


What are some performance considerations when using PHP sessions?
PHP sessions have a significant impact on application performance. Optimization methods include: 1. Use a database to store session data to improve response speed; 2. Reduce the use of session data and only store necessary information; 3. Use a non-blocking session processor to improve concurrency capabilities; 4. Adjust the session expiration time to balance user experience and server burden; 5. Use persistent sessions to reduce the number of data read and write times.
introduction
Before exploring performance considerations for PHP sessions, let’s first think about one question: Do you really understand the impact they have on your application’s performance when using PHP sessions? PHP sessions are a commonly used mechanism in web development, which are used to store and manage data between different requests of users. However, correctly understanding and optimizing session usage is critical to improving application responsiveness and scalability. In this article, we will dig into the performance considerations of PHP sessions, from basic knowledge to best practices in real-world applications, hoping to help you better understand and optimize your PHP applications.
Review of basic knowledge
PHP sessions are implemented by storing data on the server, usually using cookies or URL rewrites to track the user's session ID. These data can be user login information, shopping cart content, etc. Session data is usually stored in the server's file system, but can also be configured to use a database or other storage mechanism.
The working principle of a session is that when a user first accesses your application, PHP generates a unique session ID and sends this ID to the client (usually via cookies). In subsequent requests, PHP will read session data from the server based on this ID.
Core concept or function analysis
Definition and function of PHP session
A PHP session is a mechanism that allows you to store and access data between multiple requests from a user. Its main function is to maintain the user's status, such as login status, shopping cart content, etc. The use of sessions can greatly simplify the development of web applications because it provides an easy way to manage user data.
A simple session usage example:
<?php session_start(); <p>// Set the session variable $_SESSION['username'] = 'example_user';<p> // Read the session variable echo $_SESSION['username']; ?></p>
This example shows how to start a session, set and read session variables.
How it works
When you call session_start()
, PHP will check whether there is a valid session ID. If present, it will read the corresponding session data from the server. If it does not exist, PHP generates a new session ID and creates a new session file.
Session data is usually stored in the server's file system, and the default path is the /tmp
directory. PHP reads and writes the session file every time it is requested, which may have a performance impact.
Example of usage
Basic usage
The most common way to use the session is to store and read data through the $_SESSION
hyperglobal array:
<?php session_start(); <p>// Set the session variable $_SESSION['user_id'] = 123;<p> // Read the session variable $user_id = $_SESSION['user_id']; ?></p>
This example shows how to store and read a user ID in a session.
Advanced Usage
In some cases, you may need to use a custom session processor to optimize performance. For example, you can store session data in a database instead of a file system:
<?php class CustomSessionHandler implements SessionHandlerInterface { private $db; <pre class='brush:php;toolbar:false;'>public function __construct($db) { $this->db = $db; } public function open($save_path, $name) { return true; } public function close() { return true; } public function read($id) { $stmt = $this->db->prepare("SELECT data FROM sessions WHERE id = ?"); $stmt->execute([$id]); $result = $stmt->fetch(); return $result ? $result['data'] : ''; } public function write($id, $data) { $stmt = $this->db->prepare("REPLACE INTO sessions (id, data) VALUES (?, ?)"); return $stmt->execute([$id, $data]); } // Other methods to implement...
}
$db = new PDO('mysql:host=localhost;dbname=example', 'username', 'password'); $handler = new CustomSessionHandler($db); session_set_save_handler($handler, true); session_start(); ?>
This example shows how to implement a custom session processor that stores session data in a database.
Common Errors and Debugging Tips
- Session file locking issue : Session file locking may cause performance problems in high concurrency environments. You can solve this problem by using a non-blocking session processor or reducing the use of session files.
- Too large session data : If the session data is too large, it will increase the time to read and write to the session file. You can optimize by limiting the size of session data or using a more efficient storage mechanism.
- Session Expiration Problem : Incorrect session expiration time setting may cause users to log in frequently. You can solve this problem by adjusting the session expiration time or using a persistent session.
Performance optimization and best practices
In practical applications, the following aspects need to be considered for optimizing the performance of PHP sessions:
- Use a database to store session data : Databases can provide better performance and scalability than file systems. Using a database to store session data can reduce I/O operations in the file system and improve application response speed.
- Reduce the use of session data : minimize the use of session data and store only necessary information. Too much session data will increase the time for reading and writing, affecting performance.
- Using a non-blocking session processor : In a high concurrency environment, using a non-blocking session processor can avoid session file locking problems and improve the concurrency capabilities of the application.
- Adjust the session expiration time : Set the session expiration time reasonably according to the application's needs. An expiration time of too short will cause users to log in frequently, affecting the user experience; an expiration time of too long will increase the burden on the server.
- Using persistent sessions : In some cases, using persistent sessions can reduce the number of reads and writes of session data and improve performance.
In my actual project experience, I once encountered an e-commerce application. Due to the large session data, the application response speed became slower. We have successfully optimized the performance of our application by storing the session data in Redis and reducing the use of session data. This experience tells me that optimizing the use of a session requires comprehensive consideration of many factors and cannot simply rely on the default session processing mechanism.
In short, performance optimization of PHP sessions is a complex process that requires the formulation of appropriate strategies based on specific application scenarios and requirements. I hope this article can provide you with some useful insights and practical experience to help you better optimize your PHP application.
The above is the detailed content of What are some performance considerations when using PHP sessions?. For more information, please follow other related articles on the PHP Chinese website!
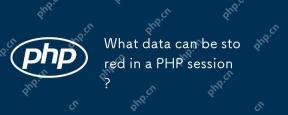
PHPsessionscanstorestrings,numbers,arrays,andobjects.1.Strings:textdatalikeusernames.2.Numbers:integersorfloatsforcounters.3.Arrays:listslikeshoppingcarts.4.Objects:complexstructuresthatareserialized.
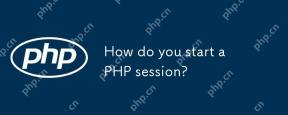
TostartaPHPsession,usesession_start()atthescript'sbeginning.1)Placeitbeforeanyoutputtosetthesessioncookie.2)Usesessionsforuserdatalikeloginstatusorshoppingcarts.3)RegeneratesessionIDstopreventfixationattacks.4)Considerusingadatabaseforsessionstoragei
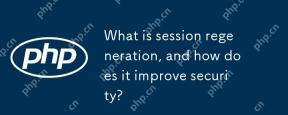
Session regeneration refers to generating a new session ID and invalidating the old ID when the user performs sensitive operations in case of session fixed attacks. The implementation steps include: 1. Detect sensitive operations, 2. Generate new session ID, 3. Destroy old session ID, 4. Update user-side session information.
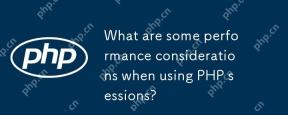
PHP sessions have a significant impact on application performance. Optimization methods include: 1. Use a database to store session data to improve response speed; 2. Reduce the use of session data and only store necessary information; 3. Use a non-blocking session processor to improve concurrency capabilities; 4. Adjust the session expiration time to balance user experience and server burden; 5. Use persistent sessions to reduce the number of data read and write times.
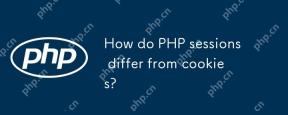
PHPsessionsareserver-side,whilecookiesareclient-side.1)Sessionsstoredataontheserver,aremoresecure,andhandlelargerdata.2)Cookiesstoredataontheclient,arelesssecure,andlimitedinsize.Usesessionsforsensitivedataandcookiesfornon-sensitive,client-sidedata.
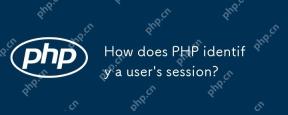
PHPidentifiesauser'ssessionusingsessioncookiesandsessionIDs.1)Whensession_start()iscalled,PHPgeneratesauniquesessionIDstoredinacookienamedPHPSESSIDontheuser'sbrowser.2)ThisIDallowsPHPtoretrievesessiondatafromtheserver.
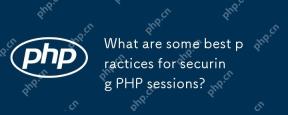
The security of PHP sessions can be achieved through the following measures: 1. Use session_regenerate_id() to regenerate the session ID when the user logs in or is an important operation. 2. Encrypt the transmission session ID through the HTTPS protocol. 3. Use session_save_path() to specify the secure directory to store session data and set permissions correctly.
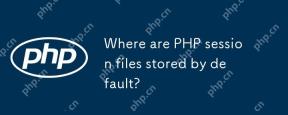
PHPsessionfilesarestoredinthedirectoryspecifiedbysession.save_path,typically/tmponUnix-likesystemsorC:\Windows\TemponWindows.Tocustomizethis:1)Usesession_save_path()tosetacustomdirectory,ensuringit'swritable;2)Verifythecustomdirectoryexistsandiswrita


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
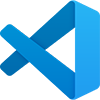
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

SublimeText3 English version
Recommended: Win version, supports code prompts!

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

Atom editor mac version download
The most popular open source editor

SublimeText3 Chinese version
Chinese version, very easy to use
