How to build a cloud storage service using Go language and Redis
How to build a cloud storage service using Go language and Redis
In the era of cloud computing, storage services are becoming more and more important. Cloud storage services allow users to store and access their data conveniently. This article will introduce how to use Go language and Redis to build a simple cloud storage service, and provide specific code examples.
1. Build the Redis environment
First, we need to build the Redis environment. You can download and install Redis through the Redis official website, and then start the Redis server locally.
2. Create a Go project
Next, create a new Go project in the terminal and create a main.go file in the project directory.
3. Import dependency packages
In the main.go file, we need to import some dependency packages, including Redis driver and HTTP service related packages. It can be installed and imported using Go's package management tools.
package main import ( "fmt" "log" "net/http" "github.com/go-redis/redis" )
4. Connect to Redis
We need to connect to the Redis server in the code. You can create a Redis client through the redis.NewClient function and set connection options using redis.NewClientOptions. In the specific code, you need to fill in your own Redis server address and password.
func main() { client := redis.NewClient(&redis.Options{ Addr: "localhost:6379", Password: "", //填写自己的Redis密码 DB: 0, }) pong, err := client.Ping().Result() if err != nil { log.Fatal(err) } fmt.Println("Connected to Redis:", pong) }
5. Processing HTTP requests
Next, we will process HTTP requests so that users can upload and download files through the HTTP interface.
First, we need to write a function that handles file uploads. In this function, we will save the file in Redis and return a unique file ID.
func uploadFile(client *redis.Client) http.HandlerFunc { return func(w http.ResponseWriter, r *http.Request) { r.ParseMultipartForm(32 << 20) file, handler, err := r.FormFile("file") if err != nil { log.Println(err) http.Error(w, "Failed to upload file", http.StatusInternalServerError) return } defer file.Close() bytes, err := ioutil.ReadAll(file) if err != nil { log.Println(err) http.Error(w, "Failed to read file", http.StatusInternalServerError) return } fileID := uuid.NewString() err = client.Set(fileID, bytes, 0).Err() if err != nil { log.Println(err) http.Error(w, "Failed to save file", http.StatusInternalServerError) return } response := map[string]string{"fileID": fileID} jsonResponse, err := json.Marshal(response) if err != nil { log.Println(err) http.Error(w, "Failed to create JSON response", http.StatusInternalServerError) return } w.Header().Set("Content-Type", "application/json") w.Write(jsonResponse) } }
Then, we write a function that handles file downloading. In this function, we will get the file content based on the file ID and return the file content to the user as an HTTP response.
func downloadFile(client *redis.Client) http.HandlerFunc { return func(w http.ResponseWriter, r *http.Request) { fileID := r.URL.Query().Get("fileID") bytes, err := client.Get(fileID).Bytes() if err != nil { log.Println(err) http.Error(w, "Failed to get file", http.StatusInternalServerError) return } w.Header().Set("Content-Type", "application/octet-stream") w.Header().Set("Content-Disposition", fmt.Sprintf("attachment; filename=%s", fileID)) w.Write(bytes) } }
Finally, we create an HTTP route in the main function and run the HTTP service.
func main() { ... http.HandleFunc("/upload", uploadFile(client)) http.HandleFunc("/download", downloadFile(client)) log.Fatal(http.ListenAndServe(":8080", nil)) }
6. Running and testing
Now, we can run the program and test it using tools such as curl or Postman.
First, run the program using the following command:
go run main.go
Then, use the following command to upload the file:
curl -X POST -H "Content-Type: multipart/form-data" -F "file=@/path/to/file" http://localhost:8080/upload
Where, "/path/to/file" should be replaced with local The path to the file.
Finally, use the following command to download the file:
curl -OJ http://localhost:8080/download?fileID=<fileID>
Among them, "
7. Summary
Through the sample code in this article, we learned how to use Go language and Redis to build a simple cloud storage service. This service can upload and download files through the HTTP interface. Of course, this is just a basic example. Actual cloud storage services may also need to consider many other aspects, such as user rights management, file sharding, data backup, etc. But through this article, you can understand the general ideas and methods of building cloud storage services using Go language and Redis, laying the foundation for further development.
The above is the detailed content of How to build a cloud storage service using Go language and Redis. For more information, please follow other related articles on the PHP Chinese website!
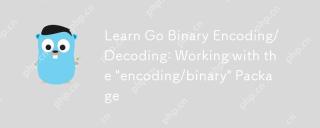
Go uses the "encoding/binary" package for binary encoding and decoding. 1) This package provides binary.Write and binary.Read functions for writing and reading data. 2) Pay attention to choosing the correct endian (such as BigEndian or LittleEndian). 3) Data alignment and error handling are also key to ensure the correctness and performance of the data.
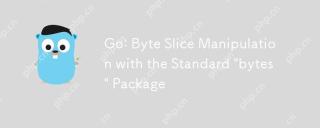
The"bytes"packageinGooffersefficientfunctionsformanipulatingbyteslices.1)Usebytes.Joinforconcatenatingslices,2)bytes.Bufferforincrementalwriting,3)bytes.Indexorbytes.IndexByteforsearching,4)bytes.Readerforreadinginchunks,and5)bytes.SplitNor
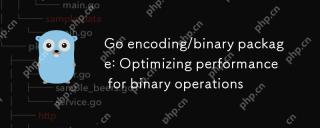
Theencoding/binarypackageinGoiseffectiveforoptimizingbinaryoperationsduetoitssupportforendiannessandefficientdatahandling.Toenhanceperformance:1)Usebinary.NativeEndianfornativeendiannesstoavoidbyteswapping.2)BatchReadandWriteoperationstoreduceI/Oover
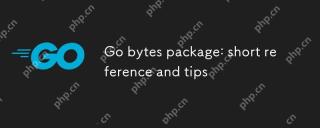
Go's bytes package is mainly used to efficiently process byte slices. 1) Using bytes.Buffer can efficiently perform string splicing to avoid unnecessary memory allocation. 2) The bytes.Equal function is used to quickly compare byte slices. 3) The bytes.Index, bytes.Split and bytes.ReplaceAll functions can be used to search and manipulate byte slices, but performance issues need to be paid attention to.
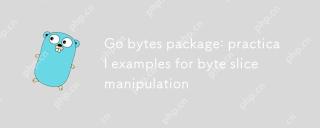
The byte package provides a variety of functions to efficiently process byte slices. 1) Use bytes.Contains to check the byte sequence. 2) Use bytes.Split to split byte slices. 3) Replace the byte sequence bytes.Replace. 4) Use bytes.Join to connect multiple byte slices. 5) Use bytes.Buffer to build data. 6) Combined bytes.Map for error processing and data verification.
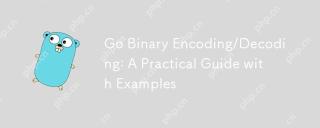
Go's encoding/binary package is a tool for processing binary data. 1) It supports small-endian and large-endian endian byte order and can be used in network protocols and file formats. 2) The encoding and decoding of complex structures can be handled through Read and Write functions. 3) Pay attention to the consistency of byte order and data type when using it, especially when data is transmitted between different systems. This package is suitable for efficient processing of binary data, but requires careful management of byte slices and lengths.
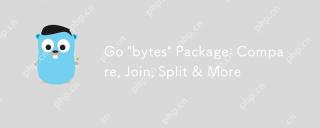
The"bytes"packageinGoisessentialbecauseitoffersefficientoperationsonbyteslices,crucialforbinarydatahandling,textprocessing,andnetworkcommunications.Byteslicesaremutable,allowingforperformance-enhancingin-placemodifications,makingthispackage
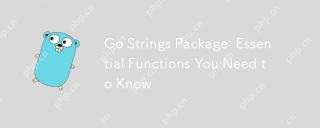
Go'sstringspackageincludesessentialfunctionslikeContains,TrimSpace,Split,andReplaceAll.1)Containsefficientlychecksforsubstrings.2)TrimSpaceremoveswhitespacetoensuredataintegrity.3)SplitparsesstructuredtextlikeCSV.4)ReplaceAlltransformstextaccordingto


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor
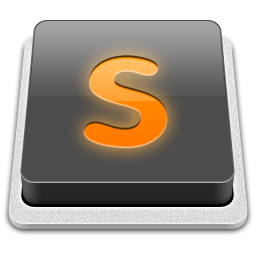
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SublimeText3 Chinese version
Chinese version, very easy to use

SublimeText3 Linux new version
SublimeText3 Linux latest version
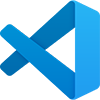
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
