


HTML, CSS, and jQuery: A technical guide to implementing drag-and-drop sorting
HTML, CSS and jQuery: Technical Guide to Implementing Drag-and-Drop Sorting
In modern web design, drag-and-drop sorting is a very common feature. It allows users to sort by dragging elements, and provides a good user experience during real-time updates. This article will introduce you how to use HTML, CSS and jQuery to implement a simple drag-and-drop sorting function.
Before we start, we first need to prepare some basic HTML structures and CSS styles, which will provide a foundation for our subsequent drag and drop sorting.
HTML structure:
<ul id="sortable"> <li>项目 1</li> <li>项目 2</li> <li>项目 3</li> <li>项目 4</li> <li>项目 5</li> </ul>
CSS style:
#sortable { list-style-type: none; margin: 0; padding: 0; } #sortable li { cursor: move; padding: 10px; margin-bottom: 5px; background-color: #f5f5f5; border: 1px solid #ccc; }
Next, we need to introduce the jQuery library and some jQuery UI plug-ins, which will provide us with the ability to implement drag and drop sorting necessary support. Insert the following code into the tag of the web page:
<link rel="stylesheet" href="https://code.jquery.com/ui/1.12.1/themes/base/jquery-ui.css"> <script src="https://code.jquery.com/jquery-3.5.1.min.js"></script> <script src="https://code.jquery.com/ui/1.12.1/jquery-ui.js"></script>
Now that we have prepared all the necessary code, libraries and plug-ins, we can start to implement drag and drop sorting .
First, we need to use jQuery UI's sortable()
method to make the list drag-and-drop sortable. Insert the following code in the JavaScript code:
$(document).ready(function() { $("#sortable").sortable(); });
Next, we need to add a callback function for the event after the sorting is completed, so that we can obtain the updated sorting results when the list sorting changes. Modify the JavaScript code as follows:
$(document).ready(function() { $("#sortable").sortable({ update: function(event, ui) { var sortedIDs = $(this).sortable("toArray"); console.log(sortedIDs); } }); });
In this code, we use jQuery UI’s toArray()
method to get the ID of the sorted list item and print it to the console . You can further process the sorting results according to actual needs, such as saving the sorting results or updating the display of the interface.
Finally, in order to increase interactivity, we can add some animation effects when sorting. Modify the JavaScript code as follows:
$(document).ready(function() { $("#sortable").sortable({ update: function(event, ui) { var sortedIDs = $(this).sortable("toArray"); console.log(sortedIDs); } }).disableSelection(); });
In this code, we use jQuery UI's disableSelection()
method to prevent the text of the list item from being selected.
So far, we have completed the implementation of a simple drag-and-drop sorting function. You can adjust the style and expand functions according to your own needs, such as adding deletion, editing and other functions.
Summary:
This article provides an example of using HTML, CSS and jQuery to implement drag-and-drop sorting. By using jQuery UI's sortable()
method, we can easily implement drag-and-drop sorting of the list, and after the sorting is completed, we can easily obtain the sorted results. I hope this article will help you understand the implementation process of drag and drop sorting.
Code example: https://codepen.io/pen/?template=WNRZebr
The above is the detailed content of HTML, CSS, and jQuery: A technical guide to implementing drag-and-drop sorting. For more information, please follow other related articles on the PHP Chinese website!
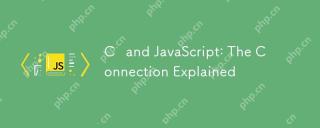
C and JavaScript achieve interoperability through WebAssembly. 1) C code is compiled into WebAssembly module and introduced into JavaScript environment to enhance computing power. 2) In game development, C handles physics engines and graphics rendering, and JavaScript is responsible for game logic and user interface.
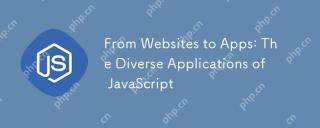
JavaScript is widely used in websites, mobile applications, desktop applications and server-side programming. 1) In website development, JavaScript operates DOM together with HTML and CSS to achieve dynamic effects and supports frameworks such as jQuery and React. 2) Through ReactNative and Ionic, JavaScript is used to develop cross-platform mobile applications. 3) The Electron framework enables JavaScript to build desktop applications. 4) Node.js allows JavaScript to run on the server side and supports high concurrent requests.
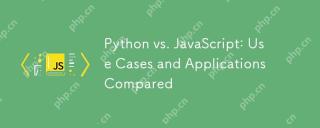
Python is more suitable for data science and automation, while JavaScript is more suitable for front-end and full-stack development. 1. Python performs well in data science and machine learning, using libraries such as NumPy and Pandas for data processing and modeling. 2. Python is concise and efficient in automation and scripting. 3. JavaScript is indispensable in front-end development and is used to build dynamic web pages and single-page applications. 4. JavaScript plays a role in back-end development through Node.js and supports full-stack development.
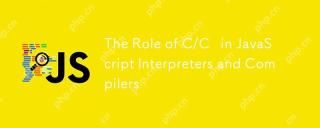
C and C play a vital role in the JavaScript engine, mainly used to implement interpreters and JIT compilers. 1) C is used to parse JavaScript source code and generate an abstract syntax tree. 2) C is responsible for generating and executing bytecode. 3) C implements the JIT compiler, optimizes and compiles hot-spot code at runtime, and significantly improves the execution efficiency of JavaScript.
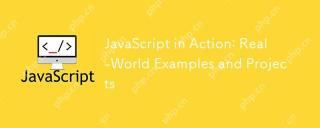
JavaScript's application in the real world includes front-end and back-end development. 1) Display front-end applications by building a TODO list application, involving DOM operations and event processing. 2) Build RESTfulAPI through Node.js and Express to demonstrate back-end applications.
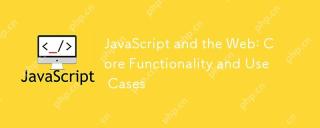
The main uses of JavaScript in web development include client interaction, form verification and asynchronous communication. 1) Dynamic content update and user interaction through DOM operations; 2) Client verification is carried out before the user submits data to improve the user experience; 3) Refreshless communication with the server is achieved through AJAX technology.
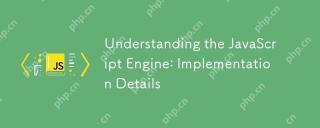
Understanding how JavaScript engine works internally is important to developers because it helps write more efficient code and understand performance bottlenecks and optimization strategies. 1) The engine's workflow includes three stages: parsing, compiling and execution; 2) During the execution process, the engine will perform dynamic optimization, such as inline cache and hidden classes; 3) Best practices include avoiding global variables, optimizing loops, using const and lets, and avoiding excessive use of closures.

Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

Atom editor mac version download
The most popular open source editor
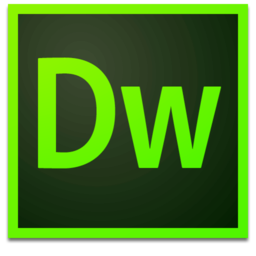
Dreamweaver Mac version
Visual web development tools

SublimeText3 Linux new version
SublimeText3 Linux latest version