How to perform system monitoring of C++ code?
How to perform system monitoring of C code?
As a programmer, designing and writing efficient and stable code is one of your responsibilities. However, even if you write the best code, you can't always avoid problems. In the development process, monitoring and debugging are very important links. They can help us discover potential problems and make timely repairs. This article will introduce how to perform system monitoring of C code to ensure the stability and reliability of the code.
- Exception handling
Exception handling is a very important part of C. By handling exceptions appropriately, we can avoid crashes or unpredictable behavior while our code is running. You can use try-catch blocks to catch and handle exceptions. In the catch block, we can record exception information, including the exception type and the values of related variables, for appropriate handling and debugging.
For example:
try { // 代码块 } catch (const std::exception& e) { // 处理异常 std::cout << "Caught exception: " << e.what() << std::endl; }
- Logging
Logging is a common monitoring and debugging method. By inserting appropriate logging statements into the code, we can record key information such as the values of variables, function calls and return values, etc. These logs can help us trace the execution path of the code and identify the problem.
You can use existing open source log libraries, such as Boost.Log, log4cpp, etc., or you can implement a simple logging system yourself.
For example:
// 添加日志记录函数 void log(const std::string& message) { std::ofstream logfile("log.txt", std::ofstream::app); if (logfile) { logfile << message << std::endl; } } // 在代码中记录日志 void someFunction() { // ... log("someFunction called"); // ... }
- Performance Analysis
During the development process, we want the code to run as efficiently as possible. Performance analysis tools can help us find bottlenecks and optimization points in the code. Performance analysis can be done using tools such as GNU gprof or Valgrind. These tools can generate code running time distribution, function call graph and other information to help us locate performance problems.
For example, using Valgrind for memory analysis:
valgrind --tool=memcheck --leak-check=yes ./yourprogram
- Resource Management
In C, manually managing resources is an important task. We need to ensure proper allocation and deallocation of resources to avoid memory leaks and other resource management issues. The use of smart pointers, RAII (Resource Acquisition Is Initialization) and other technologies can simplify the resource management process and improve the maintainability of the code.
For example, use smart pointers to manage dynamic memory:
std::shared_ptr<int> ptr(new int); // ...
- Unit testing
Unit testing is an effective way to verify the functional correctness of your code. By writing test cases, we can test the various inputs and expected outputs of the code to ensure the correctness of the code under various circumstances. You can use unit testing frameworks such as Google Test to perform automated unit testing.
For example:
TEST(MyClassTest, FunctionTest) { MyClass myObj; EXPECT_EQ(myObj.someFunction(1), 2); // ... }
In summary, system monitoring of C code requires the comprehensive application of multiple technologies and methods. Through reasonable exception handling, logging, performance analysis, resource management and unit testing, we can better monitor and debug C code and improve the reliability and stability of the code. Hope this article is helpful to you.
The above is the detailed content of How to perform system monitoring of C++ code?. For more information, please follow other related articles on the PHP Chinese website!
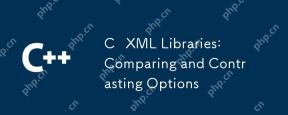
There are four commonly used XML libraries in C: TinyXML-2, PugiXML, Xerces-C, and RapidXML. 1.TinyXML-2 is suitable for environments with limited resources, lightweight but limited functions. 2. PugiXML is fast and supports XPath query, suitable for complex XML structures. 3.Xerces-C is powerful, supports DOM and SAX resolution, and is suitable for complex processing. 4. RapidXML focuses on performance and parses extremely fast, but does not support XPath queries.
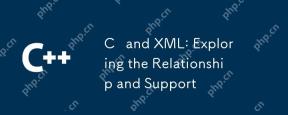
C interacts with XML through third-party libraries (such as TinyXML, Pugixml, Xerces-C). 1) Use the library to parse XML files and convert them into C-processable data structures. 2) When generating XML, convert the C data structure to XML format. 3) In practical applications, XML is often used for configuration files and data exchange to improve development efficiency.
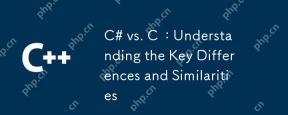
The main differences between C# and C are syntax, performance and application scenarios. 1) The C# syntax is more concise, supports garbage collection, and is suitable for .NET framework development. 2) C has higher performance and requires manual memory management, which is often used in system programming and game development.
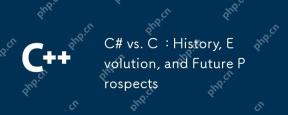
The history and evolution of C# and C are unique, and the future prospects are also different. 1.C was invented by BjarneStroustrup in 1983 to introduce object-oriented programming into the C language. Its evolution process includes multiple standardizations, such as C 11 introducing auto keywords and lambda expressions, C 20 introducing concepts and coroutines, and will focus on performance and system-level programming in the future. 2.C# was released by Microsoft in 2000. Combining the advantages of C and Java, its evolution focuses on simplicity and productivity. For example, C#2.0 introduced generics and C#5.0 introduced asynchronous programming, which will focus on developers' productivity and cloud computing in the future.
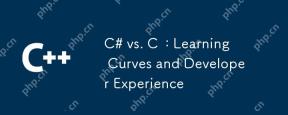
There are significant differences in the learning curves of C# and C and developer experience. 1) The learning curve of C# is relatively flat and is suitable for rapid development and enterprise-level applications. 2) The learning curve of C is steep and is suitable for high-performance and low-level control scenarios.
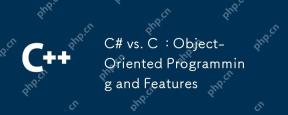
There are significant differences in how C# and C implement and features in object-oriented programming (OOP). 1) The class definition and syntax of C# are more concise and support advanced features such as LINQ. 2) C provides finer granular control, suitable for system programming and high performance needs. Both have their own advantages, and the choice should be based on the specific application scenario.
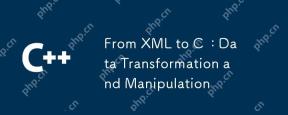
Converting from XML to C and performing data operations can be achieved through the following steps: 1) parsing XML files using tinyxml2 library, 2) mapping data into C's data structure, 3) using C standard library such as std::vector for data operations. Through these steps, data converted from XML can be processed and manipulated efficiently.
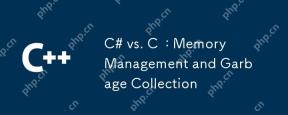
C# uses automatic garbage collection mechanism, while C uses manual memory management. 1. C#'s garbage collector automatically manages memory to reduce the risk of memory leakage, but may lead to performance degradation. 2.C provides flexible memory control, suitable for applications that require fine management, but should be handled with caution to avoid memory leakage.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
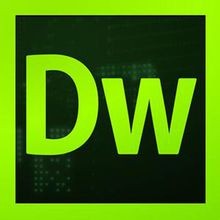
Dreamweaver CS6
Visual web development tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

Zend Studio 13.0.1
Powerful PHP integrated development environment

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool