How to handle file upload and download issues in Vue technology development
Introduction:
In modern Web development, file upload and download are common requirements , especially in Vue technology development. This article will introduce how to use Vue to handle file upload and download problems, and provide specific code examples.
1. File upload
In Vue, processing file uploads usually requires the use of HTML input elements and FormData objects. The following is an example that demonstrates how to use Vue to handle file uploads:
<template> <div> <input type="file" @change="handleFileUpload" /> <button @click="uploadFile">上传</button> </div> </template> <script> export default { data() { return { file: null, }; }, methods: { handleFileUpload(e) { this.file = e.target.files[0]; }, uploadFile() { let formData = new FormData(); formData.append('file', this.file); // 发送文件上传请求 // axios.post('/upload', formData).then(response => { // console.log(response.data); // }); // 省略上传逻辑,此处只是演示 console.log('文件上传成功'); }, }, }; </script>
In the above code, we listen for file selection through the change event of the input element and assign the selected file to the file attribute in the Vue instance. Next, we use the FormData object to create a form and add the file to it. Finally, you can use tools like axios to send file upload requests.
2. File Download
Processing file downloads usually requires the server to provide an interface to trigger file downloads through front-end requests. The following is an example that demonstrates how to use Vue to handle file downloads:
<template> <div> <button @click="downloadFile">下载</button> </div> </template> <script> export default { methods: { downloadFile() { // 发送文件下载请求 // axios.get('/download').then(response => { // let link = document.createElement('a'); // link.href = window.URL.createObjectURL(new Blob([response.data])); // link.setAttribute('download', 'filename'); // document.body.appendChild(link); // link.click(); // link.remove(); // }); // 省略下载逻辑,此处只是演示 console.log('文件下载成功'); }, }, }; </script>
In the above code, we trigger file downloads through the click event of the button. You can use tools such as axios to send a file download request, and assign the file data returned by the server to the href attribute of an a tag in the form of a URL through the Blob object. Finally, trigger the download by simulating a click.
Conclusion:
Through the above code examples, we have learned how to use Vue to handle file upload and download problems. Of course, real file upload and download operations usually need to be completed with the server side. However, the code examples provided here can help us understand the basic process of file upload and download, and can be modified and expanded accordingly in actual projects. I hope this article can help you deal with file upload and download problems in Vue technology development.
The above is the detailed content of How to handle file upload and download issues in Vue technology development. For more information, please follow other related articles on the PHP Chinese website!
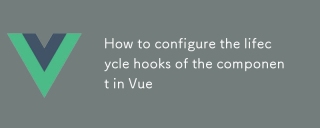
This article clarifies the role of export default in Vue.js components, emphasizing that it's solely for exporting, not configuring lifecycle hooks. Lifecycle hooks are defined as methods within the component's options object, their functionality un
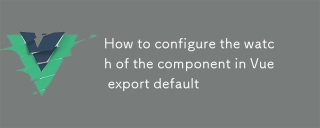
This article clarifies Vue.js component watch functionality when using export default. It emphasizes efficient watch usage through property-specific watching, judicious deep and immediate option use, and optimized handler functions. Best practices
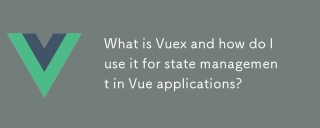
This article explains Vuex, a state management library for Vue.js. It details core concepts (state, getters, mutations, actions) and demonstrates usage, emphasizing its benefits for larger projects over simpler alternatives. Debugging and structuri

Article discusses creating and using custom Vue.js plugins, including development, integration, and maintenance best practices.
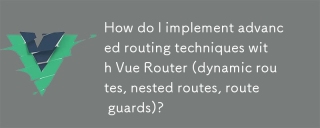
This article explores advanced Vue Router techniques. It covers dynamic routing (using parameters), nested routes for hierarchical navigation, and route guards for controlling access and data fetching. Best practices for managing complex route conf

Vue.js enhances web development with its Component-Based Architecture, Virtual DOM for performance, and Reactive Data Binding for real-time UI updates.

The article explains how to configure Vue CLI for different build targets, switch environments, optimize production builds, and ensure source maps in development for debugging.
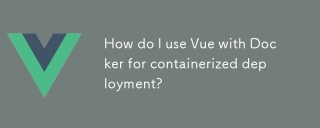
The article discusses using Vue with Docker for deployment, focusing on setup, optimization, management, and performance monitoring of Vue applications in containers.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
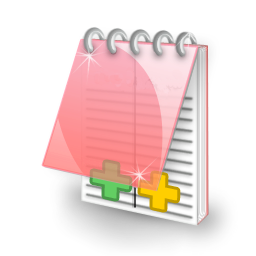
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
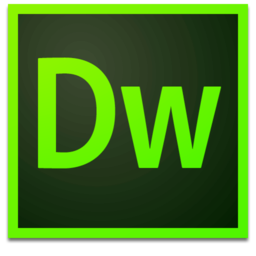
Dreamweaver Mac version
Visual web development tools

Notepad++7.3.1
Easy-to-use and free code editor
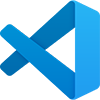
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
