


Common database connection and transaction processing problems and solutions in C
#Abstract:
With the rapid development of the Internet and information technology, the use of databases is becoming more and more common. coming more and more widely. As a developer, database connections and transaction processing are essential parts when writing applications. However, there are some common issues that can arise for various reasons. This article will introduce in detail common database connection and transaction processing problems in C#, and provide solutions and corresponding code examples.
1. Database connection problem
- Connection pool exhaustion
When the database connection is frequently opened and closed in the program, it may cause the connections in the connection pool to be exhausted. This causes the program to be unable to connect to the database, thus throwing an exception.
Solution:
Use using statement block to ensure that the connection is closed in time after using it. The sample code is as follows:
using (SqlConnection connection = new SqlConnection(connectionString)) { // 连接数据库 connection.Open(); // 执行数据库操作 // ... } // connection会自动关闭
- Connection timeout
When the database connection exceeds the preset time limit, a connection timeout exception may occur.
Solution:
You can modify the connection timeout by setting the Connection Timeout property in the connection string. The sample code is as follows:
string connectionString = "Data Source=.;Initial Catalog=MyDatabase;Integrated Security=True;Connection Timeout=30;";
- Database connection exception
When connecting to the database, various exceptions may occur, such as being unable to connect to the database, incorrect username or password, etc.
Solution:
You can capture exceptions through try-catch statement blocks and handle different exception types. The sample code is as follows:
try { using (SqlConnection connection = new SqlConnection(connectionString)) { // 连接数据库 connection.Open(); // 执行数据库操作 // ... } // connection会自动关闭 } catch (SqlException ex) { // 处理数据库连接异常 // ... } catch (Exception ex) { // 处理其他异常 // ... }
2. Transaction processing issues
- Transaction rollback
When performing database update operations, abnormal situations may occur and need to be rolled back previous operations.
Solution:
Use transaction processing. Transactions provide a mechanism to ensure the consistency of database operations. The sample code is as follows:
using (SqlConnection connection = new SqlConnection(connectionString)) { connection.Open(); // 开始事务 SqlTransaction transaction = connection.BeginTransaction(); try { // 执行数据库操作 // ... // 提交事务 transaction.Commit(); } catch (Exception ex) { // 发生异常,回滚事务 transaction.Rollback(); // 处理异常 // ... } }
- Concurrency conflicts
When multiple users modify the database at the same time, concurrency conflicts may occur.
Solution:
You can use optimistic locking or pessimistic locking to handle concurrency conflicts. Optimistic locking uses version numbers or timestamps to determine whether data has been modified, while pessimistic locking uses the database lock mechanism to ensure the integrity of transactions. The specific implementation depends on the specific database and requirements.
Conclusion:
Database connection and transaction processing are very important parts of C# applications. In actual development, you may encounter various problems, such as connection pool exhaustion, connection timeout, database connection exception, etc. This article explains these common problems in detail and provides corresponding solutions and code examples. I hope this article can help readers better understand and deal with database-related issues.
The above is the detailed content of Common database connection and transaction processing problems and solutions in C#. For more information, please follow other related articles on the PHP Chinese website!
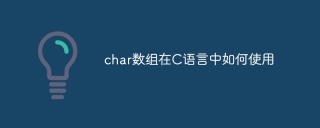
The char array stores character sequences in C language and is declared as char array_name[size]. The access element is passed through the subscript operator, and the element ends with the null terminator '\0', which represents the end point of the string. The C language provides a variety of string manipulation functions, such as strlen(), strcpy(), strcat() and strcmp().
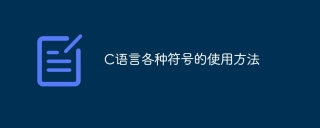
The usage methods of symbols in C language cover arithmetic, assignment, conditions, logic, bit operators, etc. Arithmetic operators are used for basic mathematical operations, assignment operators are used for assignment and addition, subtraction, multiplication and division assignment, condition operators are used for different operations according to conditions, logical operators are used for logical operations, bit operators are used for bit-level operations, and special constants are used to represent null pointers, end-of-file markers, and non-numeric values.
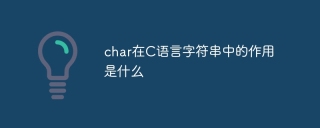
In C, the char type is used in strings: 1. Store a single character; 2. Use an array to represent a string and end with a null terminator; 3. Operate through a string operation function; 4. Read or output a string from the keyboard.
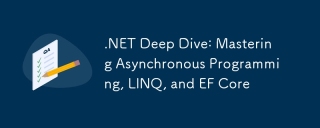
The core concepts of .NET asynchronous programming, LINQ and EFCore are: 1. Asynchronous programming improves application responsiveness through async and await; 2. LINQ simplifies data query through unified syntax; 3. EFCore simplifies database operations through ORM.
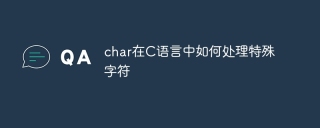
In C language, special characters are processed through escape sequences, such as: \n represents line breaks. \t means tab character. Use escape sequences or character constants to represent special characters, such as char c = '\n'. Note that the backslash needs to be escaped twice. Different platforms and compilers may have different escape sequences, please consult the documentation.
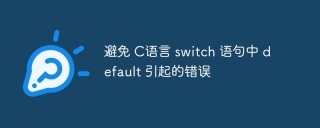
A strategy to avoid errors caused by default in C switch statements: use enums instead of constants, limiting the value of the case statement to a valid member of the enum. Use fallthrough in the last case statement to let the program continue to execute the following code. For switch statements without fallthrough, always add a default statement for error handling or provide default behavior.
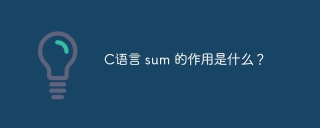
There is no built-in sum function in C language, so it needs to be written by yourself. Sum can be achieved by traversing the array and accumulating elements: Loop version: Sum is calculated using for loop and array length. Pointer version: Use pointers to point to array elements, and efficient summing is achieved through self-increment pointers. Dynamically allocate array version: Dynamically allocate arrays and manage memory yourself, ensuring that allocated memory is freed to prevent memory leaks.
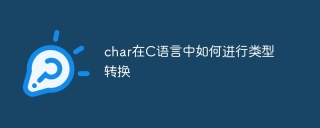
In C language, char type conversion can be directly converted to another type by: casting: using casting characters. Automatic type conversion: When one type of data can accommodate another type of value, the compiler automatically converts it.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
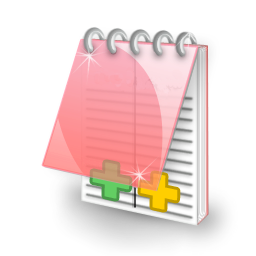
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
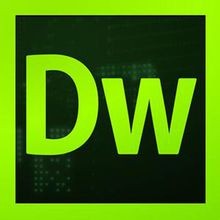
Dreamweaver CS6
Visual web development tools
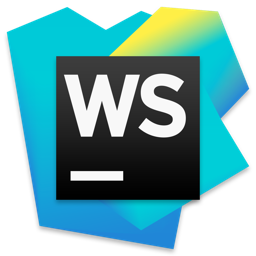
WebStorm Mac version
Useful JavaScript development tools
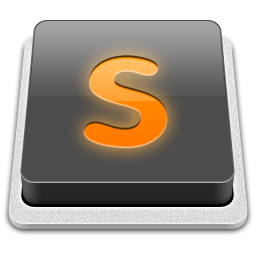
SublimeText3 Mac version
God-level code editing software (SublimeText3)

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
