


Common problems encountered in C# technology development and their solutions
Common problems and solutions encountered in C# technology development
Introduction: C# is an object-oriented high-level programming language that is widely used in Windows applications development. However, during the development process of C# technology, you may encounter some common problems. This article will introduce some common problems, provide corresponding solutions, and attach specific code examples to help readers better understand and solve these problems.
1. NullReferenceException (null reference exception)
In the C# development process, NullReferenceException is a common error. This exception occurs when we try to refer to a null reference or uninitialized object. Solutions to this problem could be by adding null checks, or by ensuring that the object is properly initialized before use.
The following code example demonstrates how to use null checking to avoid NullReferenceException:
string str = null; if (str != null) { Console.WriteLine(str.Length); }
2. Array out-of-bounds exception
The array out-of-bounds exception is caused by trying to access a value that does not exist in the array caused by indexing. To avoid this problem, we should make sure to check the length of the array before accessing its elements to ensure we are operating within a valid index range.
The following code example demonstrates how to check the length of the array before accessing the array elements:
int[] numbers = new int[3]; if (index >= 0 && index < numbers.Length) { Console.WriteLine(numbers[index]); }
3. Deadlock problem
In a multi-threaded environment, deadlock is a common question. Deadlock occurs when multiple threads wait for each other to release the lock. In order to solve the deadlock problem, we can use some technical means, such as avoiding nested locks, acquiring locks in a fixed order, and using a timeout mechanism.
The following code example demonstrates how to acquire locks in a fixed order to avoid deadlock problems:
lock (lockA) { lock (lockB) { // 执行代码 } }
4. Memory leak problem
Memory leak refers to the problem that the program does not Correctly release memory that is no longer in use, causing excessive memory usage. To avoid memory leaks, we can use the garbage collector in C#, which will automatically reclaim memory that is no longer used. In addition, we can also solve the memory leak problem by releasing resources in time, using using statements to automatically release resources, and avoiding circular references.
The following code example demonstrates how to use the using statement to automatically release resources:
using (FileStream fs = new FileStream("example.txt", FileMode.Open)) { // 执行代码 }
Conclusion:
In the development of C# technology, we may encounter some common problems , such as null reference exception, array out-of-bounds exception, deadlock problem and memory leak problem. Through the introduction of this article, we can learn some methods to solve these problems, and give specific code examples to help readers better deal with these problems. I hope this article will be helpful to readers in their practice of C# technology development.
The above is the detailed content of Common problems encountered in C# technology development and their solutions. For more information, please follow other related articles on the PHP Chinese website!
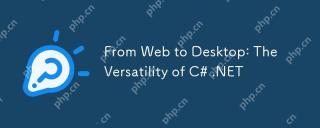
C#.NETisversatileforbothwebanddesktopdevelopment.1)Forweb,useASP.NETfordynamicapplications.2)Fordesktop,employWindowsFormsorWPFforrichinterfaces.3)UseXamarinforcross-platformdevelopment,enablingcodesharingacrossWindows,macOS,Linux,andmobiledevices.
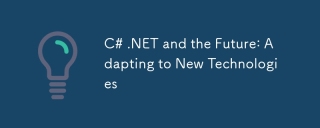
C# and .NET adapt to the needs of emerging technologies through continuous updates and optimizations. 1) C# 9.0 and .NET5 introduce record type and performance optimization. 2) .NETCore enhances cloud native and containerized support. 3) ASP.NETCore integrates with modern web technologies. 4) ML.NET supports machine learning and artificial intelligence. 5) Asynchronous programming and best practices improve performance.
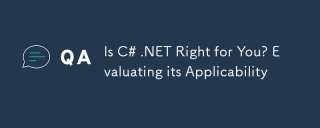
C#.NETissuitableforenterprise-levelapplicationswithintheMicrosoftecosystemduetoitsstrongtyping,richlibraries,androbustperformance.However,itmaynotbeidealforcross-platformdevelopmentorwhenrawspeediscritical,wherelanguageslikeRustorGomightbepreferable.
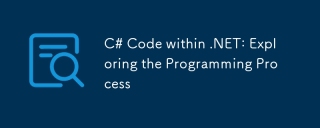
The programming process of C# in .NET includes the following steps: 1) writing C# code, 2) compiling into an intermediate language (IL), and 3) executing by the .NET runtime (CLR). The advantages of C# in .NET are its modern syntax, powerful type system and tight integration with the .NET framework, suitable for various development scenarios from desktop applications to web services.
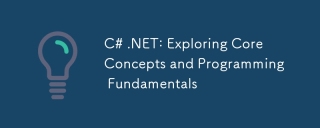
C# is a modern, object-oriented programming language developed by Microsoft and as part of the .NET framework. 1.C# supports object-oriented programming (OOP), including encapsulation, inheritance and polymorphism. 2. Asynchronous programming in C# is implemented through async and await keywords to improve application responsiveness. 3. Use LINQ to process data collections concisely. 4. Common errors include null reference exceptions and index out-of-range exceptions. Debugging skills include using a debugger and exception handling. 5. Performance optimization includes using StringBuilder and avoiding unnecessary packing and unboxing.
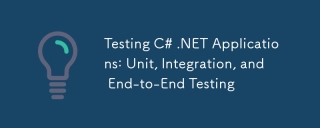
Testing strategies for C#.NET applications include unit testing, integration testing, and end-to-end testing. 1. Unit testing ensures that the minimum unit of the code works independently, using the MSTest, NUnit or xUnit framework. 2. Integrated tests verify the functions of multiple units combined, commonly used simulated data and external services. 3. End-to-end testing simulates the user's complete operation process, and Selenium is usually used for automated testing.
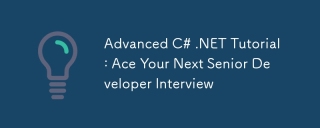
Interview with C# senior developer requires mastering core knowledge such as asynchronous programming, LINQ, and internal working principles of .NET frameworks. 1. Asynchronous programming simplifies operations through async and await to improve application responsiveness. 2.LINQ operates data in SQL style and pay attention to performance. 3. The CLR of the NET framework manages memory, and garbage collection needs to be used with caution.
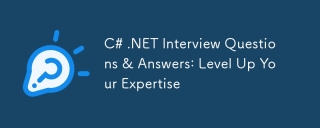
C#.NET interview questions and answers include basic knowledge, core concepts, and advanced usage. 1) Basic knowledge: C# is an object-oriented language developed by Microsoft and is mainly used in the .NET framework. 2) Core concepts: Delegation and events allow dynamic binding methods, and LINQ provides powerful query functions. 3) Advanced usage: Asynchronous programming improves responsiveness, and expression trees are used for dynamic code construction.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
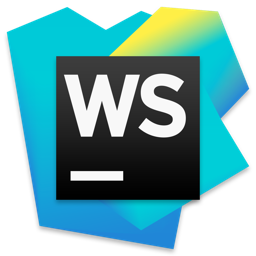
WebStorm Mac version
Useful JavaScript development tools
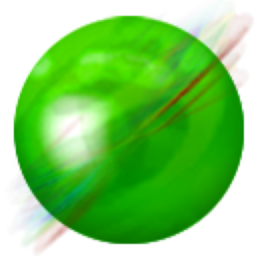
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment