


How to design a reliable MySQL table structure to implement file storage function?
How to design a reliable MySQL table structure to implement file storage function?
Currently, file storage has become an integral part of many applications. When designing a reliable MySQL table structure, we need to consider the following key factors:
- File storage method
File storage can be done in two ways: store the file directly in the database, or Store the file on disk and store the path to the file in the database. Storing files directly in the database simplifies management, but may affect database performance for large files. Therefore, when designing a reliable MySQL table structure, we recommend storing the file on disk and storing the path to the file in the database. - Table structure design
Create a file table in the database to manage file-related information. The structure of the file table can include the following fields:
-
#id
: file ID, as the primary key; -
name
: file Name; -
path
: file path; -
size
: file size; -
type
: File type; -
created_at
: file creation time; -
updated_at
: file update time.
For example, you can use the following SQL statement to create a file table:
CREATE TABLE `file` ( `id` int(11) NOT NULL AUTO_INCREMENT, `name` varchar(255) NOT NULL, `path` varchar(255) NOT NULL, `size` int(11) NOT NULL, `type` varchar(255) NOT NULL, `created_at` timestamp NOT NULL DEFAULT CURRENT_TIMESTAMP, `updated_at` timestamp NOT NULL DEFAULT CURRENT_TIMESTAMP ON UPDATE CURRENT_TIMESTAMP, PRIMARY KEY (`id`) ) ENGINE=InnoDB DEFAULT CHARSET=utf8mb4;
- File operation interface design
In order to implement operations such as uploading, downloading, and deleting files, we Corresponding operation interfaces can be designed for file tables. The following is a simple PHP sample code:
<?php function uploadFile($file) { $name = $file['name']; $path = 'uploads/' . $name; $size = $file['size']; $type = $file['type']; move_uploaded_file($file['tmp_name'], $path); $sql = "INSERT INTO `file` (`name`, `path`, `size`, `type`) VALUES ('$name', '$path', $size, '$type')"; // 执行SQL语句插入文件信息 return true; } function downloadFile($id) { $sql = "SELECT `path` FROM `file` WHERE `id` = $id"; // 执行SQL语句查询文件路径 $path = $result['path']; header('Content-Type: application/octet-stream'); header("Content-Disposition: attachment; filename="" . basename($path) . """); readfile($path); return true; } function deleteFile($id) { $sql = "SELECT `path` FROM `file` WHERE `id` = $id"; // 执行SQL语句查询文件路径 $path = $result['path']; unlink($path); $sql = "DELETE FROM `file` WHERE `id` = $id"; // 执行SQL语句删除文件信息 return true; } ?>
In actual applications, we can perform appropriate expansion and optimization according to needs, such as adding file permission management, file preview and other functions.
In summary, designing a reliable MySQL table structure to implement file storage function requires considering the file storage method, table structure design and file operation interface design. Through reasonable design and management, we can achieve efficient and stable file storage functions.
The above is the detailed content of How to design a reliable MySQL table structure to implement file storage function?. For more information, please follow other related articles on the PHP Chinese website!
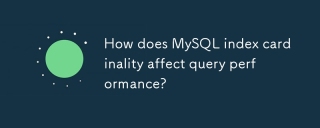
MySQL index cardinality has a significant impact on query performance: 1. High cardinality index can more effectively narrow the data range and improve query efficiency; 2. Low cardinality index may lead to full table scanning and reduce query performance; 3. In joint index, high cardinality sequences should be placed in front to optimize query.
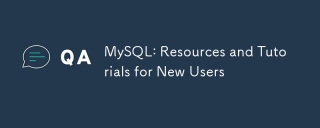
The MySQL learning path includes basic knowledge, core concepts, usage examples, and optimization techniques. 1) Understand basic concepts such as tables, rows, columns, and SQL queries. 2) Learn the definition, working principles and advantages of MySQL. 3) Master basic CRUD operations and advanced usage, such as indexes and stored procedures. 4) Familiar with common error debugging and performance optimization suggestions, such as rational use of indexes and optimization queries. Through these steps, you will have a full grasp of the use and optimization of MySQL.
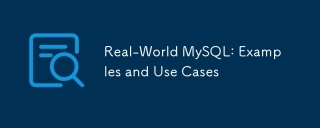
MySQL's real-world applications include basic database design and complex query optimization. 1) Basic usage: used to store and manage user data, such as inserting, querying, updating and deleting user information. 2) Advanced usage: Handle complex business logic, such as order and inventory management of e-commerce platforms. 3) Performance optimization: Improve performance by rationally using indexes, partition tables and query caches.
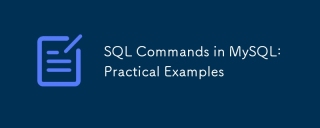
SQL commands in MySQL can be divided into categories such as DDL, DML, DQL, DCL, etc., and are used to create, modify, delete databases and tables, insert, update, delete data, and perform complex query operations. 1. Basic usage includes CREATETABLE creation table, INSERTINTO insert data, and SELECT query data. 2. Advanced usage involves JOIN for table joins, subqueries and GROUPBY for data aggregation. 3. Common errors such as syntax errors, data type mismatch and permission problems can be debugged through syntax checking, data type conversion and permission management. 4. Performance optimization suggestions include using indexes, avoiding full table scanning, optimizing JOIN operations and using transactions to ensure data consistency.
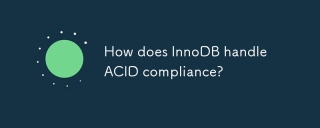
InnoDB achieves atomicity through undolog, consistency and isolation through locking mechanism and MVCC, and persistence through redolog. 1) Atomicity: Use undolog to record the original data to ensure that the transaction can be rolled back. 2) Consistency: Ensure the data consistency through row-level locking and MVCC. 3) Isolation: Supports multiple isolation levels, and REPEATABLEREAD is used by default. 4) Persistence: Use redolog to record modifications to ensure that data is saved for a long time.

MySQL's position in databases and programming is very important. It is an open source relational database management system that is widely used in various application scenarios. 1) MySQL provides efficient data storage, organization and retrieval functions, supporting Web, mobile and enterprise-level systems. 2) It uses a client-server architecture, supports multiple storage engines and index optimization. 3) Basic usages include creating tables and inserting data, and advanced usages involve multi-table JOINs and complex queries. 4) Frequently asked questions such as SQL syntax errors and performance issues can be debugged through the EXPLAIN command and slow query log. 5) Performance optimization methods include rational use of indexes, optimized query and use of caches. Best practices include using transactions and PreparedStatemen
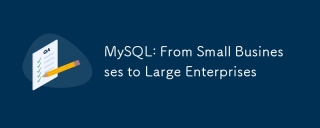
MySQL is suitable for small and large enterprises. 1) Small businesses can use MySQL for basic data management, such as storing customer information. 2) Large enterprises can use MySQL to process massive data and complex business logic to optimize query performance and transaction processing.
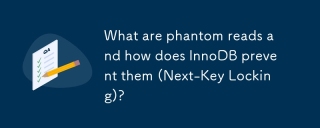
InnoDB effectively prevents phantom reading through Next-KeyLocking mechanism. 1) Next-KeyLocking combines row lock and gap lock to lock records and their gaps to prevent new records from being inserted. 2) In practical applications, by optimizing query and adjusting isolation levels, lock competition can be reduced and concurrency performance can be improved.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

SublimeText3 Linux new version
SublimeText3 Linux latest version

Atom editor mac version download
The most popular open source editor

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
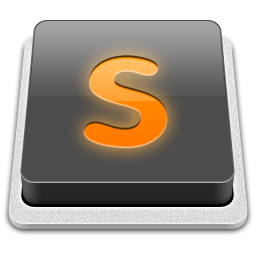
SublimeText3 Mac version
God-level code editing software (SublimeText3)