The encapsulated concurrent programming technology in PHP requires specific code examples
With the rapid development of the Internet, there are more and more demands for high-concurrency applications. As a commonly used server-side programming language, PHP has gradually begun to get involved in the field of concurrent programming. In concurrent programming, encapsulation is an important technology that can help us better manage and control concurrent operations.
Encapsulation refers to encapsulating a piece of functional code into an independent unit to achieve specific functions and be able to adapt to different concurrent operation requirements. In PHP, we can use a variety of ways to implement encapsulated concurrent programming technology. Below I will give a few commonly used examples.
- Use multiple processes to implement concurrent operations
In PHP, you can use the pcntl extension to implement multi-process concurrent operations. The following is a sample code:
<?php $workers = []; $workerNum = 5; for ($i = 0; $i < $workerNum; $i++) { $pid = pcntl_fork(); if ($pid == -1) { die("Fork failed"); } else if ($pid == 0) { // worker process // do some work exit(); } else { // parent process $workers[] = $pid; } } foreach ($workers as $pid) { pcntl_waitpid($pid); } ?>
The above code uses the pcntl_fork function to create 5 sub-processes. Each sub-process can perform an independent concurrent operation. This method can easily process a large number of tasks in parallel and improve the execution efficiency of the code.
- Use multi-threading to implement concurrent operations
In PHP, you can use the pthread extension to implement multi-threaded concurrent operations. The following is a sample code:
<?php class MyThread extends Thread { public function run() { // do some work } } $threads = []; $threadNum = 5; for ($i = 0; $i < $threadNum; $i++) { $thread = new MyThread(); $thread->start(); $threads[] = $thread; } foreach ($threads as $thread) { $thread->join(); } ?>
The above code uses the pthread class to create 5 threads, each thread can perform an independent concurrent operation. The advantage of using multi-threading is that it can share memory and manage and share resources more efficiently.
- Use coroutines to implement concurrent operations
In PHP, you can use the Swoole extension to implement coroutines concurrent operations. The following is a sample code:
<?php $coroutine = new Coroutine(); for($i = 0; $i < 5; $i++) { $coroutine->create(function() { // do some work }); } ?>
The above code uses the Coroutine class provided by Swoole to create 5 coroutines. Each coroutine can perform an independent concurrent operation. Coroutines are a lightweight concurrency model that can effectively improve the concurrent processing capabilities of programs.
Through the above example code, we can see that it is very simple to implement encapsulated concurrent programming technology in PHP. These technologies can help us better manage and control concurrent operations and improve the concurrent processing capabilities of the program. Whether using multi-process, multi-thread or coroutine, we can choose the appropriate method according to specific needs to achieve efficient concurrent programming.
The above is the detailed content of Encapsulated concurrent programming technology in PHP. For more information, please follow other related articles on the PHP Chinese website!
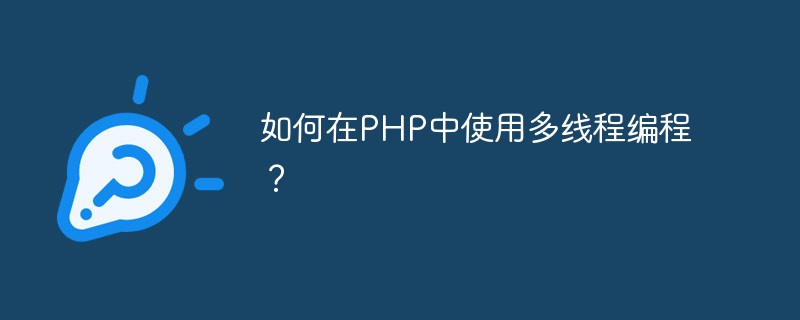
随着Web应用程序变得越来越庞大和复杂,传统的单线程PHP开发模式不再适用于高并发处理。在这种情况下,使用多线程技术可以提高Web应用程序处理并发请求的能力。本文将介绍如何在PHP中使用多线程编程。一、多线程概述多线程编程是指在一个进程中并发执行多个线程,每个线程都能单独访问进程中的共享内存和资源。多线程技术可以提高CPU和内存的使用效率,同时可以处理更多的
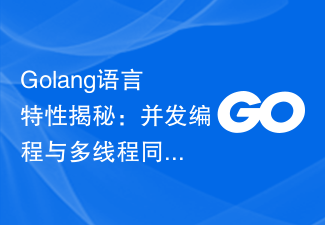
Golang语言特性揭秘:并发编程与多线程同步Golang是一种现代化的编程语言,被设计用于解决大规模并发问题。它的并发编程模型让开发人员可以轻松地创建并管理多个goroutine,实现高效的并发执行。在本文中,我们将揭秘Golang的并发编程特性,并探讨如何在多线程中进行同步。Golang的并发编程模型基于goroutine和channel。gorouti
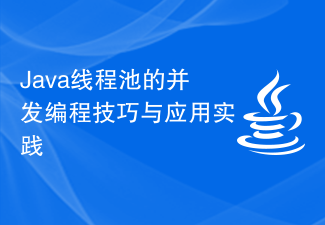
Java线程池的并发编程技巧与应用实践随着互联网和移动互联网的普及,并发访问量变得越来越大,传统单线程编程方式已经无法满足大规模并发的需求。Java线程池充分利用CPU资源,实现高效并发编程,是面向对象编程中不可或缺的一部分。本文从Java线程池的基本原理入手,介绍了线程池的核心参数配置、使用方法、线程池的应用场景及其优化策略。一、Java线程池基本原理J
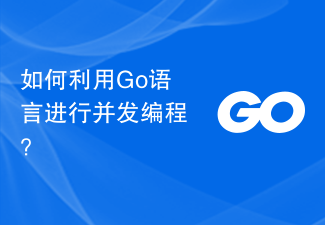
随着计算机硬件的不断发展,处理器中的CPU核心不再单独增加时钟频率,而是增加核心数量。这引发了一个显而易见的问题:如何发挥这些核心的性能?一种解决方法是通过并行编程,即同时执行多个任务,以充分利用CPU核心。这就是Go语言的一个独特之处,它是一门专为并发编程而设计的语言。在本文中,我们将探讨如何利用Go语言进行并发编程。协程首先,我们需要了解
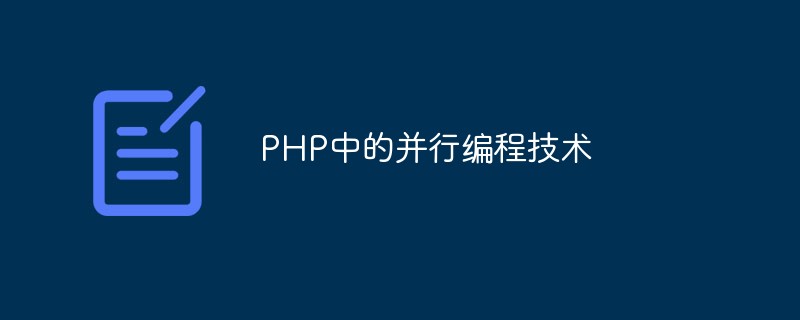
随着互联网的快速发展,大型Web应用程序的开发变得越来越流行。在这种情况下,一个Web应用程序需要处理来自数百甚至数千个用户的请求。这就需要并行编程技术,以便在处理多个请求时提高程序的性能。PHP是一个流行的脚本语言,广泛用于Web应用程序的开发。PHP提供了多种并行编程技术,包括多进程、多线程和异步编程。在本文中,我们会介绍这些技术,以及它们如何帮助我们实
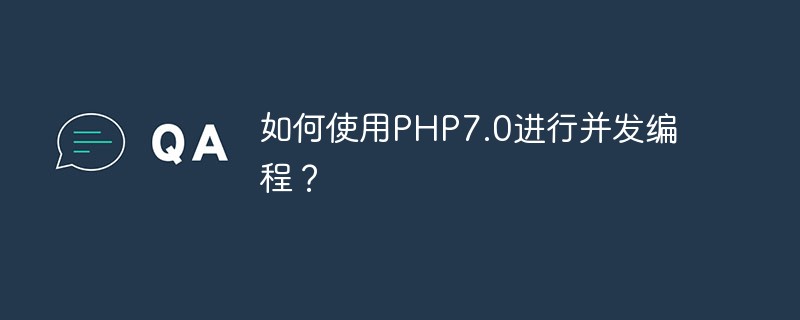
PHP7.0是当前最常用的服务器端编程语言之一。它界面友好、易于学习,功能强大,具有丰富的扩展库。在并发编程方面,PHP7.0也有许多优秀的工具和技术。本文将介绍如何在PHP7.0中进行并发编程。一、什么是并发编程并发编程是指通过多个线程,进程或协程等方式,使多个任务在同一时间内同时执行的编程方式。在编程中,有效地使用并发技术可以提高程序的性能和吞吐量。二、
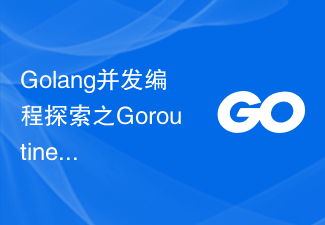
Golang并发编程探索之Goroutines的线程模型详解在当今互联网时代,高并发成为了各种系统开发中非常重要的一个课题。传统的单线程编程模型很难以满足大量并发请求的需求,而在很多编程语言中,多线程编程也存在着复杂的竞态条件、死锁等问题。而在Golang中,通过轻量级的Goroutines和基于通信的并发模型,使得并发编程变得更加简单和高效。Gorouti
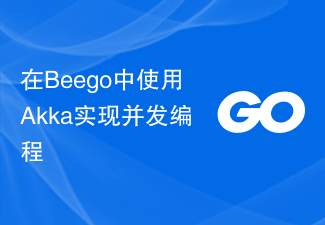
随着互联网的不断发展,高并发和分布式是大多数Web应用程序所面临的挑战。许多框架和工具已经被开发出来,以便用于解决这些挑战,而在这些框架和工具中,Beego和Akka是非常好的例子。Beego是一个开源的Web应用框架,而Akka是一个强大的并发编程框架,它能够使分布式应用程序更容易开发和维护。本文将介绍如何在Beego中使用Akka来实现并发编程。一、A


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor
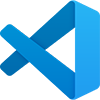
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SublimeText3 Linux new version
SublimeText3 Linux latest version

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
