Common problems and solutions for decorators in Python
Common problems and solutions for decorators in Python
- What is a decorator?
Decorator is a very powerful function in Python , can be used to modify the behavior of existing functions or classes without modifying their source code. A decorator is actually a function or class that accepts a function or class as a parameter and returns a new function or class. - How to write a simple decorator?
The following is an example of a simple decorator:
def decorator(func): def inner_function(): print("Before function") func() print("After function") return inner_function @decorator def hello(): print("Hello, World!") hello()
The output result is:
Before function Hello, World! After function
This decorator The function will print additional information before and after the original function is executed.
- What is the relationship between decorators and closures?
A decorator is essentially a closure function. Closure refers to referencing the variables of the external function in an internal function, so that the internal function can access the variables of the external function. In the decorator, the inner function accepts the parameters of the outer function and calls the outer function in the inner function. - How to pass parameters in the decorator?
Sometimes, we need to pass additional parameters in the decorator. This can be achieved by defining a decorator function with parameters.
def decorator_with_args(arg1, arg2): def decorator(func): def inner_function(*args, **kwargs): print(f"Decorator arg1={arg1}, arg2={arg2}") func(*args, **kwargs) return inner_function return decorator @decorator_with_args("Hello", 42) def hello(name): print(f"Hello, {name}!") hello("World")
The output result is:
Decorator arg1=Hello, arg2=42 Hello, World!
In this example, the decorator function decorator_with_args
receives two parameters and then returns a new decorator function. The new decorator function accepts the parameters of the target function and calls the target function while printing the parameters.
- How does the decorator retain the meta-information of the original function?
In the internal function of the decorator, the@functools.wraps
decorator is often used to retain the meta-information of the original function. Meta information. This can avoid debugging difficulties caused by the decorator modifying information such as function names and documentation strings.
import functools def decorator(func): @functools.wraps(func) def inner_function(*args, **kwargs): print("Before function") func(*args, **kwargs) print("After function") return inner_function @decorator def hello(): """This is the Hello function.""" print("Hello, World!") print(hello.__name__) print(hello.__doc__)
The output result is:
hello This is the Hello function.
In this example, @functools.wraps(func)
retains the __name__
of the original function and __doc__
attributes.
- How are decorators used in classes?
Decorators can be applied not only to functions, but also to classes. In a class decorator, the decorator function receives a class as a parameter and returns a new class.
def decorator(cls): class NewClass(cls): def decorated_method(self): print("Decorated method") super().decorated_method() return NewClass @decorator class MyClass: def decorated_method(self): print("Original method") obj = MyClass() obj.decorated_method()
The output result is:
Decorated method Original method
In this example, the decorator function creates a new class NewClass
, which inherits from the original classMyClass
and adds extra functionality to the original method.
Summary:
Decorator is a very powerful function in Python, which can be used to modify the behavior of existing functions or classes. When using decorators, you may encounter some problems, such as how to pass additional parameters, how to retain the meta-information of the original function, etc. The examples above provide solutions to some common problems, detailed with code examples. By using decorators flexibly, we can add more scalability and reusability to our code.
The above is the detailed content of Common problems and solutions for decorators in Python. For more information, please follow other related articles on the PHP Chinese website!
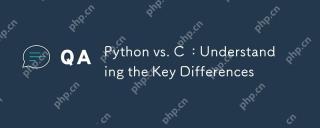
Python and C each have their own advantages, and the choice should be based on project requirements. 1) Python is suitable for rapid development and data processing due to its concise syntax and dynamic typing. 2)C is suitable for high performance and system programming due to its static typing and manual memory management.
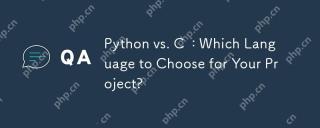
Choosing Python or C depends on project requirements: 1) If you need rapid development, data processing and prototype design, choose Python; 2) If you need high performance, low latency and close hardware control, choose C.
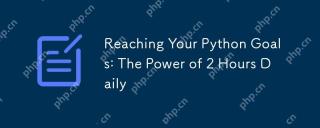
By investing 2 hours of Python learning every day, you can effectively improve your programming skills. 1. Learn new knowledge: read documents or watch tutorials. 2. Practice: Write code and complete exercises. 3. Review: Consolidate the content you have learned. 4. Project practice: Apply what you have learned in actual projects. Such a structured learning plan can help you systematically master Python and achieve career goals.
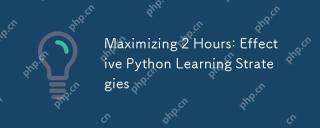
Methods to learn Python efficiently within two hours include: 1. Review the basic knowledge and ensure that you are familiar with Python installation and basic syntax; 2. Understand the core concepts of Python, such as variables, lists, functions, etc.; 3. Master basic and advanced usage by using examples; 4. Learn common errors and debugging techniques; 5. Apply performance optimization and best practices, such as using list comprehensions and following the PEP8 style guide.

Python is suitable for beginners and data science, and C is suitable for system programming and game development. 1. Python is simple and easy to use, suitable for data science and web development. 2.C provides high performance and control, suitable for game development and system programming. The choice should be based on project needs and personal interests.
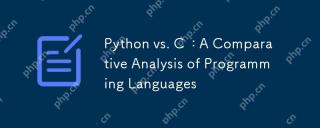
Python is more suitable for data science and rapid development, while C is more suitable for high performance and system programming. 1. Python syntax is concise and easy to learn, suitable for data processing and scientific computing. 2.C has complex syntax but excellent performance and is often used in game development and system programming.
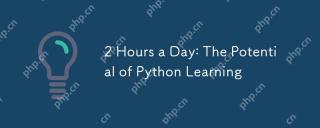
It is feasible to invest two hours a day to learn Python. 1. Learn new knowledge: Learn new concepts in one hour, such as lists and dictionaries. 2. Practice and exercises: Use one hour to perform programming exercises, such as writing small programs. Through reasonable planning and perseverance, you can master the core concepts of Python in a short time.
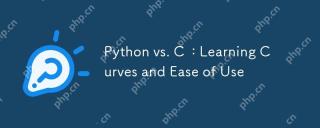
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor
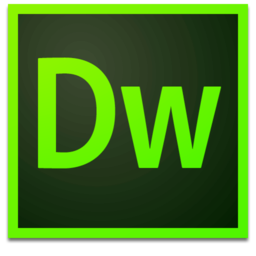
Dreamweaver Mac version
Visual web development tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
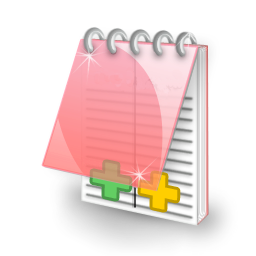
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function