session_start() is crucial in PHP for managing user sessions. 1) It initiates a new session if none exists, 2) resumes an existing session, and 3) sets a session cookie for continuity across requests, enabling applications like user authentication and personalized content.
The session_start()
function is a cornerstone in web development, particularly when dealing with user sessions in PHP. Its significance lies in its ability to initialize or resume a session, allowing you to store and retrieve data across multiple page requests. Let's dive into why this function is crucial and how it shapes the landscape of web applications.
The Essence of session_start()
In the wild world of web development, where statelessness reigns supreme, session_start()
is your trusty companion for maintaining state. When you call this function, it does a few magical things:
- Initiates a Session: If no session exists, it creates one, assigning a unique session ID to the user.
- Resumes a Session: If a session already exists, it retrieves the session data associated with the current session ID.
- Sets the Session Cookie: It sends a cookie to the user's browser with the session ID, ensuring continuity across requests.
Here's a simple yet elegant example of how you might use it:
<?php session_start(); // Store data in the session $_SESSION['username'] = 'JohnDoe'; // Retrieve data from the session echo $_SESSION['username']; // Outputs: JohnDoe ?>
The Inner Workings
Understanding how session_start()
operates under the hood can give you a deeper appreciation for its role. When you call this function, PHP checks for a session cookie in the user's request. If found, it uses the session ID to locate the corresponding session data on the server. If no session exists, PHP generates a new session ID and initializes a new session.
This process is crucial for maintaining user-specific data, like login information, shopping carts, or any other data that needs to persist across page loads. Without session_start()
, you'd be reinventing the wheel every time you need to keep track of user state.
Practical Applications and Pitfalls
In my journey through the coding wilderness, I've found session_start()
to be indispensable for various applications:
- User Authentication: It's the backbone of keeping users logged in across different pages.
- Shopping Carts: It allows you to store items in a user's cart without losing them between visits.
- Personalized Content: It enables you to tailor content based on user preferences or history.
However, there are pitfalls to watch out for:
- Session Hijacking: If not secured properly, session IDs can be stolen, leading to unauthorized access.
- Session Fixation: An attacker might fixate a session ID on a user before they log in, gaining access to their session after login.
- Performance Overhead: Managing sessions can add overhead, especially with large-scale applications.
To mitigate these risks, always use HTTPS, regenerate session IDs after login, and consider using session handlers like Redis for better performance.
Optimizing and Best Practices
When wielding session_start()
, consider these tips to optimize your code and follow best practices:
-
Use it Early: Call
session_start()
at the beginning of your script to ensure session data is available throughout. - Clean Up: Regularly clean up old sessions to prevent server bloat.
-
Secure Your Sessions: Use
session_regenerate_id()
after login to prevent session fixation attacks.
Here's an example of how you might implement these practices:
<?php // Start the session at the beginning of the script session_start(); // Regenerate session ID after login to prevent session fixation if (isset($_POST['login'])) { // Login logic here session_regenerate_id(true); } // Store user data $_SESSION['user_id'] = 123; // Clean up old sessions if (rand(1, 100) === 1) { // 1% chance to run $maxlifetime = ini_get("session.gc_maxlifetime"); foreach (glob(session_save_path() . '/*') as $file) { if (filemtime($file) $maxlifetime < time() && file_exists($file)) { unlink($file); } } } ?>
Wrapping Up
The session_start()
function is more than just a line of code; it's a gateway to managing user state in a stateless environment. By understanding its significance, you can harness its power to create more dynamic, user-friendly web applications. Just remember to tread carefully, securing your sessions and optimizing their use to avoid common pitfalls. Happy coding!
The above is the detailed content of What is the significance of the session_start() function?. For more information, please follow other related articles on the PHP Chinese website!
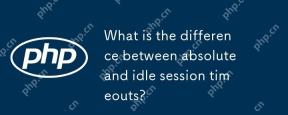
Absolute session timeout starts at the time of session creation, while an idle session timeout starts at the time of user's no operation. Absolute session timeout is suitable for scenarios where strict control of the session life cycle is required, such as financial applications; idle session timeout is suitable for applications that want users to keep their session active for a long time, such as social media.
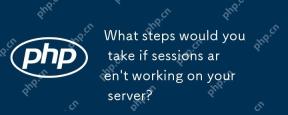
The server session failure can be solved through the following steps: 1. Check the server configuration to ensure that the session is set correctly. 2. Verify client cookies, confirm that the browser supports it and send it correctly. 3. Check session storage services, such as Redis, to ensure that they are running normally. 4. Review the application code to ensure the correct session logic. Through these steps, conversation problems can be effectively diagnosed and repaired and user experience can be improved.
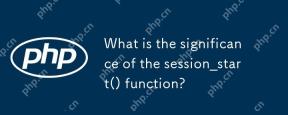
session_start()iscrucialinPHPformanagingusersessions.1)Itinitiatesanewsessionifnoneexists,2)resumesanexistingsession,and3)setsasessioncookieforcontinuityacrossrequests,enablingapplicationslikeuserauthenticationandpersonalizedcontent.
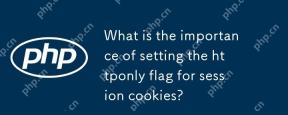
Setting the httponly flag is crucial for session cookies because it can effectively prevent XSS attacks and protect user session information. Specifically, 1) the httponly flag prevents JavaScript from accessing cookies, 2) the flag can be set through setcookies and make_response in PHP and Flask, 3) Although it cannot be prevented from all attacks, it should be part of the overall security policy.
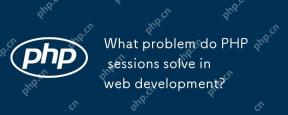
PHPsessionssolvetheproblemofmaintainingstateacrossmultipleHTTPrequestsbystoringdataontheserverandassociatingitwithauniquesessionID.1)Theystoredataserver-side,typicallyinfilesordatabases,anduseasessionIDstoredinacookietoretrievedata.2)Sessionsenhances
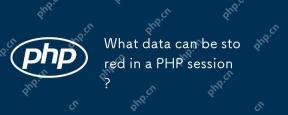
PHPsessionscanstorestrings,numbers,arrays,andobjects.1.Strings:textdatalikeusernames.2.Numbers:integersorfloatsforcounters.3.Arrays:listslikeshoppingcarts.4.Objects:complexstructuresthatareserialized.
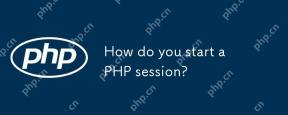
TostartaPHPsession,usesession_start()atthescript'sbeginning.1)Placeitbeforeanyoutputtosetthesessioncookie.2)Usesessionsforuserdatalikeloginstatusorshoppingcarts.3)RegeneratesessionIDstopreventfixationattacks.4)Considerusingadatabaseforsessionstoragei
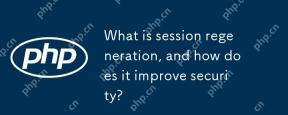
Session regeneration refers to generating a new session ID and invalidating the old ID when the user performs sensitive operations in case of session fixed attacks. The implementation steps include: 1. Detect sensitive operations, 2. Generate new session ID, 3. Destroy old session ID, 4. Update user-side session information.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
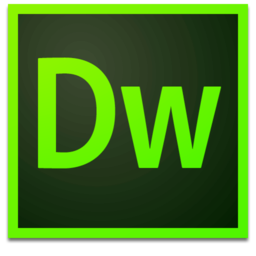
Dreamweaver Mac version
Visual web development tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
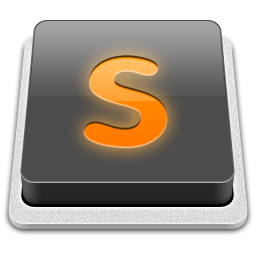
SublimeText3 Mac version
God-level code editing software (SublimeText3)
