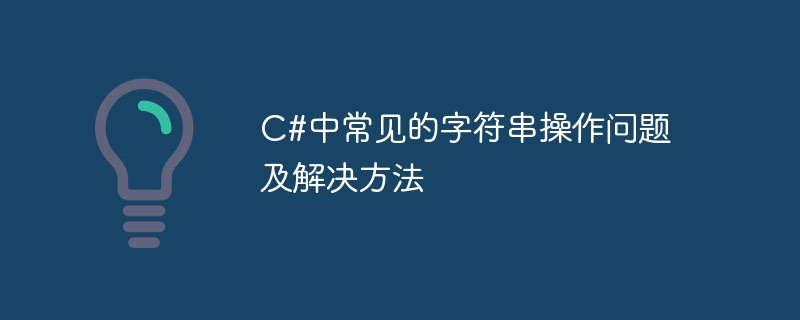
Common string operation problems and solutions in C
- #String splicing problems
In C#, we often need to combine multiple characters Strings are concatenated together, but if you use the simple plus " " operator, there will be performance issues. This is because a new string object is created every time strings are spliced, resulting in frequent allocation and recycling of memory. The solution is to use the StringBuilder class, which can concatenate strings without creating a new string object.
The sample code is as follows:
string str1 = "Hello";
string str2 = "World";
StringBuilder sb = new StringBuilder();
sb.Append(str1);
sb.Append(str2);
string result = sb.ToString();
- String comparison problem
In C#, when comparing whether two strings are equal, the "==" operation is generally used symbol. However, this method only applies to ordinary string comparisons. For different string comparison rules (such as case insensitivity, special character comparison, etc.), you need to use the String.Compare or String.Equals method and specify the comparison rules. .
The sample code is as follows:
string str1 = "hello";
string str2 = "Hello";
if (String.Compare(str1, str2, StringComparison.OrdinalIgnoreCase) == 0)
{
Console.WriteLine("字符串相等");
}
- String interception problem
In C#, we often need to intercept part of the string, but if we use the SubString method, then A new string object will be created, which will also cause performance issues. The solution is to use the String.Substring method and save the intercepted result in a new string variable.
The sample code is as follows:
string str = "Hello, World!";
string subStr = str.Substring(0, 5);
Console.WriteLine(subStr); // 输出 "Hello"
- String splitting problem
In C#, sometimes it is necessary to split a string into multiple substrings. The commonly used method is Use the String.Split method. However, if the split string is very long or contains many substrings, it can cause performance issues. The solution is to use the StringTokenizer class, which can split the string one by one according to the specified splitting characters to avoid loading the entire string into memory at once.
The sample code is as follows:
string str = "apple, banana, orange";
string[] fruits = str.Split(',');
foreach (string fruit in fruits)
{
Console.WriteLine(fruit.Trim()); // 输出 "apple", "banana", "orange"
}
- String formatting problem
In C#, we often need to format some data into strings. The commonly used method is Use the String.Format method. However, a mismatch in the number of placeholders and parameters in the format string will cause a runtime exception. The solution is to use the String.Format method to increase the number of placeholders in the formatted string and remove the excess placeholders.
The sample code is as follows:
string name = "Tom";
int age = 20;
string message = String.Format("My name is {0} and I am {1} years old", name, age);
Console.WriteLine(message); // 输出 "My name is Tom and I am 20 years old"
Summary:
When performing string operations in C#, we should pay attention to performance issues and standard usage methods to avoid unnecessary errors. . By using the StringBuilder class for string concatenation, the String.Compare or String.Equals method for string comparison, the String.Substring method for string interception, the StringTokenizer class for string splitting, and the correct use of the String.Format method for character processing. String formatting can improve code readability and performance.
The above is the detailed content of Common string operation problems and solutions in C#. For more information, please follow other related articles on the PHP Chinese website!