PHP sessions are server-side, while cookies are client-side. 1) Sessions store data on the server, are more secure, and handle larger data. 2) Cookies store data on the client, are less secure, and limited in size. Use sessions for sensitive data and cookies for non-sensitive, client-side data.
PHP sessions and cookies, while often used together, serve different purposes and have distinct characteristics. Let's dive into the nuances of each, explore their differences, and share some real-world insights on when to use one over the other.
PHP sessions are a server-side mechanism for maintaining user data across multiple page requests. When a user interacts with your PHP application, a unique session ID is generated and stored on the server. This session ID is typically sent to the client as a cookie, but it's the server that holds the actual data. Here's a quick look at how sessions work in PHP:
// Start the session session_start(); // Store data in the session $_SESSION['username'] = 'john_doe'; // Access the stored data echo $_SESSION['username']; // Outputs: john_doe
On the other hand, cookies are client-side storage mechanisms. They store data directly on the user's browser and can be sent back to the server with each request. Here's how you might set and retrieve a cookie in PHP:
// Set a cookie setcookie('username', 'john_doe', time() 3600, '/'); // Retrieve the cookie if (isset($_COOKIE['username'])) { echo $_COOKIE['username']; // Outputs: john_doe }
Now, let's break down the key differences between PHP sessions and cookies:
Storage Location: Sessions are stored on the server, while cookies are stored on the client's browser. This means sessions are more secure because the data never leaves the server, but cookies can be more convenient for storing non-sensitive data.
Data Size: Sessions can handle larger amounts of data compared to cookies, which are limited in size (typically around 4KB). If you need to store a lot of information, sessions are the way to go.
Security: Sessions are generally more secure since the data is not accessible to the client. However, the session ID sent as a cookie can be vulnerable to session hijacking if not properly secured. Cookies, on the other hand, are more exposed and can be tampered with or stolen.
Expiration: Sessions expire when the user closes their browser or when the session timeout is reached (default is 24 minutes in PHP). Cookies can be set to expire at a specific time, allowing for longer-term data storage.
Accessibility: Sessions are only accessible by the server-side script that created them, whereas cookies can be accessed and modified by client-side scripts (like JavaScript), which can be both a benefit and a security risk.
From my experience, choosing between sessions and cookies often depends on the specific needs of your application. Here are some insights and best practices:
Use Sessions for Sensitive Data: If you're dealing with user authentication or any sensitive information, sessions are the safer choice. I've worked on projects where we used sessions to store user IDs and other critical data, ensuring it never left the server.
Use Cookies for Non-Sensitive, Client-Side Data: For things like user preferences or remembering the last visited page, cookies are perfect. They're lightweight and can be easily managed on the client side. I once implemented a feature where we stored user-selected themes in cookies, making the application more responsive without server requests.
Hybrid Approach: In some cases, using both sessions and cookies can be beneficial. For instance, you might store a session ID in a cookie to maintain the user's session, but keep all sensitive data in the session itself. This approach balances security with convenience.
Be Aware of Session Limitations: While sessions are powerful, they can become a bottleneck if not managed properly. I've seen applications slow down due to too many active sessions. Implementing session garbage collection and using session handlers like Memcached can help mitigate these issues.
Cookie Security: If you do use cookies, make sure to set the
HttpOnly
andSecure
flags to enhance security. These flags prevent client-side scripts from accessing the cookies and ensure they're only sent over HTTPS, respectively.
In terms of pitfalls to watch out for:
Session Fixation: This is a common vulnerability where an attacker can fixate a session ID on a user's browser before they log in. Always regenerate the session ID after a successful login to prevent this.
Cookie Tampering: Since cookies are stored on the client side, they can be tampered with. Use techniques like encryption or digital signatures to ensure the integrity of the data stored in cookies.
Performance: Both sessions and cookies can impact performance if not used judiciously. Sessions require server resources, and too many cookies can slow down page loads. Monitor your application's performance and optimize accordingly.
In conclusion, understanding the differences between PHP sessions and cookies is crucial for building secure and efficient web applications. By leveraging the strengths of each and being aware of their limitations, you can make informed decisions that enhance your application's functionality and security.
The above is the detailed content of How do PHP sessions differ from cookies?. For more information, please follow other related articles on the PHP Chinese website!
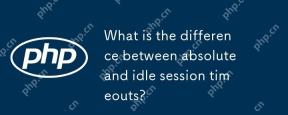
Absolute session timeout starts at the time of session creation, while an idle session timeout starts at the time of user's no operation. Absolute session timeout is suitable for scenarios where strict control of the session life cycle is required, such as financial applications; idle session timeout is suitable for applications that want users to keep their session active for a long time, such as social media.
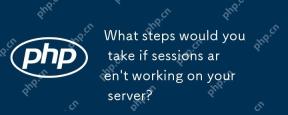
The server session failure can be solved through the following steps: 1. Check the server configuration to ensure that the session is set correctly. 2. Verify client cookies, confirm that the browser supports it and send it correctly. 3. Check session storage services, such as Redis, to ensure that they are running normally. 4. Review the application code to ensure the correct session logic. Through these steps, conversation problems can be effectively diagnosed and repaired and user experience can be improved.
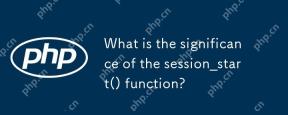
session_start()iscrucialinPHPformanagingusersessions.1)Itinitiatesanewsessionifnoneexists,2)resumesanexistingsession,and3)setsasessioncookieforcontinuityacrossrequests,enablingapplicationslikeuserauthenticationandpersonalizedcontent.
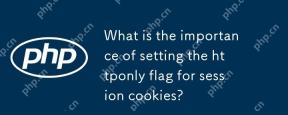
Setting the httponly flag is crucial for session cookies because it can effectively prevent XSS attacks and protect user session information. Specifically, 1) the httponly flag prevents JavaScript from accessing cookies, 2) the flag can be set through setcookies and make_response in PHP and Flask, 3) Although it cannot be prevented from all attacks, it should be part of the overall security policy.
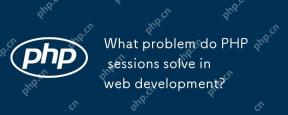
PHPsessionssolvetheproblemofmaintainingstateacrossmultipleHTTPrequestsbystoringdataontheserverandassociatingitwithauniquesessionID.1)Theystoredataserver-side,typicallyinfilesordatabases,anduseasessionIDstoredinacookietoretrievedata.2)Sessionsenhances
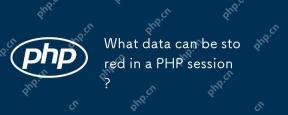
PHPsessionscanstorestrings,numbers,arrays,andobjects.1.Strings:textdatalikeusernames.2.Numbers:integersorfloatsforcounters.3.Arrays:listslikeshoppingcarts.4.Objects:complexstructuresthatareserialized.
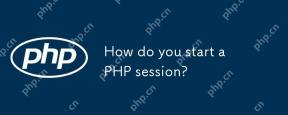
TostartaPHPsession,usesession_start()atthescript'sbeginning.1)Placeitbeforeanyoutputtosetthesessioncookie.2)Usesessionsforuserdatalikeloginstatusorshoppingcarts.3)RegeneratesessionIDstopreventfixationattacks.4)Considerusingadatabaseforsessionstoragei
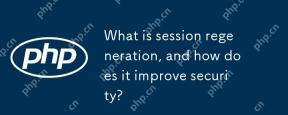
Session regeneration refers to generating a new session ID and invalidating the old ID when the user performs sensitive operations in case of session fixed attacks. The implementation steps include: 1. Detect sensitive operations, 2. Generate new session ID, 3. Destroy old session ID, 4. Update user-side session information.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

Zend Studio 13.0.1
Powerful PHP integrated development environment
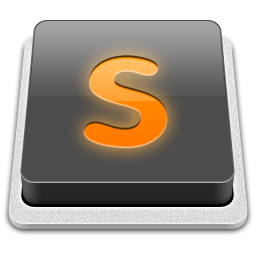
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SublimeText3 Linux new version
SublimeText3 Linux latest version

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
