InnoDB achieves atomicity through undo log, consistency and isolation through locking mechanism and MVCC, and persistence through redo log. 1) Atomicity: Use undo log to record the original data to ensure that the transaction can be rolled back. 2) Consistency: Ensure the data consistency through row-level locking and MVCC. 3) Isolation: Supports multiple isolation levels, and REPEATABLE READ is used by default. 4) Persistence: Use redo log to record modifications to ensure that data is saved for a long time.
introduction
In the world of databases, ACID (atomicity, consistency, isolation, persistence) is an important criterion for measuring transaction processing capabilities. Today we are going to discuss how the InnoDB storage engine implements these features. As the most commonly used storage engine in MySQL, InnoDB is known for its powerful ACID support. Through this article, you will gain an in-depth understanding of how InnoDB ensures the integrity and reliability of your data. At the same time, I will share some experiences and pitfalls I encountered when using InnoDB in actual projects.
Review of basic knowledge
Before we dive into the ACID implementation of InnoDB, we will briefly review what ACID is. Atomicity ensures that transactions are either completed or not completed; consistency ensures that the database remains consistent before and after transactions; isolation ensures that transactions do not interfere with each other; persistence ensures that data is permanently saved once transactions are committed. As a storage engine for relational databases, InnoDB uses a variety of technologies to implement these features.
Core concept or function analysis
How InnoDB implements atomicity
InnoDB achieves atomicity by using undo log (rollback log). When the transaction begins, InnoDB records the original values of all data to be modified. If a transaction fails during execution or is rolled back, InnoDB will use undo log to restore the data to the state before the transaction starts. This ensures the atomicity of the transaction.
-- Example: Transaction Rollback START TRANSACTION; INSERT INTO users (name, email) VALUES ('John Doe', 'john@example.com'); -- Assume that an error ROLLBACK occurs here; -- After the transaction is rolled back, 'John Doe' will not be inserted into the users table
In actual projects, I have encountered transaction interruptions due to network problems. Fortunately, InnoDB's undo log mechanism can safely roll back data, avoiding the problem of data inconsistency.
How InnoDB achieves consistency
Consistency is achieved through InnoDB's lock mechanism and MVCC (multi-version concurrent control). InnoDB uses row-level locks to ensure that other transactions cannot modify the data being modified during transaction execution. MVCC ensures that the data seen by the transaction is consistent by creating a snapshot for each transaction.
-- Example: MVCC START TRANSACTION; SELECT * FROM users WHERE id = 1; -- Snapshot seen by transaction 1 -- Other transactions may have modified the record with id = 1 COMMIT at this time; -- After transaction 1 is submitted, you still see a snapshot from the beginning
When using MVCC, I found a common misunderstanding that it can completely avoid the use of locks. In fact, MVCC still needs locking in some cases, especially when writing operations, which requires special attention.
How InnoDB achieves isolation
Isolation is achieved through InnoDB's locking mechanism and MVCC. InnoDB supports multiple isolation levels (READ UNCOMMITTED, READ COMMITTED, REPEATABLE READ, SERIALIZABLE), and uses REPEATABLE READ by default. Through these isolation levels, InnoDB ensures that transactions are not affected by other transactions during execution.
-- Example: Isolation Level SET TRANSACTION ISOLATION LEVEL REPEATABLE READ; START TRANSACTION; SELECT * FROM users WHERE id = 1; -- Snapshot seen by transaction 1 -- Other transactions may have modified the record with id=1 at this time SELECT * FROM users WHERE id = 1; -- What transaction 1 still sees is the snapshot at the beginning COMMIT;
In actual projects, I found that the choice of isolation level has a great impact on performance. Although REPEATABLE READ provides high isolation, it may also lead to more lock waiting and deadlock problems, which need to be weighed according to the specific business scenario.
How InnoDB achieves persistence
Persistence is achieved through InnoDB's redo log (redo log). The redo log records all modifications to the data by the transaction. Once the transaction is committed, these modifications will be written to the redo log. Even if the database crashes, InnoDB can recover data through redo log to ensure the persistence of the data.
-- Example: redo log START TRANSACTION; INSERT INTO users (name, email) VALUES ('Jane Doe', 'jane@example.com'); COMMIT; -- After the transaction is submitted, the modification will be written to the redo log
When using redo log, I have encountered the problem that redo log cannot be written due to insufficient disk space, which reminds us that we need to pay special attention to disk space management when configuring InnoDB.
Example of usage
Basic usage
The basic usage of InnoDB is very simple, just specify the InnoDB storage engine when creating the table.
CREATE TABLE users ( id INT PRIMARY KEY AUTO_INCREMENT, name VARCHAR(100), email VARCHAR(100) ) ENGINE=InnoDB;
In actual projects, I found that using the basic configuration of InnoDB can already meet most needs, but sometimes some parameters need to be adjusted according to the specific business, such as innodb_buffer_pool_size.
Advanced Usage
Advanced usage of InnoDB includes the use of transactions, locks, and MVCC to handle complex business logic.
-- Example: Using transactions and locks START TRANSACTION; SELECT * FROM users WHERE id = 1 FOR UPDATE; -- Get exclusive lock UPDATE users SET name = 'New Name' WHERE id = 1; COMMIT;
When using advanced features, I found that special attention should be paid to the use of locks to avoid performance problems caused by lock competition. At the same time, the rational use of MVCC can significantly improve concurrency performance.
Common Errors and Debugging Tips
Common errors when using InnoDB include deadlocks, lock waiting timeouts, and transaction rollback failures. When debugging these problems, you can use InnoDB monitoring tools, such as SHOW ENGINE INNODB STATUS, to view the current lock status and transaction information.
-- Example: View InnoDB status SHOW ENGINE INNODB STATUS;
In actual projects, I found that using InnoDB's monitoring tools can quickly locate and solve problems, but it should be noted that these tools may have a certain impact on performance and need to be used with caution in production environments.
Performance optimization and best practices
In practical applications, optimizing the performance of InnoDB requires many aspects to be started. The first is to adjust the configuration parameters of InnoDB, such as innodb_buffer_pool_size, innodb_log_file_size, etc. The adjustment of these parameters can significantly improve performance.
-- Example: Adjust InnoDB configuration SET GLOBAL innodb_buffer_pool_size = 128 * 1024 * 1024 * 1024; -- Set to 128GB
In actual projects, I found that adjusting these parameters needs to be done according to specific hardware and business needs, and blind adjustments may lead to performance degradation. The second is to optimize SQL queries. Using indexes and avoiding full table scanning can significantly improve query performance.
-- Example: Using index CREATE INDEX idx_name ON users(name);
Finally, there are programming habits and best practices, such as shortening the execution time of transactions as much as possible when using transactions and avoiding holding locks for a long time; when using MVCC, reasonably select isolation levels to balance performance and consistency.
In actual projects, I found that these best practices not only improve performance, but also improve the readability and maintenance of the code. In short, InnoDB's ACID implementation is the basis of its powerful capabilities. Understanding and correct use of these features can help us better manage data and ensure data integrity and reliability.
The above is the detailed content of How does InnoDB handle ACID compliance?. For more information, please follow other related articles on the PHP Chinese website!
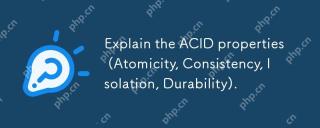
ACID attributes include atomicity, consistency, isolation and durability, and are the cornerstone of database design. 1. Atomicity ensures that the transaction is either completely successful or completely failed. 2. Consistency ensures that the database remains consistent before and after a transaction. 3. Isolation ensures that transactions do not interfere with each other. 4. Persistence ensures that data is permanently saved after transaction submission.
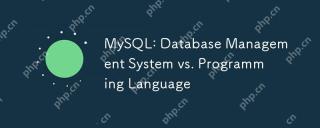
MySQL is not only a database management system (DBMS) but also closely related to programming languages. 1) As a DBMS, MySQL is used to store, organize and retrieve data, and optimizing indexes can improve query performance. 2) Combining SQL with programming languages, embedded in Python, using ORM tools such as SQLAlchemy can simplify operations. 3) Performance optimization includes indexing, querying, caching, library and table division and transaction management.
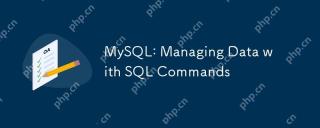
MySQL uses SQL commands to manage data. 1. Basic commands include SELECT, INSERT, UPDATE and DELETE. 2. Advanced usage involves JOIN, subquery and aggregate functions. 3. Common errors include syntax, logic and performance issues. 4. Optimization tips include using indexes, avoiding SELECT* and using LIMIT.
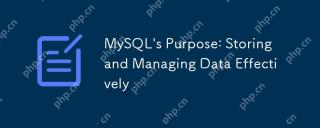
MySQL is an efficient relational database management system suitable for storing and managing data. Its advantages include high-performance queries, flexible transaction processing and rich data types. In practical applications, MySQL is often used in e-commerce platforms, social networks and content management systems, but attention should be paid to performance optimization, data security and scalability.
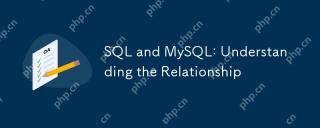
The relationship between SQL and MySQL is the relationship between standard languages and specific implementations. 1.SQL is a standard language used to manage and operate relational databases, allowing data addition, deletion, modification and query. 2.MySQL is a specific database management system that uses SQL as its operating language and provides efficient data storage and management.
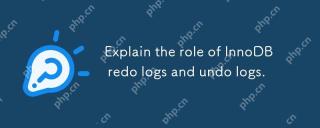
InnoDB uses redologs and undologs to ensure data consistency and reliability. 1.redologs record data page modification to ensure crash recovery and transaction persistence. 2.undologs records the original data value and supports transaction rollback and MVCC.
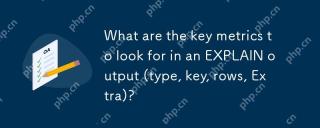
Key metrics for EXPLAIN commands include type, key, rows, and Extra. 1) The type reflects the access type of the query. The higher the value, the higher the efficiency, such as const is better than ALL. 2) The key displays the index used, and NULL indicates no index. 3) rows estimates the number of scanned rows, affecting query performance. 4) Extra provides additional information, such as Usingfilesort prompts that it needs to be optimized.
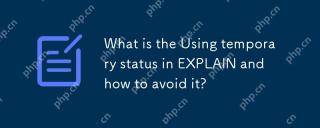
Usingtemporary indicates that the need to create temporary tables in MySQL queries, which are commonly found in ORDERBY using DISTINCT, GROUPBY, or non-indexed columns. You can avoid the occurrence of indexes and rewrite queries and improve query performance. Specifically, when Usingtemporary appears in EXPLAIN output, it means that MySQL needs to create temporary tables to handle queries. This usually occurs when: 1) deduplication or grouping when using DISTINCT or GROUPBY; 2) sort when ORDERBY contains non-index columns; 3) use complex subquery or join operations. Optimization methods include: 1) ORDERBY and GROUPB


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
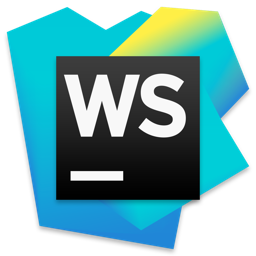
WebStorm Mac version
Useful JavaScript development tools
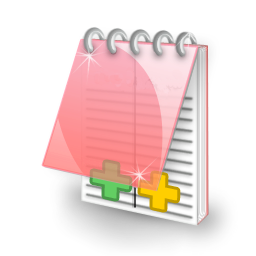
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
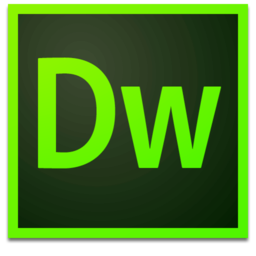
Dreamweaver Mac version
Visual web development tools

Zend Studio 13.0.1
Powerful PHP integrated development environment

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.