


Absolute session timeout starts at the time of session creation, while an idle session timeout starts at the time of user's no operation. Absolute session timeout is suitable for scenarios where strict control of the session life cycle is required, such as financial applications; idle session timeout is suitable for applications that want users to keep their session active for a long time, such as social media.
introduction
It is crucial to understand the difference between absolute session timeout and idle session timeout when handling user sessions. These concepts not only affect user experience, but also involve security and resource management. Through this article, you will gain an in-depth understanding of the definitions, how these two timeout mechanisms work, and best practices in practical applications. I will combine my personal experience to share some interesting cases and common misunderstandings to help you better understand and apply these concepts.
Review of basic knowledge
Session timeout is a common concept in web applications and is used to manage the life cycle of user sessions. Session timeouts can be divided into two types: absolute session timeout and idle session timeout. Session management is an important part of ensuring application security and performance. It involves technologies such as HTTP sessions, cookies, and server-side session storage.
Core concept or function analysis
Definition and function of absolute session timeout
Absolute session timeout refers to the timeout starting from session creation, and the session terminates once the set timeout is reached, regardless of whether the user is active or not. This mechanism is often used in scenarios where strict control of the session life cycle is required, such as financial applications or systems with high security requirements.
The function of absolute session timeout is to ensure that even if the user forgets to log out, the session will automatically terminate after a certain period of time, reducing security risks. For example, in a banking application, the session will automatically end after 30 minutes regardless of whether there is an operation or not after the user logs in.
// Absolute session timeout example (Java Servlet) session.setMaxInactiveInterval(1800); // Set the absolute session timeout to 30 minutes
Definition and function of idle session timeout
The idle session timeout starts when the user has no operation. If there is no user activity within the set time, the session will be terminated. This mechanism is more suitable for applications that want users to keep their conversations active for a long time, such as social media or email services.
The function of idle session timeout is to keep the user's session active and at the same time release resources when the user is inactive for a long time. For example, on a blog platform, a user may want to stay logged in for several hours, but if there is no operation for more than 1 hour, the session will be terminated.
// Idle session timeout example (Java Servlet) session.setMaxInactiveInterval(3600); // Set the idle session timeout to 1 hour
How it works
Absolute session timeout works by a timer based on session creation time. Once the session is created, the timer starts counting down until the set timeout time is reached. This method is simple and direct, but may cause the user to be forced to exit the session if he does not operate for a long time.
The working principle of idle session timeout is based on a timer for user activity. Whenever the user performs any action (such as clicks, inputs, etc.), the timer resets and restarts the timing. This method is more flexible and can manage sessions based on the actual activity status of the user, but requires more server resources to monitor user activity.
Example of usage
Basic usage
In actual applications, setting an absolute session timeout is very simple, just set the timeout time when the session is created. For example, in a Java Servlet, you can use setMaxInactiveInterval
method to set an absolute session timeout.
// Set HttpSession session = request.getSession(); session.setMaxInactiveInterval(1800); // 30 minutes
Setting up an idle session timeout is just as simple as setting the timeout time when the session is created and making sure the timer is reset every time the user operates.
// Set HttpSession session = request.getSession(); session.setMaxInactiveInterval(3600); // 1 hour
Advanced Usage
In some cases, it may be necessary to dynamically adjust the timeout based on the user role or session type. For example, in an enterprise application, the administrator's session timeout may be longer than the average user.
// Dynamically set the session timeout HttpSession session = request.getSession(); if (user.isAdmin()) { session.setMaxInactiveInterval(7200); // Administrator 2 hours} else { session.setMaxInactiveInterval(3600); // 1 hour for ordinary users}
Another advanced usage is to combine session timeout and periodic heartbeat mechanisms to keep the session active. For example, in a real-time collaboration application, the session can be prevented from timeout due to long periods of non-operation by sending a heartbeat signal regularly.
// Example of heartbeat mechanism function sendHeartbeat() { $.ajax({ type: "POST", url: "/heartbeat", success: function() { console.log("Heartbeat sent"); } }); } <p>setInterval(sendHeartbeat, 300000); // Send heartbeat every 5 minutes</p>
Common Errors and Debugging Tips
A common mistake is to misunderstand the difference between absolute session timeout and idle session timeout, resulting in improper settings. For example, after setting an absolute session timeout, the user may be forced to exit the session when there is no operation for a long time, affecting the user experience.
One of the debugging tips is to monitor session timeout events through logging. For example, logs can be recorded when the session timeout for subsequent analysis and optimization.
// Log session timeout log session.setAttribute("timeoutListener", new HttpSessionListener() { @Override public void sessionDestroyed(HttpSessionEvent se) { logger.info("Session timed out: " se.getSession().getId()); } });
Performance optimization and best practices
In practical applications, optimizing session timeout settings can improve system performance and user experience. One way is to dynamically adjust the session timeout based on the frequency of user activity. For example, if the user performs multiple operations in a short time, the session timeout can be appropriately extended.
// Dynamically adjust the session timeout HttpSession session = request.getSession(); if (user.getRecentActivityCount() > 10) { session.setMaxInactiveInterval(7200); // 2 hours} else { session.setMaxInactiveInterval(3600); // 1 hour}
Another best practice is to combine session timeout and load balancing policies to ensure that sessions can be effectively managed under high load conditions. For example, session stickiness can be set on the load balancer to ensure that the user's session is processed on the same server.
// Session stickiness settings (Nginx example) http { upstream backend { ip_hash; // Enable session stickiness server backend1.example.com; server backend2.example.com; } }
In general, absolute session timeout and idle session timeout have their own advantages and disadvantages. Which mechanism to choose should be determined based on the specific needs of the application and user experience. In practical applications, flexibly combining these two mechanisms and dynamically adjusting and optimizing strategies can maximize the security and performance of the system.
The above is the detailed content of What is the difference between absolute and idle session timeouts?. For more information, please follow other related articles on the PHP Chinese website!
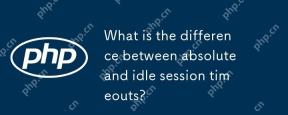
Absolute session timeout starts at the time of session creation, while an idle session timeout starts at the time of user's no operation. Absolute session timeout is suitable for scenarios where strict control of the session life cycle is required, such as financial applications; idle session timeout is suitable for applications that want users to keep their session active for a long time, such as social media.
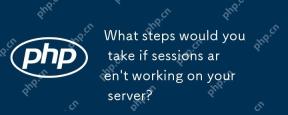
The server session failure can be solved through the following steps: 1. Check the server configuration to ensure that the session is set correctly. 2. Verify client cookies, confirm that the browser supports it and send it correctly. 3. Check session storage services, such as Redis, to ensure that they are running normally. 4. Review the application code to ensure the correct session logic. Through these steps, conversation problems can be effectively diagnosed and repaired and user experience can be improved.
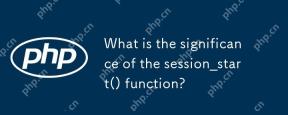
session_start()iscrucialinPHPformanagingusersessions.1)Itinitiatesanewsessionifnoneexists,2)resumesanexistingsession,and3)setsasessioncookieforcontinuityacrossrequests,enablingapplicationslikeuserauthenticationandpersonalizedcontent.
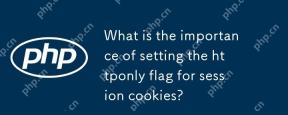
Setting the httponly flag is crucial for session cookies because it can effectively prevent XSS attacks and protect user session information. Specifically, 1) the httponly flag prevents JavaScript from accessing cookies, 2) the flag can be set through setcookies and make_response in PHP and Flask, 3) Although it cannot be prevented from all attacks, it should be part of the overall security policy.
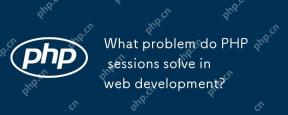
PHPsessionssolvetheproblemofmaintainingstateacrossmultipleHTTPrequestsbystoringdataontheserverandassociatingitwithauniquesessionID.1)Theystoredataserver-side,typicallyinfilesordatabases,anduseasessionIDstoredinacookietoretrievedata.2)Sessionsenhances
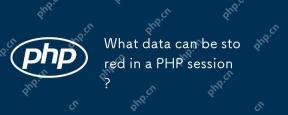
PHPsessionscanstorestrings,numbers,arrays,andobjects.1.Strings:textdatalikeusernames.2.Numbers:integersorfloatsforcounters.3.Arrays:listslikeshoppingcarts.4.Objects:complexstructuresthatareserialized.
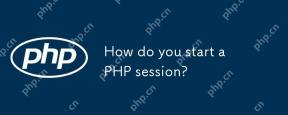
TostartaPHPsession,usesession_start()atthescript'sbeginning.1)Placeitbeforeanyoutputtosetthesessioncookie.2)Usesessionsforuserdatalikeloginstatusorshoppingcarts.3)RegeneratesessionIDstopreventfixationattacks.4)Considerusingadatabaseforsessionstoragei
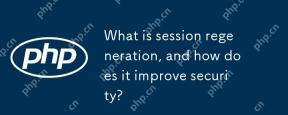
Session regeneration refers to generating a new session ID and invalidating the old ID when the user performs sensitive operations in case of session fixed attacks. The implementation steps include: 1. Detect sensitive operations, 2. Generate new session ID, 3. Destroy old session ID, 4. Update user-side session information.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
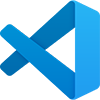
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
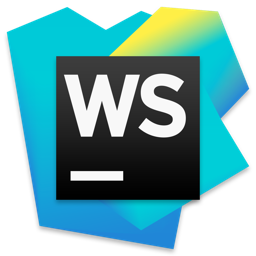
WebStorm Mac version
Useful JavaScript development tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SublimeText3 Linux new version
SublimeText3 Linux latest version
