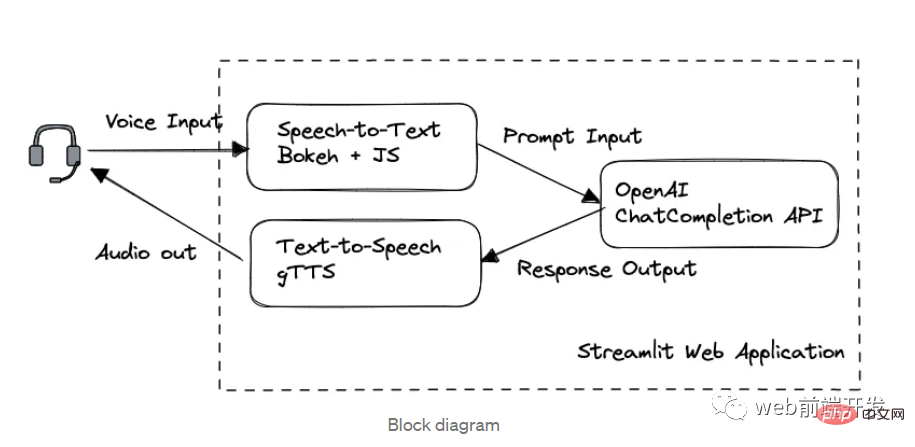
今天这篇文章的重点是使用 ChatGPT API 创建私人语音 Chatbot Web 应用程序。目的是探索和发现人工智能的更多潜在用例和商业机会。我将逐步指导您完成开发过程,以确保您理解并可以复制自己的过程。为什么需要不是每个人都欢迎基于打字的服务,想象一下仍在学习写作技巧的孩子或无法在屏幕上正确看到单词的老年人。基于语音的 AI Chatbot 是解决这个问题的方法,就像它如何帮助我的孩子要求他的语音 Chatbot 给他读睡前故事一样。鉴于现有可用的助手选项,例如,苹果的 Siri 和亚马
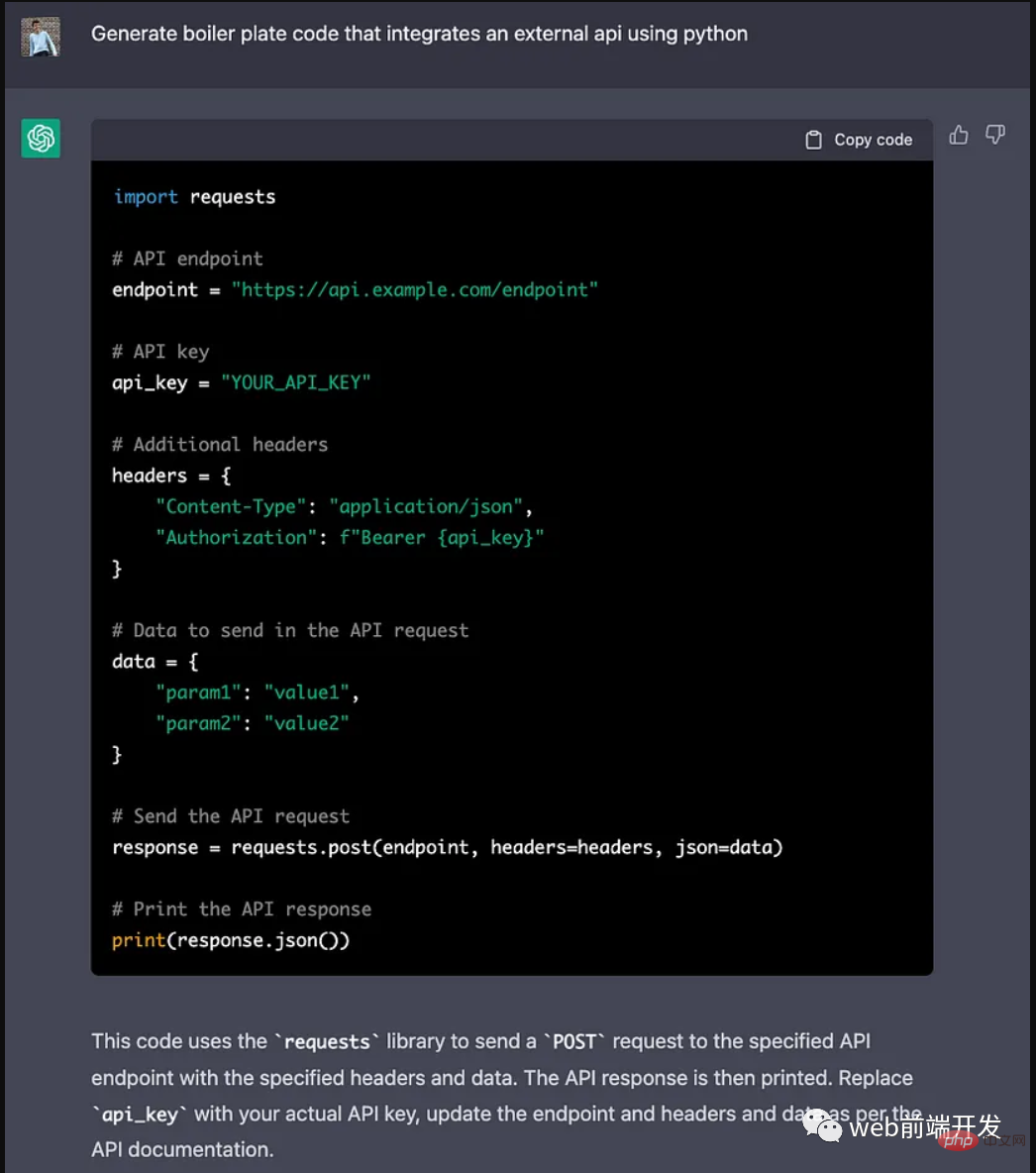
ChatGPT 目前彻底改变了开发代码的方式,然而,大多数软件开发人员和数据专家仍然没有使用 ChatGPT 来改进和简化他们的工作。这就是为什么我在这里概述 5 个不同的功能,以提高我们的日常工作速度和质量。我们可以在日常工作中使用它们。现在,我们一起来了解一下吧。注意:切勿在 ChatGPT 中使用关键代码或信息。01.生成项目代码的框架从头开始构建新项目时,ChatGPT 是我的秘密武器。只需几个提示,它就可以生成我需要的代码框架,包括我选择的技术、框架和版本。它不仅为我节省了至少一个小时
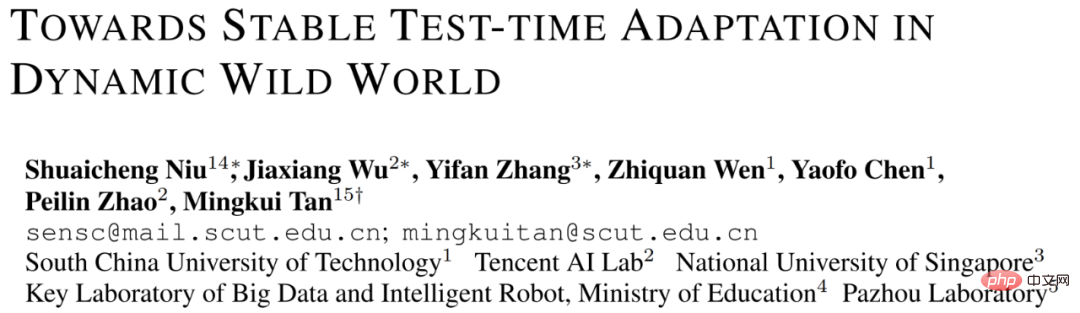
测试时自适应(Test-TimeAdaptation,TTA)方法在测试阶段指导模型进行快速无监督/自监督学习,是当前用于提升深度模型分布外泛化能力的一种强有效工具。然而在动态开放场景中,稳定性不足仍是现有TTA方法的一大短板,严重阻碍了其实际部署。为此,来自华南理工大学、腾讯AILab及新加坡国立大学的研究团队,从统一的角度对现有TTA方法在动态场景下不稳定原因进行分析,指出依赖于Batch的归一化层是导致不稳定的关键原因之一,另外测试数据流中某些具有噪声/大规模梯度的样本
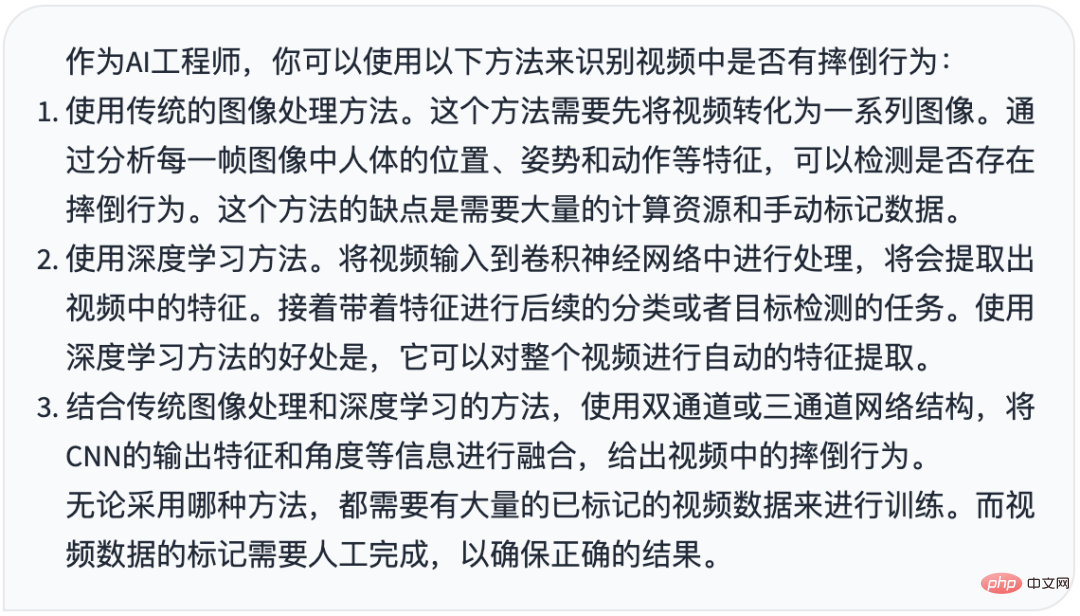
哈喽,大家好。之前给大家分享过摔倒识别、打架识别,今天以摔倒识别为例,我们看看能不能完全交给ChatGPT来做。让ChatGPT来做这件事,最核心的是如何向ChatGPT提问,把问题一股脑的直接丢给ChatGPT,如:用 Python 写个摔倒检测代码 是不可取的, 而是要像挤牙膏一样,一点一点引导ChatGPT得到准确的答案,从而才能真正让ChatGPT提高我们解决问题的效率。今天分享的摔倒识别案例,与ChatGPT对话的思路清晰,代码可用度高,按照GPT返回的结果完全可以开
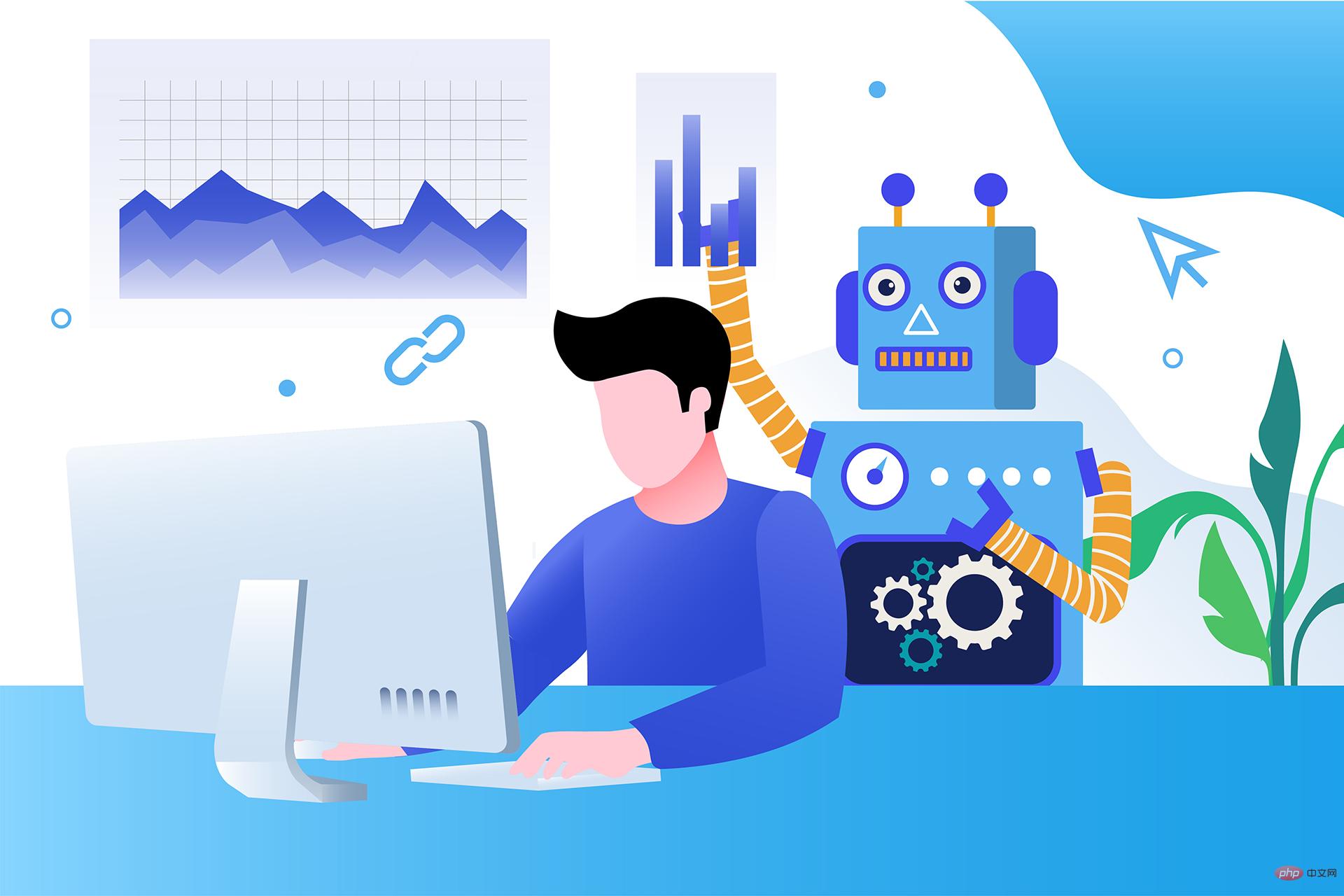
自 2020 年以来,内容开发领域已经感受到人工智能工具的存在。1.Jasper AI网址:https://www.jasper.ai在可用的 AI 文案写作工具中,Jasper 作为那些寻求通过内容生成赚钱的人来讲,它是经济实惠且高效的选择之一。该工具精通短格式和长格式内容均能完成。Jasper 拥有一系列功能,包括无需切换到模板即可快速生成内容的命令、用于创建文章的高效长格式编辑器,以及包含有助于创建各种类型内容的向导的内容工作流,例如,博客文章、销售文案和重写。Jasper Chat 是该
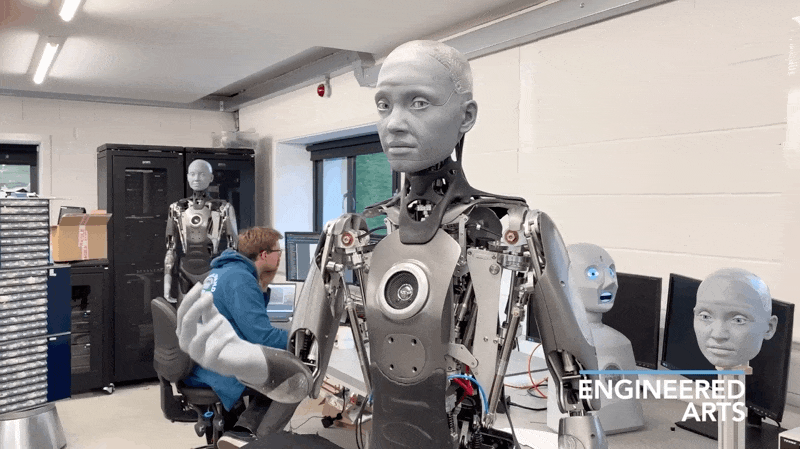
1970年,机器人专家森政弘(MasahiroMori)首次描述了「恐怖谷」的影响,这一概念对机器人领域产生了巨大影响。「恐怖谷」效应描述了当人类看到类似人类的物体,特别是机器人时所表现出的积极和消极反应。恐怖谷效应理论认为,机器人的外观和动作越像人,我们对它的同理心就越强。然而,在某些时候,机器人或虚拟人物变得过于逼真,但又不那么像人时,我们大脑的视觉处理系统就会被混淆。最终,我们会深深地陷入一种对机器人非常消极的情绪状态里。森政弘的假设指出:由于机器人与人类在外表、动作上相似,所以人类亦会对
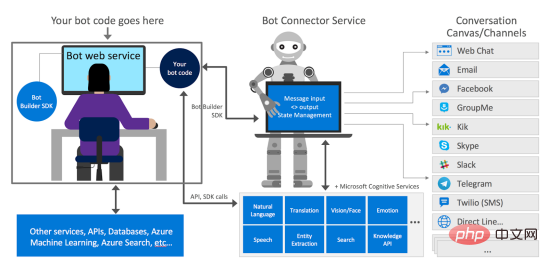
译者 | 李睿审校 | 孙淑娟信使、网络服务和其他软件都离不开机器人(bot)。而在软件开发和应用中,机器人是一种应用程序,旨在自动执行(或根据预设脚本执行)响应用户请求创建的操作。在本文中, NIX United公司的.NET开发人员Daniil Mikhov介绍了使用微软Azure Bot Services创建聊天机器人的一个例子。本文将对想要使用该服务开发聊天机器人的开发人员有所帮助。 为什么使用Azure Bot Services? 在Azure Bot Services上开发聊


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
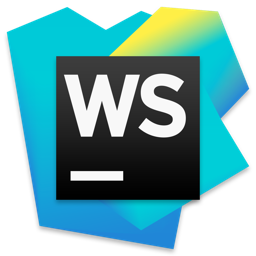
WebStorm Mac version
Useful JavaScript development tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
